Question
Computer Science 111 Introduction to Algorithms and Programming: Java Programming Project #5 Arrays, Files, and Sorting (15 points) Phase 1 - Numbers Posted below in
Computer Science 111 Introduction to Algorithms and Programming: Java
Programming Project #5 Arrays, Files, and Sorting (15 points)
Phase 1 - Numbers
Posted below in the information sections is the name of an array, the name of an input file, the name of an output file, and a list of integer numbers of which the first one will be an exact count of the remaining numbers in the list. So if the first number in the list is 500, there will be 500 additional numbers in the list.
Copy and paste the number data, including the count into a plain text file with the name of the input file given. Your mission, if you choose to accept it, is to write a console program ( NO GUI ). Open and read the first line of this input file and create an integer array with the name of the array given and a size to match the first line of the file. So if the first line of the file contains 500, the array will have 500 slots. Read the remaining numbers into the array. Write a method named sort, which takes the array as a parameter, and sorts it from low to high. You MUST write your own sort method, do not use a built in sort method. When the sort is complete, open and write a file with the name of the output file, the count will be first, followed by the sorted list of numbers (one per line). Finally, write a simple loop to compute the average of the numbers in the list (not including the count). Output this average as the last line of the output file. Be sure to use a double data type for the sum and average variables.
:Your information for this assignment is:
The array name is data_array
The input file name is testnums.txt
The output file name is outputnumbers.txt
This is just simple test data, get your program working with this data before trying the larger data set. 11 12345 23456 123 4567 123456 7654 999 3453 997733 43 654321
Full Data:491 1714 8761 1305 2527 1431 2488 4814 3268 4009 550 545 570 1913 6769 3899 2467 313 3644 6878 6808 5474 9584 6425 5753 1402 5199 7076 9829 8775 5819 3654 490 4580 4859 2918 5912 7248 7632 9081 1258 8083 9526 1728 9896 6296 5528 2364 6510 9073 9142 3319 4547 8727 9645 300 130 4844 7277 9859 3620 3096 3513 4010 7577 8273 6828 3489 5521 4460 2570 6680 2544 2097 8308 2441 8294 3837 4706 4805 2910 3849 8024 7358 2576 7670 7558 2606 2515 4836 2465 6035 7832 5879 9945 5410 4152 6773 8800 9574 1234 1370 6254 3679 3368 4563 6020 1662 8300 726 6368 1211 4475 4393 8469 6952 2063 6027 9458 4478 863 1924 513 8596 7703 459 4007 1855 7133 2807 1429 8268 4078 7583 1947 7346 2147 7868 8909 447 8495 5277 1558 2971 9570 9927 9823 1634 5955 9282 6012 6719 1206 6426 5316 8809 6785 9224 665 3919 2031 1995 2187 6009 9479 4034 3356 1626 1902 2265 1973 397 7443 3432 3268 7013 3360 3092 8547 9216 2374 4560 5936 3480 986 1253 2290 7672 477 2856 1591 2408 4751 3679 8318 4231 7613 1674 5757 9416 3839 7631 9714 1282 1064 2983 8196 4324 5975 6744 3541 8249 1304 9377 1730 2191 631 3921 9764 1008 6677 1356 3317 1429 4935 1635 5561 2549 3210 1318 1965 6950 8850 1679 8133 9814 4562 6329 4139 537 3074 7580 8687 4278 6958 418 6370 7489 4239 6134 8398 917 7390 1715 2247 2326 3251 7708 4775 6361 8927 6640 3312 7777 8220 1445 7591 2782 7675 1731 3220 749 9211 1908 4928 6170 2226 1298 3660 6365 7333 2058 7183 4723 3674 9330 6949 6825 7039 1725 3187 5966 8265 6399 3743 6485 7744 1335 9168 5419 2966 2389 6069 2178 4197 997 8249 6323 2195 1909 2689 9429 3867 9772 4152 7442 9103 1102 4267 6142 2727 7355 2108 993 3754 5752 7379 1499 6988 6547 6819 9855 8836 2888 2033 3034 3786 283 9257 5882 2092 1946 5311 5860 1719 9364 3302 822 466 7470 6864 3094 4825 8873 3988 8479 4626 1367 9879 1614 7815 6698 1469 6652 9487 3403 9586 3273 3586 8844 9055 5579 791 4366 1439 2410 3730 4641 3132 4097 2111 9897 7092 6836 8770 1080 5316 3396 2348 5195 4911 164 1894 6280 6716 1381 9584 6303 4554 3170 5148 3609 8649 5839 7876 9989 8149 1606 4630 1282 5603 6642 1179 2695 3479 9850 3676 8696 3246 5924 3892 8057 5988 5686 4338 2705 6967 3922 8909 1522 6993 4057 5032 5643 9796 2908 5632 7946 4414 263 9128 9918 6805 308 2614 285 158 6190 8881 3305 2115 2773 1363 8004 8360 5601 709 5327 9424 9519 6750 6417 3576 1782 2061 3373 4590 7593 1320 8905 7757 449 8824 4562 658 1438 4748 716 7529 3629 3922 9544 6303 5185 7548 4663 787 8158 9891 211 7677 6641
Phase 2 - Strings
Posted Below is the name of an array, the name of an input file, the name of an output file, and a list of Strings of which the first one will be an exact count of the remaining Strings in the list. So if the first string in the list is 200, there will be 200 additional Strings in the list.
