Question
Complete the code for the four problems that follow. Leave everything as a public boolean. Possibly relevant code is included here: Rules: * - DO
Complete the code for the four problems that follow. Leave everything as a public boolean. Possibly relevant code is included here:
Rules:
* - DO NOT change the Node class.
* - DO NOT change the first line of any function: name, parameters, types.
* - you may add new functions, but don't delete anything
* - functions must be recursive
* - no loops
* - each function must have exactly one recursive helper function, which you add
* - each function must be independent --- do not call any function other than the helper
* - no fields (variables declared outside of a function)
public int size () {
return size (root, 0);
}
private static int size (Node x, int sz) {
if (x != null) {
sz = sz + 1;
sz = size (x.left, sz);
sz = size (x.right, sz);
}
return sz;
}
// the height of the tree
public int height() {
return height(this.root);
}
private int height(Node current) {
if (current == null) {
return -1;
}
else {
return 1+ Math.max(height(current.left), height(current.right));
}
}
THINGS NEEDING SOLVING STARTS HERE:
#1
// tree is perfect if for every node, size of left == size of right
// hint: in the helper, return -1 if the tree is not perfect, otherwise return the size
public boolean isPerfectlyBalancedS() {
// TODO: complete code
return false;
}
#2
// tree is perfect if for every node, height of left == height of right
// hint: in the helper, return -2 if the tree is not perfect, otherwise return the height
public boolean isPerfectlyBalancedH() {
// TODO: complete code
return false;
}
#3
// tree is odd-perfect if for every node, #odd descendant on left == # odd descendants on right
// A node is odd if it has an odd key
// hint: in the helper, return -1 if the tree is not odd-perfect, otherwise return the odd size
public boolean isOddBalanced() {
// TODO: complete code
return false;
}
#4
// tree is semi-perfect if every node is semi-perfect
// A node with 0 children is semi-perfect.
// A node with 1 child is NOT semi-perfect.
// A node with 2 children is semi-perfect if (size-of-larger-child <= size-of-smaller-child * 3)
// hint: in the helper, return -1 if the tree is not semi-perfect, otherwise return the size
public boolean isSemiBalanced() {
// TODO: complete code
return false;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
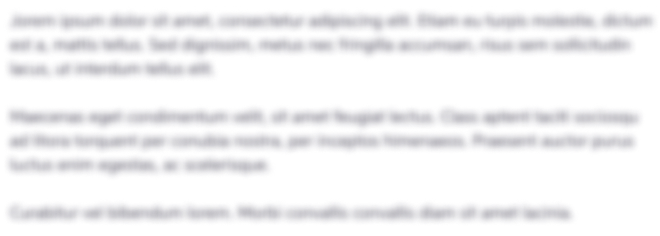
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started