Question
Computer Science - Coding in Python Optimizing a trajectory Callista Cherry Bomb Davidson is a world-famous stunt woman, and she is trying to achieve the
Computer Science - Coding in Python
Optimizing a trajectory
Callista "Cherry Bomb" Davidson is a world-famous stunt woman, and she is trying to achieve the long distance world record human cannonball. She needs your help, though, because she has no idea what angle is best to aim her cannon. You will need to simulate firing the cannon at all integer angles from 1 to 90 inclusive.
Her cannon will shoot her at a velocity of 70ms70ms at a starting height of 5m5m. We will assume an acceleration of gravity g=?9.8ms2g=?9.8ms2. We will simulate her trajectory for 10 seconds in 1,000 steps (so 1,001 states including the initial state.)
Angles
We will simulate 90 different angles from 1??90?1??90?. You will need to find the horizontal xx and vertical yy components of her initial velocity at each angle to simulate in two dimensions.
vx0=v0cos(?)vy0=v0sin(?).vx0=v0cos(?)vy0=v0sin(?).
(Note that you will need to convert from degrees to radians before using the numpy sin and cos functions.)
Drag
To ensure Callista goes as far as possible, we cannot neglect drag in both the vertical and horizontal dimensions. To simplify things a bit, we will assume her surface area is equal in both dimensions. We will need to make use of the drag equation,
FD=?12?v2CDA.FD=?12?v2CDA.
Callista has a surface area of A=0.8m2A=0.8m2 and a mass of mass=65kgmass=65kg. Her costume gives her a drag coeffienct of CD=1.4CD=1.4. The mass density of the air is ?=1.225kgm3?=1.225kgm3. This force will always oppose her travel, so she will experience an acceleration of
axdrag=??CDA2massv2???vxv???.axdrag=??CDA2massv2|vxv|.
(In other words, when she is ascending drag tends to slow her rise, and when she is descending drag tends to slow her fall. An if statement may be useful in implementing this.)
This expression has been changed to more accurate physics. Please double-check your code, and consult the starting code below.
Remember that you will need to simulate this acceleration in both the xx and yy dimensions (both vertical and horizontal.) To simplify things a bit, compute this acceleration in the xx and yy dimensions independently.
Simulation
When Callista hits the ground, she stops. To simulate this, if her altitude is ever less than 0, set her velocity in all directions to zero, her height to zero, and her xx location to its previous value. Her xx location should then remain the same until the end of the simulation. In other words, when she hits the ground, she stops moving until the 10 seconds we are simulating are over.
For each angle, you will need to simulate all 1,000 time steps. Each time you update the state variables, first compute her acceleration (using her previous speed to compute the drag,) then her velocity (using her current acceleration,) then her location (using her current velocity.)
Final answer
After the simulation is complete, we are not yet finished. Callista needs to know the optimum angle in integer degrees (being a cannonball not a mathematician).
Extra fun
Once you have the model finished, you can have fun with it. Plotting her trajectory at each angle produces a striking graph. You can also try changing the parameters to ridiculous values. How far would she travel if the cannon shot her at 1,000 meters per second? How hard would she hit the ground?
STARTER CODE:
# 0. Place your necessary imports here. You may find it useful to be able to plot when debugging and visualizing your result. import numpy as np
# 1. Create a 1D vector named `t` and 2D arrays named `vx`, `vy`, `x`, and `y` to hold the state variables, of size 90 x 1001. t = ???
# 2. Store the angles from 1 to 90 degrees as radians in a variable called `radians`. Use this to initialize the state variables for `vx` and `vy`. m = 90 # angles to fire at angles = ??? radians = angles * 2*np.pi/360
# 3. Define properties like gravity, Callista's surface area, and Callista's mass, and any other parameters you may need as they come up. A = 0.8 # m^2 g = 9.8 # m/s^2 # etc. Note that I expect an `initial_height` and `initial_velocity` below.
