Question
Complete the implementation of the StatsArray.java class based on the UML below. As you complete each method, use your tester application to test them one
Complete the implementation of the StatsArray.java class based on the UML below. As you complete each method, use your tester application to test them one by one. The data array is a partially filled array(only a part of the array will be occupied by elements) (Note: Do not use any of the methods in the Arrays class)
(code is in bold, comments are in italics)
StatsArray |
---|
-data[] : int //an int array named data -currentSize : int //the current size of the array |
+StatsArray(int size) //Constructor. Creates an array of size, size. Initialize currentSize to 0 +getCurrentSize(): int // returns the currentSize of the array +append(target: int ): boolean // if currentSize is less than the size of the array, add the target at the end and return true. Otherwise returns false. +findTotal():int //Calculate and return the total of all values in the array. +findMean():double //Calculate and return the mean of the values in the array. +findMedian():double //Find and return the Median of all the elements in the array. To do this, you need to sort the array and then find the number in the middle. Remember that if there is an even number, the Median is the average of the two numbers in the middle. +findStandardDeviation():double //Calculate and return the standard deviation of the data array +removeValue(target: int) : int //Given a target, it searches the Array for that target. If found returns the index of the target and removes the target from the list. It then adjusts the current size of the array. if not found, it returns -1 +cloneArray( ): int [] // creates a new array of size currentSize. Copy the data array into it. It then returns the array. Use it to make a copy of the data array. +findValue(target: int ): int // searches the array for value. Returns the location(index) of the first occurance of the value. Returns -1 if not found +sortArray ():int [] // Pick your favorite sort and implement it (Do not use the sort method in the Arrays class). It first makes a copy of the data array, sort the copy and returns the sorted copy +swap (array: int[], index1:int ,index2:int): void // swap the array values at index1 and index2. Use this method in sortArray +display():void //if currentSize is greater than 10 then displays mot more than 10 elements (0-9) of the array with the index in [] brackets +toFile(fileName: String):void // Write the data array to fileName file. Elements are separated by comma. Throws an exception if the file can't be created. +toString(): String // Returns a String containing the total, mean, median, and Standard Deviation rounded to at least 3 decimal places. +fromFile(fileName: String):void // Read the array elements from the file into the data array. Throws an exception if the file can't be opened for reading. Assume that the file has 2 or more scores |
Now use the provided StatsArrayDriver.java to test your implementation. Make sure to write complete Javadoc comments throughout the class. Generate javadocs and zip the doc folder along with the source files.
Hint: You may want to use selection sort to sort the array. Make sure to make a copy of the data array and then sort the copy and return it.
Example Output |
---|
How many scores do you want to store in the array? 5 Enter 5 scores between 0 -100 inclusive 45 67 90 100 89 E.x.a.m Scores --------------- [0]45 [1]67 [2]90 [3]100 [4]89 The E.x.a.m Statistics --------------- Total: 391.000 Mean: 78.200 Median: 89.000 StdDev: 19.793
You have a perfect score Enter a score to be removed: 67 Found and Removed from the index: 1 The new stats are: --------------- Total: 324.000 Mean: 81.000 Median: 89.500 StdDev: 21.225
----- sorted array---- [ 0 ]45 [ 1 ]89 [ 2 ]90 [ 3 ]100 Adding two more scores Added Added The current size of the array : 6 Array is full Removing 10 random values Found and removed Found and removed Found and removed Found and removed Found and removed The current size: 95 The new stats are: ----------------- Total: 4913.000 Mean: 51.716 Median: 53.000 StdDev: 30.713