Copy and paste the String data, including the count into a plain text file with the name of the input file given. Your mission, if you choose to accept it, is to write a console program ( NO GUI ). Open and read the first line of this input file and create a String array with the name of the array given and a size to match the first line of the file. So if the first line of the file contains 200, the array will have 200 slots. Read the remaining Strings into the array. Write a method named sortstrings, which takes the array as a parameter, and sorts it from low to high. When the sort is complete, open and write a file with the name of the output file, the count will be first, followed by the sorted list of Strings (one per line). Finally, output the String in the middle of the array as the last line of the output file. So if there are 11 slots in the array, the middle item will be in slot 5 of the sorted array. If there are an even number of slots take the lower slot number. So if there are 10 slots in the array, the middle item will be in slot 4 of the sorted array.
Your String information for this project is:
The array name is strdata_array
The input file name is teststrs.txt
The output file name is outputstrings.txt
This is just simple test data, get your program working with this data before trying the larger data set.
9 Jones, Chris C Ferguson, Tom J Pryor, Richard P Williams, Robin M Seinfeld, Jerry P David, Larry L Kinneson, Sam A Smith, Chris P Jones, Tom J
Actual Data
237 Clifford, Tammie D Dianne, Chuck G Joeseph, Edd K Abbot, Austin B Candy, Oscar J Darcy, Al J Peters, Ophela O Xavier, Nancy O Berta, Jo S Zeke, Tammie P Hank, Alec L Joey, Elroy P Penny, Ron X Brett, Sandy Y Clifford, Zima Q Leroy, Dominic Q Wilson, Victor I Jack, Tammy Z Allen, Andy M Quincy, Zeke J Fredricks, Fredrick K Ronald, Sindy R Geoff, Kerry L Dianne, Ned G Cane, Sindy G Toms , Geo M Joeseph, Elroy J Sandra, Paula G Robby, Robbie G Dianne, Jerry G Mick, Ronny P Zilly, Kane K Ottaman, Ted Y Brett, Colin Q Hank, Frank L Mick, Carrie V Austin, Mick C Izzy, Holly V Paul, Don P Wilma, Hal C Candy, Alec W Robby, Abbot K Crawford, Paul H Berta, Sage K Ronald, Clifford T Donald, Robby X Abel, Tom N Colins, Ellen Y Zilly, Zed E Michaels, Vincent P Ottaman, Bob Q Karry, Ted R Woods, Ann K Cynthia, Dan F Mick, Woodrow K George, Carrie E Dominic, Clifford A Robbie, Franklin I Paul, Frank H Usher, Vinny F Jack, Lenny I Benjamin, Berta F Robby, Alec H Colins, Danella M Hank, Sindy K Rory, Cece O Will, Geo T Zed, Berta C Franks, Sandie L Michaels, Nuckle J Toms , Berta Y Clifford, Daniel B Henrietta, Yancey C Otto, Chris I Berta, Jackson E Leroy, Dede I Bracken, Clifford R