# 4. At this point, you should have defined `t`, `x`, `y`, `vx`, `vy`, `radians`, and the properties you need. Now, initialize the starting condition in each array: for i in range(m): y[ i ][ 0 ] = initial_height vx[ i ][ 0 ] = initial_velocity * np.cos( radians[ i ] ) vy[ i ][ 0 ] = ??? # (see "Angles" above)
# 5. Now you are ready to begin the simulation proper. You will need two loops, one over every angle, and one over every time step for that angle's launch. for i in ???: # loop over each angle for j in ???: # loop over each time step # check that the location isn't below the ground; if so, adjust as specified above
# calculate the acceleration including drag (for both x and y, x shown) v = np.sqrt( vx[ i,j-1 ]**2 + vy[ i,j-1 ]**2 ) ax = -( 0.5*rho*C*A/mass ) * v**2 * ( vx[ i,j-1 ] / v )
# calculate the change in position at time `ts` using the current velocities (`vx[ i ][ j ]`) and the previous positions (`x[ i ][ j-1 ]`). This is slightly different from the previous example you solved in an earlier homework.
# 6. The purpose of these calculations was to show which angle yielded the farthest distance. Find this out and store the result in a variable named `best_angle`.
. We will simulate her trajectory for 10 seconds in Her cannon will shoot her at a velocity of 70" at a starting height of 5m. We will assume an acceleration of gravity g = -9.8 1,000 steps (so 1,001 states including the initial state.) Angles We will simulate 90 different angles from 1 90. You will need to find the horizontal x and vertical y components of her initial velocity at each angle to simulate in two dimensions. V.0 = Vecos(2) Vyo = vosin(e). (Note that you will need to convert from degrees to radians before using the numpy sin and cos functions.) Drag To ensure Callista goes as far as possible, we cannot neglect drag in both the vertical and horizontal dimensions. To simplify things a bit, we will assume her surface area is equal in both dimensions. We will need to make use of the drag equation, Fp =-_pv cpa. Callista has a surface area of A = 0.8 m and a mass of mass = 65 kg. Her costume gives her a drag coeffienct of Cp = 1.4. The mass density of the air is p = 1.225 % . This force will always oppose her travel, so she will experience an acceleration of Ardtag = - Blast 2 . We will simulate her trajectory for 10 seconds in Her cannon will shoot her at a velocity of 70" at a starting height of 5m. We will assume an acceleration of gravity g = -9.8 1,000 steps (so 1,001 states including the initial state.) Angles We will simulate 90 different angles from 1 90. You will need to find the horizontal x and vertical y components of her initial velocity at each angle to simulate in two dimensions. V.0 = Vecos(2) Vyo = vosin(e). (Note that you will need to convert from degrees to radians before using the numpy sin and cos functions.) Drag To ensure Callista goes as far as possible, we cannot neglect drag in both the vertical and horizontal dimensions. To simplify things a bit, we will assume her surface area is equal in both dimensions. We will need to make use of the drag equation, Fp =-_pv cpa. Callista has a surface area of A = 0.8 m and a mass of mass = 65 kg. Her costume gives her a drag coeffienct of Cp = 1.4. The mass density of the air is p = 1.225 % . This force will always oppose her travel, so she will experience an acceleration of Ardtag = - Blast 2Step by Step Solution
There are 3 Steps involved in it
Step: 1
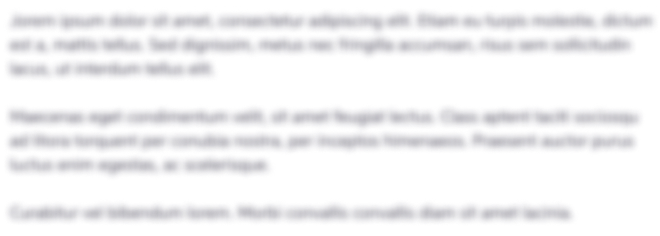
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started