Enter the file Name to store the array: scores.txt File is written. Good bye
Scores from File: ---------------- [0]45 [1]90 [2]100 [3]89 [4]90 [5]100 [6]10 [7]89 [8]57 [9]86 |
StatsArrayDriver.java |
---|
package module2project1updated; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Random; import java.util.Scanner; public class StatsArrayDriver { public static void main(String[] args) throws FileNotFoundException { Scanner scan = new Scanner(System.in); final int SIZE = 100; StatsArray newArray = new StatsArray(SIZE); int index = 1; int numScores; String fName; Random rand = new Random(); System.out.print("How many scores do you want to store in the array? "); numScores = scan.nextInt(); System.out.println("Enter " + numScores + " scores between 0 -100 inclusive"); while (index <= numScores) { newArray.append(scan.nextInt()); index++; } System.out.println("E.x.a.m Scores"); System.out.println("---------------"); newArray.display(); System.out.println("The E.x.a.m Statistics"); System.out.println("---------------"); System.out.println(newArray.toString()); // Testing findValue if (newArray.findValue(100) != -1) { System.out.println("You have a perfect score"); } else System.out.println(" You DO NOT have a perfect score"); // Testing remove Value System.out.print("Enter a score to be removed: "); index = newArray.removeValue(scan.nextInt()); if (index != -1) { System.out.println("Found and Removed from the index: " + index); System.out.println("The new stats are: "); System.out.println("---------------"); System.out.println(newArray); } else System.out.println("Not found"); System.out.println("----- sorted array----"); int[] sortedArray = newArray.sortArray(); for (int i = 0; i < newArray.getCurrentSize(); i++) { System.out.println("[ " + i + " ]" + sortedArray[i]); } // Testing append and if the array can grow System.out.println("Adding two more scores"); if (newArray.append(90)) System.out.println("Added"); if (newArray.append(100)) System.out.println("Added"); System.out.println("The current size of the array : " + newArray.getCurrentSize()); // Testing if refused to add more after adding the 100th element for (int i = 0; i < SIZE; i++) { if (!newArray.append(rand.nextInt(101))) { System.out.println("Array is full"); break; // break once the array is full } } System.out.println("Removing 10 random values "); for (int i = 0; i < 10; i++) { if (newArray.removeValue(rand.nextInt(101)) != -1) { System.out.println("Found and removed"); } } System.out.println("The current size: " + newArray.getCurrentSize()); System.out.println("The new stats are: "); System.out.println("-----------------"); System.out.println(newArray); // Testing toFile and fromFile System.out.print("Enter the file Name to store the array: "); fName = scan.next(); try { newArray.toFile(fName); System.out.println("File is written. Good bye"); } catch (IOException e) { // TODO Auto-generated catch block System.out.println(" ERROR: File cannot be opened"); } System.out.println("Scores from File"); System.out.println("----------------"); newArray.fromFile(fName); newArray.display(); } } /* * Output of the run * * E.x.a.m scores --------------- [0] 41 [1] 37 [2] 21 [3] 11 [4] 57 [5] 85 [6] 92 * [7] 77 [8] 73 [9] 93 The E.x.a.m Statistics --------------- Total: 587 Mean: * 58.7 Median: 65.0 Mode: 92 StdDev: 28.3 You DO NOT have a perfect score Enter * a number to be removed: 11 Found and Removed from the index: 3 E.x.a.m scores * --------------- [0] 41 [1] 37 [2] 21 [3] 57 [4] 85 [5] 92 [6] 77 [7] 73 [8] * 93 [9] 0 The new stats are: --------------- Total: 576 Mean: 57.6 Median: * 65.0 Mode: 92 StdDev: 30.3 */ |
Note: The periods in "e.x.a.m" are there because it's a forbidden word. Please remove these periods from each instance of this word when posting your answer, thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
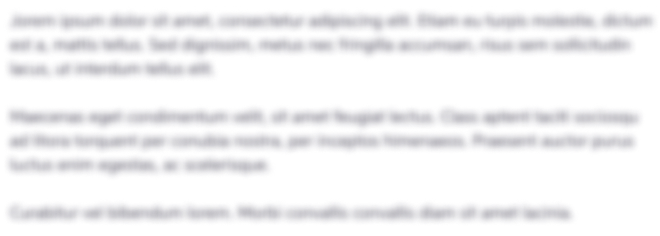
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started