Question
Computer Science 1- Java Please include all the code for my reference. This is my set up. PLEASE add comments for easier understanding. Paste the
Computer Science 1- Java
Please include all the code for my reference. This is my set up. PLEASE add comments for easier understanding. Paste the whole code as plain text as the answer. Only make changes to file "Light.java" and "Strand.java". PLEASE don't import anything new and keep it simple. The output of the program has to be the same as the sample runs in the rubric.
---------------------------------------------------------------------------------
public class Light { private boolean on; private boolean burntOut; private String color = ""; public Light() { } public Light(boolean o, boolean b, String c) { } public String toString() { } public void flip() { } public String getColor() { } public void setColor(String c) { } public boolean isOn() { } public void burnOut() { } public boolean isBurntOut() { } }
--------------------------------------------------------------------------------------
import java.util.ArrayList; public class Strand { private ArrayListstrand; public Strand() { } public Strand(int n) { } public String toString() { } public void setColor(String c) { } public void setMulti() { } public void turnOn() { } public void turnOff() { } public void burnOut(int i) { } public int getSize() { } }
-------------------------------------------------------------------------------------------------
public class LightStrandTester { public static void main(String[] args) { //Tests for Light class: //************************************************************************* // 1. Test Light() //************************************************************************* Light light1 = new Light(); System.out.println("1. Create light one with default constructor"); System.out.println(light1); System.out.println(" Test burnOut on light 1"); light1.burnOut(); System.out.println(light1); //************************************************************************* // 2. Test Light(boolean b, boolean o, String c) //************************************************************************* System.out.println(" 2. Create Light2 with other constructor"); Light light2 = new Light(true, true, "GreeN"); System.out.println(light2); //************************************************************************* // 3. Test flip() //************************************************************************* // light3 is currently on and not burnt out. Lets flip the light off and on and see if it works properly.System.out.println(" 4. Test flip()"); Light light3 = new Light(); System.out.println(" 3. Create Light3 with default constructor"); System.out.println(light3); light3.flip(); System.out.println("Flip switch to turn Light3 off"); System.out.println(light3); light3.flip(); System.out.println("Flip switch to turn Light3 on"); System.out.println(light3); // Try to flip light1 on - this should fail since light1 is burnt out. light1 should stay off System.out.println("Try to flip light1 on - this should fail since light1 is burnt out."); light1.flip(); System.out.println(light1); //************************************************************************* // 4. Test isOn() //************************************************************************* System.out.println(" 4. Test isOn()"); // We know light1 is off, and light3 is on. Verify that the method isOn reports this correctly. if (!light1.isOn()) { System.out.println("*** PASS: isOn() working properly"); } else { System.out.println("*** FAIL: isOn() not working properly"); } if (light3.isOn()) { System.out.println("*** PASS: isOn() working properly"); } else { System.out.println("*** FAIL: isOn() not working properly"); } //************************************************************************* // 5. Test getColor() //************************************************************************* System.out.println(" 5. Test getColor()"); if (light1.getColor().equals("white")) { System.out.println("*** PASS: getColor() working properly"); } else { System.out.println("*** FAIL: problem with getColor()"); } //************************************************************************* // 6. Test setColor(String) //************************************************************************* System.out.println(" 6. Test setColor(String)"); light1.setColor("red"); System.out.println("*** " + testLightColor(light1, "red")); light1.setColor("BLUE"); // should