Question
Complete the implementation of the methods in this SimpleBoundedList class. Must use the generics and do not use HashMap util. V remove(K key), V remove(int
Complete the implementation of the methods in this SimpleBoundedList class. Must use the generics and do not use HashMap util.
V remove(K key), V remove(int n), V lookup(K key), V remove(), size(), V get(int n), and Object[] toArray()
SimpleBoundedList.java
import java.util.*;
public class SimpleBoundedList
private class Entry {
protected K key; protected V value;
public Entry(K key, V value) { this.key = key; this.value = value; } } protected Object[] values;
private int start = 0; private int nextEmpty = 0;
/** * */ @SuppressWarnings("unchecked") public SimpleBoundedList(int bound) { values = new Object[bound]; }
@Override public boolean add(K key, V value) { boolean modify = false; int nextIndex = nextEmpty;
if (((nextEmpty + 1) % values.length) != start) { nextEmpty = (nextEmpty + 1) % values.length; modify = true; } else if (values[nextEmpty] == null) { modify = true; }
if (modify) values[nextIndex] = new Entry(key, value);
return modify; }
@Override public V remove(K key) { // TODO Auto-generated method stub return null; }
@Override public V lookup(K key) { return null; }
@Override public V remove(int n) { // TODO Auto-generated method stub return null; }
@Override public int size() { return 0; }
@Override public V get(int n) { return null; }
@Override public V remove() { // TODO Auto-generated method stub return null; }
@Override public Object[] toArray() { // TODO Auto-generated method stub return null; }
public void searchElement(V id) { for(Object en: values) { Entry e = (Entry) en; if(e.value.equals(id)) { System.out.println(" Found ! Name : " + e.key + " ID : " + e.value); } } }
@Override public String toString() { StringBuilder sb = new StringBuilder();
for(Object en: values) { Entry entry = (Entry) en; sb.append(" Name : " + entry.key + " ID : " + entry.value); } return sb.toString(); } }
public interface List
public abstract boolean add(K key,V value);
public abstract V remove(K key);
public abstract V remove(int n);
public abstract V remove();
public abstract V lookup(K key);
public abstract int size();
public abstract V get(int n);
public abstract Object[] toArray();
public abstract String toString();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
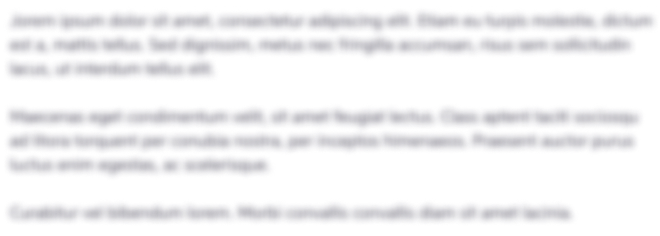
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started