Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete the implementation of the ArrayStack class presented inChapter 12. Specifically, complete the implementations of theisEmpty, size, and toString methods. See Base_A05Q1.java for astarting place
Complete the implementation of the ArrayStack class presented inChapter 12. Specifically, complete the implementations of theisEmpty, size, and toString methods. See Base_A05Q1.java for astarting place and a description of these methods.
/** * Write a description of the program here. * * @author Lewis et al., (your name) * @version (program version) */import java.util.Arrays;public class Base_A05Q1{ /** * Program entry point for stack testing. * @param args Argument list. */ public static void main(String[] args) { ArrayStack stack = new ArrayStack(); System.out.println("STACK TESTING"); stack.push(3); stack.push(7); stack.push(4); System.out.println(stack.peek()); stack.pop(); stack.push(9); stack.push(8); System.out.println(stack.peek()); System.out.println(stack.pop()); System.out.println(stack.peek()); System.out.println("The size of the stack is: " + stack.size()); System.out.println("The stack contains:" + stack.toString()); } /** * An array implementation of a stack in which the bottom of the * stack is fixed at index 0. * * @author Java Foundations * @version 4.0 */ public static class ArrayStack implements StackADT { private final static int DEFAULT_CAPACITY = 100; private int top; private T[] stack; /** * Creates an empty stack using the default capacity. */ public ArrayStack() { this(DEFAULT_CAPACITY); } /** * Creates an empty stack using the specified capacity. * @param initialCapacity the initial size of the array */ @SuppressWarnings("unchecked") //see p505. public ArrayStack(int initialCapacity) { top = 0; stack = (T[])(new Object[initialCapacity]); } /** * Adds the specified element to the top of this stack, expanding * the capacity of the array if necessary. * @param element generic element to be pushed onto stack */ public void push(T element) { if (size() == stack.length) expandCapacity(); stack[top] = element; top++; } /** * Creates a new array to store the contents of this stack with * twice the capacity of the old one. */ private void expandCapacity() { stack = Arrays.copyOf(stack, stack.length * 2); } /** * Removes the element at the top of this stack and returns a * reference to it. * @return element removed from top of stack * @throws EmptyCollectionException if stack is empty */ public T pop() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack"); top--; T result = stack[top]; stack[top] = null; return result; } /** * Returns a reference to the element at the top of this stack. * The element is not removed from the stack. * @return element on top of stack * @throws EmptyCollectionException if stack is empty */ public T peek() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack"); return stack[top-1]; } /** * Returns true if this stack is empty and false otherwise. * @return true if this stack is empty */ public boolean isEmpty() { //TODO: Implement me. return false; } /** * Returns the number of elements in this stack. * @return the number of elements in the stack */ public int size() { //TODO: Implement me. return 0; } /** * Returns a string representation of this stack. The string has the * form of each element printed on its own line, with the top most * element displayed first, and the bottom most element displayed last. * If the list is empty, returns the word "empty". * @return a string representation of the stack */ public String toString() { //TODO: Implement me. return ""; } }}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
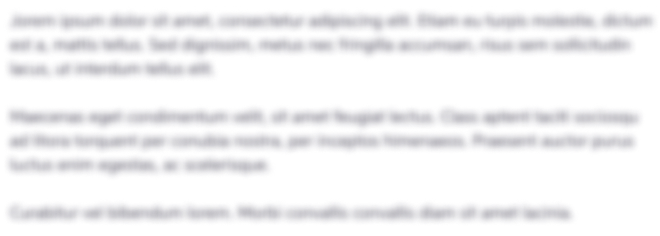
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started