set light to blue System.out.println("*** " + testLightColor(light1, "blue")); light1.setColor("yellow"); // yellow is not allowed, should set light to white System.out.println("*** " + testLightColor(light1, "white")); System.out.println(" below are tests for Strand class"); // ************************************************************************* // 7. Test Strand() // ************************************************************************* System.out.println("7. Test the default constructor Strand()"); Strand strand1 = new Strand(); if (strand1.getSize() == 1) System.out.println("*** PASS: Strand() creates a strand of size 1"); else System.out.println("*** FAIL: Strand() creates a strand of size " + strand1.getSize() + ", when a strand of size 1 is expected."); // *********************************** // 8. Test Strand(n) // *********************************** System.out.println(" 8. Test the constructor Strand(n)"); // Try to create a strand of lights with 0 bulbs Strand emptyStrand = new Strand(0); if (emptyStrand.getSize() == 1) System.out.println("*** PASS: Strand(0) creates a strand of size 1"); else System.out.println("*** FAIL: Strand(0) creates a strand of size " + emptyStrand.getSize() + ", when a strand of size 1 is expected."); // Try to create a strand of lights with a negative number Strand negativeStrand = new Strand(-7); if (negativeStrand.getSize() == 1) System.out.println("*** PASS: Strand(-7) creates a strand of size 1"); else System.out.println("*** FAIL: Strand(-7) creates a strand of size " + negativeStrand.getSize() + ", when a strand of size 1 is expected."); // Try to create a strand of lights with a positive number Strand strandWithFiveBulbs = new Strand(5); if (strandWithFiveBulbs.getSize() == 5) System.out.println("*** PASS: Strand(5) creates a strand of size 5"); else System.out.println("*** FAIL: Strand(5) creates a strand of size " + strandWithFiveBulbs.getSize() + ", when a strand of size 5 is expected."); // Verify that all the light bulbs are initialized properly System.out.println(" Strand(5) should be all on and white"); System.out.println(strandWithFiveBulbs); // *********************************** // 9. Test setColor(String) // *********************************** System.out.println(" 9. Test setColor(String)"); // All of the bulbs in our strandWithFiveBulbs are white. Set them to green. strandWithFiveBulbs.setColor("green"); System.out.println("Strand(5) should be all on and green"); System.out.println(strandWithFiveBulbs); // All of the bulbs in our strandWithFiveBulbs are white. Set them to multi. strandWithFiveBulbs.setMulti(); System.out.println("Strand(5) should be all on and multi - red, green, blue, red, green"); System.out.println(strandWithFiveBulbs); // Now try to set them to a color that is not supported. This should // cause all the bulbs to be set back to white. strandWithFiveBulbs.setColor("pink"); System.out.println("Strand(5) should be all on and white again"); System.out.println(strandWithFiveBulbs); // *********************************** // 10. Test turnOff() // *********************************** System.out.println(" 10. Test turnOff()"); strandWithFiveBulbs.turnOff(); System.out.println("Strand(5) should be all off and white"); System.out.println(strandWithFiveBulbs); // *********************************** // 11. Test turnOn() // *********************************** System.out.println(" 11. Test turnOn()"); strandWithFiveBulbs.turnOn(); System.out.println("Strand(5) should be all on and white"); System.out.println(strandWithFiveBulbs); // *********************************** // 12. Test burnOut(int) // *********************************** System.out.println(" 12. Test burnOut(n)"); strandWithFiveBulbs.burnOut(0); System.out.println("the first bulb in Strand(5) should be burnt out and off"); System.out.println(strandWithFiveBulbs); } // Private helper methods private static String testLightColor(Light light, String c) { if (!light.getColor().equals(c)) { return "FAIL: color is not set correctly (color should be set to " + c + ", but it is set to " + light.getColor() + ")"; } else { return "PASS: color is set correctly (" + light.getColor() + ")"; } } }
-------------------------------------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
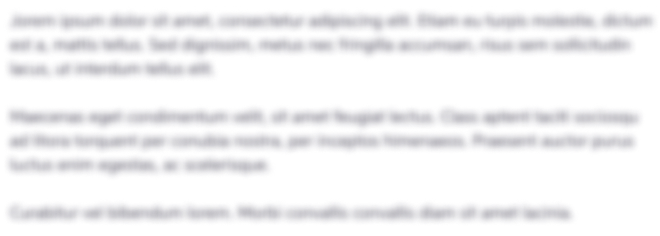
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started