Question
Computer Simulations Computers are often used to simulate complex scenarios such as weather patterns, nuclear reactions, climate change and potential spread of infectious diseases. Many
Computer Simulations
Computers are often used to simulate complex scenarios such as weather patterns, nuclear reactions, climate change and potential spread of infectious diseases. Many of the simulations have the same basic design. Events are scheduled to occur at a particular time in the future. As the events occur they create additional events. This sequence continues until an ending condition of the simulation.
A list of future events is similar to a to do list you might create for today. The eventual sequence of tasks is not known at the start of the simulation. You may start the day with a number of tasks to complete at particular times such as go to class, meet a friend for lunch or go to work. Throughout the day, you might add unexpected tasks such as picking up your younger sister from ballet practice. This event might create an additional task such as take your sister to dinner.
Our GVSU Market Place Simulation
You will create an application to simulate customers arriving and departing the GVSU Market Place on a busy football Saturday. It will be used by the store manager to predict how long customers will be waiting in line and how many cashiers to schedule. Customers enter the store, browse for items, and then some customers get in line with their purchases.
After shopping, customers get in a single line and wait for their turn at one of the cashiers. Customers eventually move to the next available cashier. After the customer pays for her purchases, she leaves and the next customer in line (if any) moves to the cashier. This continues all day long until closing time. Customers in line at closing are served but no new customers can get in line. The store opens at 10:00 am and closes at 6:00 pm.
Simulation Parameters
The manager can manipulate three parameters to influence the simulation.
The average customer arrival time predicts how often a customer gets in line. This can be based on store history. If customers arrive every 2 minutes on average, the simulation will generate random customer arrival times above and below 2 minutes.
The average service time is how long a cashier takes to serve one customer. Service times vary based on the number of purchases and the speed of the casher.
The number of cashiers on duty determine how many customers can be served simultaneously. The manager wants to schedule the fewest number of cashiers without causing long lines.
Simulation Results
The manager will see several results: 1) the number of customers served during the day, 2) the average customer wait time in line, 3) the length and time of the longest line and 4) time of the last customer departure. Wait time for a customer includes the amount of time in line but NOT the service time at the cashier. Simulations generate events randomly resulting in slightly different results each time.
Store Events
Event times are maintained as a double representing the number of minutes into the day starting at midnight. For example, thirty minutes into the simulation is 12:30 am. Four hundred minutes into the simulation is 6:40 am. Another example, 5:36 pm is 1,056 minutes into the simulation.
An arrival event generates a new Customer object and places it in line. If no customers are currently in line, the new customer proceeds directly to an available cashier (if any).
A departure event relates to a particular cashier. Customers pay the cashier and leave the store. This frees up the cashier for the next customer in line (if any).
Customers
Customers are represented with a simple class that maintains the time the customer gets in line. This time is used to determine how long the customer waited in line.
Cashiers
Cashiers are represented as a single array of Customer. If no customer is currently with the cashier, the array element contains null. Otherwise, the array element contains a Customer reference. For example, the following array shows four cashiers with customers at location 0 and 2 but not at 1 or 3.
{Customer, null, Customer, null}
Future Event Queue
A list of future events is maintained in a Java data structure called a PriorityQueue. It is a special version of an ArrayList that maintains objects in sorted order. Regardless of the order that future events are added, they are removed in the order they should occur. Using PriorityQueues is identical to using ArrayLists with the exception of the method used to remove the next item. Review the following sample code:
GVevent evt;
PriorityQueue
futureEvents = new PriorityQueue
futureEvents.add(evt);
evt = futureEvents.poll(); // remove item from front of list
Step 1: Create a New BlueJ Project
Step 2: Use existing class GVevent
Rather than writing your own GVevent class, we are providing the completed class for you. Create a new class in BlueJ called GVevent and delete all of the provided code. Copy and paste the provided code from (GVevent.java) into the newly created class. It should compile with no errors. Do not make any changes to this code. However, you should read the internal documentation to understand how it will be used in this project.
Step 3: Use existing class GVrandom
Rather than writing your own GVrandom class, we are providing the completed class for you. Create a new class in BlueJ called GVrandom and delete all of the provided code. Copy and paste the provided code from (GVrandom.java) into the newly created class. It should compile with no errors. Do not make any changes to this code. However, you should read the internal documentation to understand how it works.