Victor, Cece V Zeke, Irv Q Irving, Austin H Ronald, Tommy K Paul, Abbot Q Robbie, Habib P Theodore, Benjamin T Clifford, Danny F Francis, Rory M Nikko, Usher R Francis, Ralene V Berta, Ron H Robby, Cindy I Alexstein, Vin E Joey, Mickie F Brett, Fredrica Q Quincy, Don S Joe, Francis Y Paul, Ophela S Clifford, Edward P Toms , Nancy J Oscar, Rory D Willa, Kane H Dedra, Margaret F Irving, Beatrice V Nikko, Danny E Elroy, Woody J Zeke, Hank T Wilson, Brett K Nick, Didi T Cane, Beatrice J Minnie, Paula D Paul, Nikko R Zima, Ted H Cane, Elaine J Allen, Vinny P Cane, Clifford N Mickie, Cece Y Francis, Barney A Robbie, Otto H Joeseph, Theodore K Usher, Ickels D Zima, Crawford U Otto, Charles R Wilma, Franklin Y Francis, Fiona W Wilson, Larry B Ice, Chuck G Henry, Ickels H Humprey, Dom Z Pete, Ophela W Beatrice, Kelly I Danella, Jo M Andrews, Cane Q Ottaman, Colin Y Nikko, Irv I Kim, Fredrica W Mickie, Robby Z Sally, Crawford L Brett, Lawrence R Bebe, Di I Zima, Francis W Francis, Lenny J Sandra, Marge O Icabod, Di S Henrietta, Usher D Paul, Harry M Marge, Zed H Marge, Sam L Allen, Victor Z Oprah, Allen G Allen, Alice P Vinny, Elisabeth F Elaine, Colin U Thomas, Lawrence B Karry, Woody Y Ottaman, Nancy J Clifford, Don I Jack, Ron D Vinny, Ralene A Victor, Irv B Berta, Ice B Ronald, Fredrica K Xavier, Micky N Ronald, Icabod B Sally, Zeke A Robby, Nan Q Brett, Sondra W Jack, Elroy P Minnie, Cynthia J Nancy, Ann V Otto, Clifford H Quincy, Willa R Bebe, Ulysiss H Nicolas, Nicolas T Paul, Icabod T Pete, Donald K Harold, Fred F Cane, Sam N Sage, Francis R Candy, Wilma T Aliceman, Micky Y Fiona, Fiona W Yancey, Icabod T Dianne, Betty K Zima, Ronny W Penny, Jackson C Bebe, Sal L Georgia, Austin F Theodore, Tom P Karry, Abbot O Evens, Anne E Candy, Francis O Alecson, Elisabeth A Sally, Linda C Danella, Leroy W Benjamin, Elroy J Cynthia, Harry F Nikko, Paula G Ulysiss, Linda C Jack, Bebe D Aliceman, Fredrica E Cane, Kerry O Robert, Margaret W Robbie, Henry L Theodore, Nancy A Penny, Pauline H Barney, Vin R Franks, Kerry L Lawrence, Hank A Oscar, Tommie C Samual, Tammy L Zed, Alexander A Kane, Sandy P Henrietta, Larry A Thomas, Ginny Q Theodore, Bracken J Berta, Dan H Toms , Jack W Dianne, Al G Evens, Nuckle D Harold, Irv Z Sally, Henrietta B Sally, Willa W Icabod, Elisabeth K Izzy, Cece U Benjamin, Andy X Joeseph, Charles C Samual, Wilma P Nan, Danny Q Vincent, Ice H Allen, Sage T Aliceman, Mick E Alexstein, Irv C Will, Tammie L Harold, Eddy P Charles, Hank P Alexander, Usher T Candy, Nuckle S Sams, Ed X
Step by Step Solution
There are 3 Steps involved in it
Step: 1
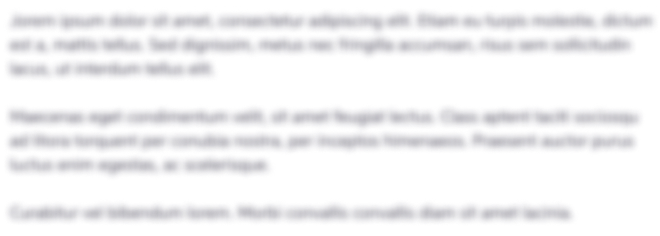
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started