Question
complete thr following methods you can use recursion using the binaryNode class @Override public T getMin() { return null; } @Override public T getMax() {
complete thr following methods you can use recursion using the binaryNode class
@Override
public T getMin() {
return null;
}
@Override
public T getMax() {
return null;
}
@Override
public T getNextToSmallest() {
return null;
}
@Override
public T getNextToLargest() {
return null;
}
@Override
public T getMedian() {
return null;
}
@Override
public T getIthValue(int i) {
return null;
}
@Override
public int getSortedIndex(T value) {
return 0;
}
class BinaryNode
private T data;
private BinaryNode
private BinaryNode
private int numNodesLeft, numNodesRight;
public BinaryNode() {
this(null); // Call next constructor
} // end default constructor
public BinaryNode(T dataPortion) {
this(dataPortion, null, null); // Call next constructor
} // end constructor
public BinaryNode(T dataPortion, BinaryNode
data = dataPortion;
leftChild = newLeftChild;
rightChild = newRightChild;
} // end constructor
/**
* Retrieves the data portion of this node.
*
* @return The object in the data portion of the node.
*/
public T getData() {
return data;
} // end getData
/**
* Sets the data portion of this node.
*
* @param newData
* The data object.
*/
public void setData(T newData) {
data = newData;
} // end setData
/**
* Retrieves the left child of this node.
*
* @return The nodes left child.
*/
public BinaryNode
return leftChild;
} // end getLeftChild
/**
* Sets this nodes left child to a given node.
*
* @param newLeftChild
* A node that will be the left child.
*/
public void setLeftChild(BinaryNode
leftChild = newLeftChild;
} // end setLeftChild
/**
* Detects whether this node has a left child.
*
* @return True if the node has a left child.
*/
public boolean hasLeftChild() {
return leftChild != null;
} // end hasLeftChild
/**
* Retrieves the right child of this node.
*
* @return The nodes right child.
*/
public BinaryNode
return rightChild;
} // end getRightChild
/**
* Sets this nodes right child to a given node.
*
* @param newRightChild
* A node that will be the right child.
*/
public void setRightChild(BinaryNode
rightChild = newRightChild;
} // end setRightChild
/**
* Detects whether this node has a right child.
*
* @return True if the node has a right child.
*/
public boolean hasRightChild() {
return rightChild != null;
} // end hasRightChild
/**
* Detects whether this node is a leaf.
*
* @return True if the node is a leaf.
*/
public boolean isLeaf() {
return (leftChild == null) && (rightChild == null);
} // end isLeaf
/**
* Counts the nodes in the subtree rooted at this node.
*
* @return The number of nodes in the subtree rooted at this node.
*/
public int getNumberOfNodes() {
int leftNumber = 0;
int rightNumber = 0;
if (leftChild != null)
leftNumber = leftChild.getNumberOfNodes();
if (rightChild != null)
rightNumber = rightChild.getNumberOfNodes();
return 1 + leftNumber + rightNumber;
} // end getNumberOfNodes
/**
* Computes the height of the subtree rooted at this node.
*
* @return The height of the subtree rooted at this node.
*/
public int getHeight() {
return getHeight(this); // Call private getHeight
} // end getHeight
private int getHeight(BinaryNode
int height = 0;
if (node != null)
height = 1 + Math.max(getHeight(node.getLeftChild()), getHeight(node.getRightChild()));
return height;
} // end getHeight
/**
* Copies the subtree rooted at this node.
*
* @return The root of a copy of the subtree rooted at this node.
*/
public BinaryNode
BinaryNode
if (leftChild != null)
newRoot.setLeftChild(leftChild.copy());
if (rightChild != null)
newRoot.setRightChild(rightChild.copy());
return newRoot;
} // end copy
} // end BinaryNode
Step by Step Solution
There are 3 Steps involved in it
Step: 1
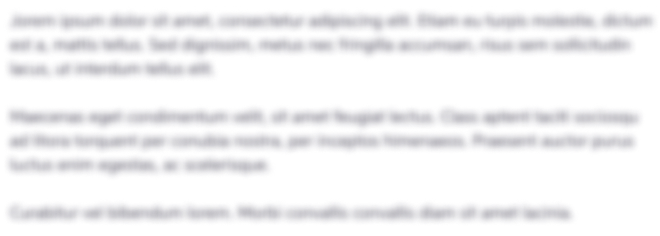
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started