Question
CompSci 251: Assignment 6- JAVA Due 3/27, 2017 10:00am Topics Covered: Classes with arrays, multiple classes 1 Introduction So, its already 2018 and time for
CompSci 251: Assignment 6- JAVA
Due 3/27, 2017 10:00am
Topics Covered: Classes with arrays, multiple classes
1 Introduction
So, its already 2018 and time for the Winter Olympics. You will write a program to keep track of standings in a new kind of speed skating competition. Skaters will race twice and they will be compared by the lower of the two times they get. Skaters may get disqualified during the race process.
2 Program tasks
You will write a program that keeps track of the race results and can print reports of the standings at any time(you can use the toString method in SkateRace to return a string that represents the current stand- ing). UML diagrams for your classes appear below. Notice that the SkateRace class holds an Array of Skaters.
Your program should ask the user to enter how many skators he wants to enter. The program then will have three phases:
The first phase corresponds to the first half of the race. Your program will read in the name, State, and first race time for a series of skaters.
After each entry, your program will print the current leader(the skater who has the lowest time in the array so far).
The phase will end once the user enters all the skaters in to the array. The complete standings will be printed along with the leader who has the lowest race time(assume each racer has a unique race time)
The second phase corresponds to the second half of the race. The program will read in the name and second race time of the skaters and print the leader after each update.
The phase will end once the user done updating and the complete standings will be printed.
In the third phase, your program will read in the names of any skaters that have been disqualified and
remove them from the race. Your program will also print the overall standings at the end of the race.
1
Here are the UML diagrams for the classes we expect you to use. This program structure is pretty common and standard. You have a simple data element class (Skater), a larger class that holds the data elements (SkateRace), and a driver class (RaceDriver) that makes the classes do things.
Skater |
-name: String //only one word, no spaces -state: String //only one word, no spaces -time: double |
+ public Skater(name: String, state: String ,time: double ) + getName(): String + getTime(): double + getState(): String + setTime(time: double): void + toString(): String |
SkateRace |
skaters: Skater[ ] size: int// companion variable to keep track of current size of skaters array |
+ SkateRace(numberOfSkaters: int)//constructor + getSize():int//accessor for size + getLength():int//returns the length of the array of Skaters + addSkater(skater: Skater) : void + getSkater(name: String): Skater + getSkater(position: int) : Skater + updateSkater(name: String, secondTime: double): boolean + removeSkater(name: String) : void + toString(): String |
RaceDriver |
+main(String[] args) : static void -phase1(stdIn: Scanner, sRace: SkateRace): void -phase2(stdIn: Scanner, sRace: SkateRace): void -phase3(stdIn: Scanner, sRace: SkateRace): void #findLeader(sRace: SkateRace ): Skater |
You can assume that the two Strings in the Skater class will only hold single words. This simplifies reading the input from the user, because you can use the Scanner.next() method to read the Strings (instead of Scanner.nextLine())
The addSkater method adds the skater to the array and increments the size, you should check if there is a free space in the array before adding, if not do nothing.
The SkateRace class has two overloaded getSkater methods, getSkater(String name) and getSkater(int position). getSkater(String name) returns the skater whose name matches the argument(assuming unique name for each skater). If the name does not exist, the getSkater(String name) method should return null.
For getSkater(int position), the method should check first if the position is a valid index within the array(position>=0 and position Your driver code that uses getSkater, needs to check for null return values or your program could crash. 2 3 The updateSkater method returns a boolean. It should find the Skater with the given name and, if the new race time is lower, it should change that Skaters time. It should return true when the Skater is found and updated. It should return false either when the name given doesnt match any Skaters or the new race time is larger than the current skaters race time . The removeSkater method works differently from updateSkater and doesnt return anything. If the name isnt found, it does nothing. The driver will have to use the getSkater method to check whether a Skater is already in the race, when checking names to be disqualified. Your driver class should have four helper methods: one for each phase and findLeader. This design should simplify your main method(we asked you to make the access modifier for findLeader to be protected just for testing purposes). The findLeader method returns the skater who has the lowest time among the other skaters in the array argument (assume each racer has a unique race time). The toString method in SkateRace class should return a string for the content of the array(you can rely on the toString for each object inside the array), make sure to handle partially filled array. Sample Output The comments below are not part of the output, I just put them for your understanding 3 Goodbye! Please enter how many Skater you want to enter:5 Welcome to Skate Race Program
Starting First Phase Enter Skaters data as: Name State Time Enter Skater data: James CA 9.3 Current Leader: James (CA) 9.3
Enter Skater data: Mike VA 10.1 Current Leader: James (CA) 9.3
Enter Skater data: Alex IL 10.0 Current Leader: James (CA) 9.3
Enter Skater data: Sam WI 9.2 Current Leader: Sam (WI) 9.2
Enter Skater data: Dan FL 9.5 Current Leader: Sam (WI) 9.2
Current Standings:
The Leader is : Sam (WI) 9.2
1: James (CA) 9.3 2: Mike (VA) 10.1 3: Alex (IL) 10.0 4: Sam (WI) 9.2 5: Dan (FL) 9.5
Starting Second Phase
Enter the name and updated time of the skater you want to update or "quit": Foo 9.0 Skater Mile is not updated!
Enter the name and updated time of the skater you want to update or "quit": Mike 9.0 Skater Mike is updated and the new Skater data: Mike (VA) 9.0
Enter the name and updated time of the skater you want to update or "quit": Dan 15.1 Skater Dan is not updated!
Enter the name and updated time of the skater you want to update or "quit": quit Current Standings: The Leader is : Mike (VA) 9.0
1: James (CA) 9.3 2: Mike (VA) 9.0 3: Alex (IL) 10.0 4: Sam (WI) 9.2 5: Dan (FL) 9.5
Starting third/disqualification phase Enter the name of the skater you want to disqualify or "quit" Dan Enter the name of the skater you want to disqualify or "quit" Alex Enter the name of the skater you want to disqualify or "quit" Mike Enter the name of the skater you want to disqualify or "quit" quit
Final Standings: The Leader is : Sam (WI) 9.2
1: James (CA) 9.3 2: Sam (WI) 9.2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
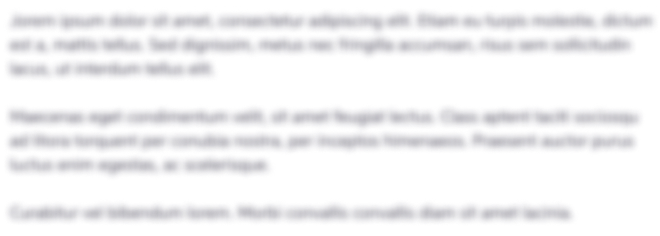
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started