Question
complete the following code in java in-class client : Add a method transferfee that transfers an amount of money. Ensures that the required amount does
complete the following code in java
in-class client :
Add a method transferfee that transfers an amount of money. Ensures that the required amount does not exceed the client balance. Also, the transfer fee should be deducted from the balance.
add class banksystem
In the class BankSystem with the main method, you will create an array of 3 objects of the class NormalClient and another array of 3 objects of the class GoldenClient. Write a method that prompts the user to enter the Name and the initial balance and fill the array. Write a print method that prints all the objects in the array the main method. Then ask the user for the wanted operation (show balance, deposit, or debit) and perform it.
package client; import java.util.*;
public class Client {
static int No; String ID; String Name; double balance; final double Fee=2.5; Client(String id, String name, double initialBalance){ this.ID=id; this.Name=name; if(initialBalance>0){ this.balance= initialBalance; } else{ this.balance=0.0; } } Client(Client c){ this.ID=c.getID(); this.Name=c.getName(); this.balance= c.getBalance(); } public String getID(){ return ID; } public void setID(String idx){ this.ID=idx; } public String getName(){ return Name; } public void setName(String n1){ this.Name= n1; } public double getBalance(){ return balance; } public void setBalance(double balance){ this.balance= balance; }
@Override public String toString() { return "Client{" + "ID=" + ID + ", Name=" + Name + ", balance=" + balance + '}'; } public void deposit(double deposit){ this.balance+=deposit; System.out.println(this.balance); } public void debit(double withdraw){ Scanner scan = new Scanner(System.in); boolean contniue= true; while(contniue){ if(withdraw<= this.balance){ this.balance -=withdraw; System.out.println(this.balance); break; } else{ System.out.println("The debit amount exceeded account balance"); System.out.println("enter other amount or 0 to exit"); double number= scan.nextDouble(); if (number==0){ break; } } } } }
package client; public class GoldenClient { static int GldNo; String ID; String Name; double balance;
GoldenClient(String id, String name, double balance){ this.ID=id; this.Name=name; if(balance>0){ this.balance= balance; } else{ this.balance=0.0; } } GoldenClient(GoldenClient gc){ this.ID=gc.getID(); this.Name=gc.getName(); this.balance= gc.getBalance(); } public String getID(){ return ID; } public void setID(String idx){ this.ID=idx; } public String getName(){ return Name; } public void setName(String n1){ this.Name= n1; } public double getBalance(){ return balance; } public void setBalance(double balance){ this.balance= balance; }
@Override public String toString() { return "GoldenClient{" + "ID=" + ID + ", Name=" + Name + ", balance=" + balance + '}'; } public void deposit(double deposit){ this.balance+=deposit; System.out.println(this.balance); } public void debit(double withdraw){ Scanner scan = new Scanner(System.in); boolean contniue= true; while(contniue){ if(withdraw<= this.balance){ this.balance -=withdraw; System.out.println(this.balance); break; } else{ System.out.println("The debit amount exceeded account balance"); System.out.println("enter other amount or 0 to exit"); double number= scan.nextDouble(); if (number==0){ break; } } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
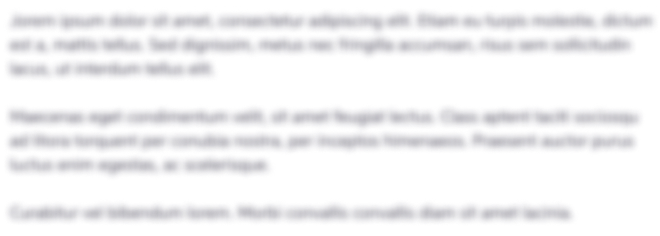
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started