Step 4: Create a class called Customer (4 pts)
Implement a Customer class to maintain the time the customer arrived in line (double).
public Customer (double time) initialize the instance variable.
public void setArrivalTime (double t) set the instance variable.
public double getArrivalTime () return the arrival time
include a brief main method to test this class
Step 5: Create a GVarrival class (4 pts)
Refer to zyBook 13.1 13.3 for basic information about inheritance. Implement a class called GVarrival that extends GVevent.
public GVarrival (MarketPlace store, double time) invoke the base class constructor to set the MarketPlace object and the event time.
super(store, time);
public void process () use the MarketPlace object in the base class to invoke customerGetsInLine(). One line of code but will not compile until after Step 7.
Step 6: Create a GVdeparture class (4 pts)
Implement a class called GVdeparture that extends GVevent.
public GVdeparture (MarketPlace store, double time, int id) invoke the base class constructor to set the MarketPlace object, the event time, and the cashier ID.
super(store, time, id);
public void process () use the MarketPlace object in the base class to invoke customerPays().One line of code but will not compile until after Step 7.
Step 7: Start the MarketPlace class (8 pts)
This is a complex class that relies heavily on the other classes such as Customer, GVarrival, GVdeparture and GVrandom to simulate the arrival and departure of customers throughout the day.
import static org.junit.Assert.*; import org.junit.*; /**************************************************** * MAKE NO CHANGES TO THIS CODE * This class is used for automatically testing Part 1 * of the MarketPlace simulation. ***************************************************/ public class JunitTest1{ private MarketPlace theStore; private Customer cust; private GVarrival arrive; private GVdeparture depart; private double TOLERANCE = 0.1; /****************************************************** * Instantiate a Market Place object. * * Called before every test case method. *****************************************************/ @Before public void setUp(){ theStore = new MarketPlace(); cust = new Customer(30); arrive = new GVarrival(theStore, 45.7); depart = new GVdeparture(theStore, 45.7, 99); } /****************************************************** * Test Customer methods *****************************************************/ @Test public void testCustomer(){ Assert.assertEquals("Customer(): arrival time should be 30.0", 30, cust.getArrivalTime(), TOLERANCE); cust.setArrivalTime(53.2); Assert.assertEquals("Customer(): arrival time should be 53.2", 53.2, cust.getArrivalTime(), TOLERANCE); } /****************************************************** * Test GVarrival methods *****************************************************/ @Test public void testArrival(){ Assert.assertEquals("GVarrival(): time should be 45.7", 45.7, arrive.getTime(), TOLERANCE); } /****************************************************** * Test GVdeparture methods *****************************************************/ @Test public void testDeparture(){ Assert.assertEquals("GVdeparture(): time should be 45.7", 45.7, depart.getTime(), TOLERANCE); Assert.assertEquals("GVdeparture(): cashier ID should be 99", 99, depart.getCashier()); } /****************************************************** * Test initial values of the constructor *****************************************************/ @Test public void testConstructor(){ // confirm the default number of tellers Assert.assertEquals("MarketPlace(): should start with 3 tellers ", 3, theStore.getNumCashiers()); // confirm the default service time Assert.assertEquals("MarketPlace(): should start with 6.6 service time ", 6.6, theStore.getServiceTime(), TOLERANCE); // confirm the default arrival time Assert.assertEquals("MarketPlace(): should start with 2.5 arrival time ", 2.5, theStore.getArrivalTime(), TOLERANCE); } /****************************************************** * Test set parameters *****************************************************/ @Test public void testSetParameters(){ theStore.setParameters(6, 14.5, 4.8, false); // confirm cashiers changed Assert.assertEquals("Number of cashiers should be changed", 6, theStore.getNumCashiers()); // confirm arrival time Assert.assertEquals("Arriva time should be changed", 4.8, theStore.getArrivalTime(), TOLERANCE); // confirm cashiers Assert.assertEquals("Service time should be changed", 14.5, theStore.getServiceTime(), TOLERANCE); } /****************************************************** * Confirm public methods exist *****************************************************/ @Test public void testPublicMethods(){ theStore.getReport(); theStore.getArrivalTime(); theStore.getServiceTime(); theStore.getNumCustomersServed(); theStore.getLongestLineLength(); theStore.getAverageWaitTime(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
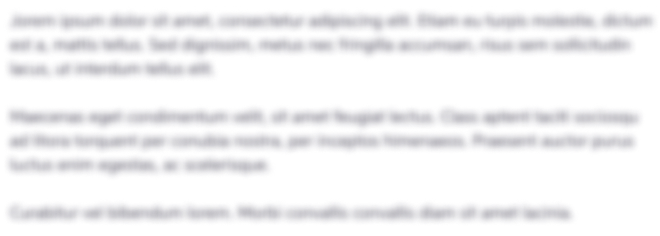
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started