Question
Complete the following case study: Case study: Three implementations of contains - comparing approaches to adding functionality Set A contains Set B if every element
Complete the following case study:
Case study: Three implementations of contains - comparing approaches to adding functionality
Set A contains Set B if every element in Set B is also in Set A. We will compare three ways to determine whether Set A contains Set B.
Approach 1: Write code in the main method in a test class
Add code to the main of ArraySetTester to create SetA and SetB, fill them with data and write a contains operation that tests if SetA contains SetB.
Question: What is wrong with this approach? Answer: While the code does the operation for the specific collections SetA and SetB, it only applies to these two collections. The code can't be used for other Sets without repeating it.
Approach 2: Write a static method in a test class
Comment out the code from approach 1.
Add a static method to ArraySetTester with prototype:
public static boolean contains(SetADT
Question: Why does contains need to be static? Answer: We aren't instantiating an object to call the contains. Therefore contains has to be associated with the ArraySetTester class itself, rather than a particular instance.
Question: Why were Set1 and Set2 declared as SetADT rather than as ArraySet? Answer: This way the contains method can be called for any implementation of SetADT.
Approach 3: Add a prototype to the interface
Add the following prototype to the SetADT interface and add an implementation of contains to ArraySet.
public boolean contains(SetADT
Question: Why is Approach 3 generally better than Approach 2? Answer: We have associated the code for contains with the classes that need it most, i.e., if you call contains, you will need SetADT and an implementation of it. This is the object-oriented approach. We can ask the object itself whether it contains another.
ArraySet.java
package arraysetpackage;
import java.util.Iterator;
public class ArraySetTester {
public static void main(String[] args) { SetADT
for (int i = 0; i < 12; i++) mySet.add(new String("apple"+i));
System.out.println(mySet); System.out.println("mysize = "+mySet.size()+ " [expect 12]"); mySet.add(new String ("apple0")); System.out.println("mysize = "+mySet.size()+ " [expect 12]"); System.out.println("contains 11? = "+mySet.contains(new String("11"))); System.out.println("contains apple11? = "+mySet.contains(new String("apple11"))); try { String removedItem = mySet.remove("apple7"); System.out.println(mySet); System.out.println(removedItem+ " was removed"); } catch (Exception e) { System.out.println("item not found, can't remove"); } try { String removedItem = mySet.remove("apple17"); System.out.println(mySet); System.out.println(removedItem+ " was removed"); } catch (Exception e) { System.out.println("item not found, can't remove"); } Iterator
ArraySetIterator.java
package arraysetpackage;
import java.util.Iterator; import java.util.NoSuchElementException;
public class ArraySetIterator
}
ArraySetTester.java
package arraysetpackage;
import java.util.Iterator;
public class ArraySetTester {
public static void main(String[] args) { SetADT
for (int i = 0; i < 12; i++) mySet.add(new String("apple"+i));
System.out.println(mySet); System.out.println("mysize = "+mySet.size()+ " [expect 12]"); mySet.add(new String ("apple0")); System.out.println("mysize = "+mySet.size()+ " [expect 12]"); System.out.println("contains 11? = "+mySet.contains(new String("11"))); System.out.println("contains apple11? = "+mySet.contains(new String("apple11"))); try { String removedItem = mySet.remove("apple7"); System.out.println(mySet); System.out.println(removedItem+ " was removed"); } catch (Exception e) { System.out.println("item not found, can't remove"); } try { String removedItem = mySet.remove("apple17"); System.out.println(mySet); System.out.println(removedItem+ " was removed"); } catch (Exception e) { System.out.println("item not found, can't remove"); } Iterator
SetADT
for (int i = 0; i < 12; i++) mySet2.add(new String("orange"+i)); System.out.println(mySet2); // add code here to test methods you finish in ArraySet // after you complete the existing methods, do the Case Study // Approach 1 will be here in the main // Approach 2 will be here in ArraySetTester, but you will // create a local static method that you will call from the main // Approach 3 will start with uncommenting the prototype in SetADT // and then creating the method in ArraySet. Finally you will write // code here to test the new method }
}
SetADT.java
package arraysetpackage;
import java.util.Iterator;
public interface SetADT
Step by Step Solution
There are 3 Steps involved in it
Step: 1
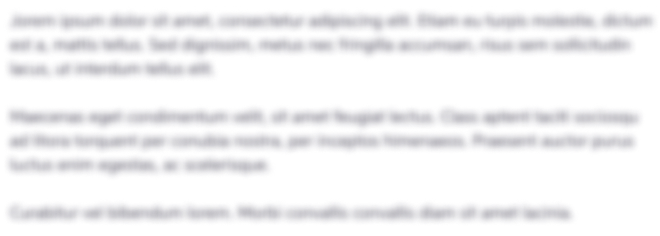
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started