Question
Complete the definition of the class OddList that is a subclass of list, of which a partial definition can be found in the template file
Complete the definition of the class OddList that is a subclass of list, of which a partial definition can be found in the template file posted to D2L. The class behaves just like an ordinary list except that it only prints the items in the odd-numbered (e.g. 1, 3, 5, ...) indices. To do this you will need to write the OddListIterator class which has two methods:
__init__ that initializes the list to be printed and the first index to be printed appropriately
__next__ that determines and returns the next item in the list, updating the variables of the class as needed
The following shows how the OddList class could be used:
Implement an exception class InvalidAge() for the Animal class implemented in the second lab and found in the template file. In addition to writing the InvalidAge class you must modify the setAge() method of the Animal class to check if the age provided as a parameter is negative. If it is, it raises an InvalidAge exception. It should raise the exception before any object variables are modified. If the age is positive or zero, it should set the age of the animal to the specified parameter. The constructor should be modified in a similar way so that Animal objects with negative ages cannot be created. The following demonstrates how the classes could be used when completed (with appropriate edits to the output to not give away the answer to the problem:
>>> djengo = Animal('cat', 'meow', 15) >>> djengo.speak() 'I am a 15 year-old cat and I meow' >>> djengo.setAge(-16) Traceback (most recent call last): File "
Modify the processAnimals() function written for the second lab so that exceptions raised for negative ages in the file are handled. Any animal in the file with a negative age should be ignored and the rest of the file processed as before. You must use exception handling to deal with invalid ages. Do not use branching to anticipate the problem. Instead use try-except blocks to handle exceptions that may be raised by the methods of the Animal class. The information below shows how you would call the completed function on two example files. The first file is the same as the one provided for the second lab. The second file is one that contains some negative ages. Look carefully at the file to see which lines in the file are being added to the list of animals and which lines are raising exceptions and being skipped as a result.
animals.txt
cat,meow,8 tiger,roar,-5 dog,bark,22 python,hiss, -1 bat,use sonar,2
template file
#1 #Subclass of list tat iterates over odd indices class OddList(list): def __init__(self): pass
def __iter__(self): pass
class ListIterator: def __init__(self): pass
def __next__(self): pass #2 #Modified Animal class with InvalidAge exception for negative ages class InvalidAge(Exception): pass
class Animal:
#modify __init__ def __init__(self, newSpecies="default", newLanguage="default", newAge=0): if newAge
def __repr__(self): return "Animal('{}','{}',{})".format(self.species, self.language, self.age)
def __eq__(self, otherAnimal): return self.species == otherAnimal.species and self.language == otherAnimal.language and self.age == otherAnimal.age
def setSpecies(self, newSpecies): self.species = newSpecies
def setLanguage(self, newLanguage): self.language = newLanguage
#modify setAge() def setAge(self, newAge): self.age = newAge
def speak(self): print("I am a {} year-old {} and I {}.".format(self.age, self.species, self.language))
#3 #Modified processAnimals function with InvalidAge exception def processAnimals(file): animalList = []
inFile = open(file) lineList = inFile.readlines() inFile.close()
#modify the code in loop to handle Animals being made with negative ages (refer to lab for output examples) for line in lineList: data = line.strip(' ').split(',') newAnimal = Animal(data[0], data[1], eval(data[2])) animalList.append(newAnimal) newAnimal.speak() return animalList
**** PLEASE GIVE ME A COMPLETE SOLUTION TO THIS TEMPLATE FILE****
Python 3.4.1 Shell File Edit Shell Debug Options Windows Help lst 1 2, 3, 4] lst 1 Odd List lst for num in lst 1 print (num) for num in lst print (num) lst2 Odd List cat i dog hermit crab for a in lst2 print (a, end dog lat Odd List C only for word in lst3 print (word) lstA Odd List for n in lstA print (n) Ln: 31 Col: 0Step by Step Solution
There are 3 Steps involved in it
Step: 1
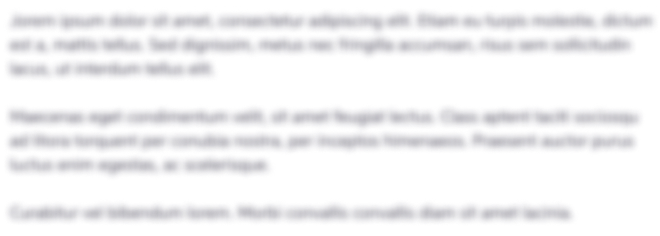
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started