Question
Complete the following codes. import java.util.Comparator; /** Implementation of a priority queue by means of a sorted node list. */ public class SortedListPriorityQueue implements PriorityQueue
Complete the following codes.
import java.util.Comparator; /** Implementation of a priority queue by means of a sorted node list. */
public class SortedListPriorityQueue
protected Comparator
}
/** Creates the priority queue with the default comparator. */ public SortedListPriorityQueue () { entries = new NodePositionList
public int size() {return entries.size();} public boolean isEmpty() {return entries.isEmpty();} /** Returns but does not remove an entry with minimum key. */ public Entry
/** Determines whether a given key is valid */ protected void checkKey(K key) throws InvalidKeyException {
if (key == null) throw new InvalidKeyException("Invalid key");
}
/** Auxiliary method used for insertion. */ protected void insertEntry(Entry
public static void main(String[] args) { SortedListPriorityQueue
for (int i = 0; i <= n; ++i) { SPQ.removeMin(); System.out.println ( SPQ.removeMin()); }
SortedListPriorityQueue
System.out.println(); System.out.println(" Unorted strings are: ");
for (String s: SArray) { Entry
SortedListPriorityQueue
System.out.println("Inserted entries are: "); for (int i = 0; i <= n; ++i) {
SPQC.insert (i, i); System.out.println(SPQC.insert (i, i)); } System.out.println(" Sorted entries by the integerComparator are: ");
for (int i = 0; i <= n; ++i) { SPQC.removeMin(); System.out.println ( SPQC.removeMin()); }
System.out.println();
SortedListPriorityQueue
System.out.println("Inserted entries are: "); for (int i = 0; i <= n; ++i) {
SPQCB.insert (i, i); System.out.println(SPQCB.insert (i, i)); } System.out.println(" Sorted entries by the BinaryTest are: ");
for (int i = 0; i <= n; ++i) { SPQCB.removeMin(); System.out.println ( SPQCB.removeMin()); }
System.out.println();
} }
Please who can help with this question in Java
Thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
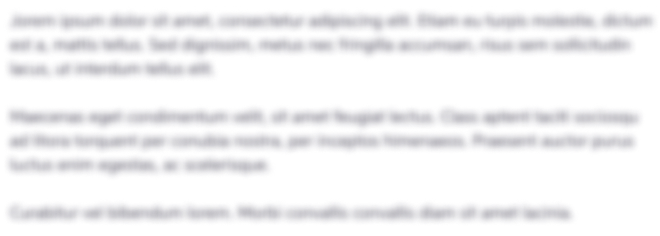
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started