Question
Complete the minimum assignment and the optional section. Ignore the discussion board section. Below is also some code to get started. #include #include using namespace
Complete the minimum assignment and the optional section. Ignore the discussion board section. Below is also some code to get started.
-
#include
-
#include
-
-
using namespace std;
-
-
// class Book
-
// with three private data fields: book title, author, copyright, and price
-
// four public methods to retrieve fields (called "getters")
-
// and one public non-default constructor
-
-
class Book {
-
-
public:
-
-
// member function prototypes
-
void assign (string, string, int, float); // this is your constructor
-
string getTitle();
-
string getAuthor();
-
int getCopyRightYear();
-
float getPrice();
-
-
-
private:
-
-
// data members
-
string title;
-
string author;
-
int copyRightYear;
-
float price;
-
};
-
-
-
// these are the actual member functions
-
-
// this member function is a "constructor" that will create a new book
-
void Book::assign (string bookTitle, string bookAuthor, int bookDate, float bookPrice) {
-
title = bookTitle;
-
author = bookAuthor;
-
copyRightYear = bookDate;
-
price = bookPrice;
-
}
-
-
// this member function is a "getter" that will retrieve that book title value
-
string Book::getTitle() {
-
return title;
-
}
-
-
// this member function is a "getter" that will retrieve the primary book author value
-
string Book::getAuthor() {
-
return author;
-
}
-
-
// this member function is a "getter" that will retrieve the year the book was copyrighted
-
int Book::getCopyRightYear() {
-
return copyRightYear;
-
}
-
-
// this member function is a "getter" that will retrieve the list price of the book
-
float Book::getPrice() {
-
return price;
-
}
-
-
-
-
int main()
-
{
-
-
cout
-
-
// Set up space to create 5 instances of the class Book to use with our constructor
-
Book b1, b2, b3, b4, b5;
-
-
// Use our constructor to create the first book, replace my book below with info on your favorite book, use b1
-
b1.assign ("C++ for Dummies", "Stephen R Davis", 1998, 29.99);
-
-
cout
-
cout
-
cout
-
-
// Use the constructor again to create another book, again, replacing my book below with one your favorite books, use b2
-
b2.assign ("C++ - The Complete Reference", "Herbert Schildt", 2003, 52.99);
-
-
cout
-
cout
-
cout
-
-
// use constructor (its called assign) again to create and then print information about book 3, another favorite book of yours ... remember to use b3
-
-
// use constructor again to create and then print information about book 4, your fourth favorite book ... remember to use b4
-
-
// use constructor again to create and then print information about book 5, your fifth favorite book ... remember to use b5
-
-
return (0);
-
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
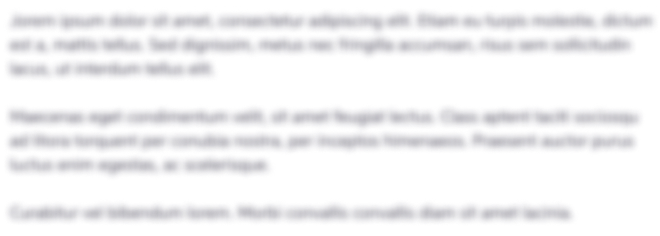
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started