Question
Consider an interface public interface NumberFormatter { String format (int n); } Provide four additional classes that implement this interface. COP3337 Assignment 4 A DefaultFormatter
Consider an interface public interface NumberFormatter { String format (int n); } Provide four additional classes that implement this interface. COP3337 Assignment 4 A DefaultFormatter formats an integer in the usual way. A DecimalSeparatorFormatter formats an integer with decimal separators; for example, one million as 1,000,000. An AccountingFormatter formats negative numbers with parenthesis; for example, -1 as (1). A BaseFormatter formats the number as base n, where n is any number between 2 and 36 that is provided in the constructor.
/** Class to test the format method from classes that implement the NumberFormatter interface */
public class NumberFormatterTester { public static void main(String[] args) { final int small = 5; final int neg = -10000; final int large = 1000000; final int maxBase = 36; NumberFormatter numFormat; numFormat = new DefaultFormatter(); System.out.println ("Default: " + numFormat.format(small) + " " + numFormat.format(neg) + " " + numFormat.format(large) + " " + numFormat.format(maxBase)); System.out.println ("Expected: 5 -10000 1000000 36"); numFormat = new DecimalSeparatorFormatter(); System.out.println ("Decimal: " + numFormat.format(small) + " " + numFormat.format(neg) + " " + numFormat.format(large) + " " + numFormat.format(maxBase)); System.out.println ("Expected: 5 -10,000 1,000,000 36"); numFormat = new AccountingFormatter(); System.out.println ("Accounting: " + numFormat.format(small) + " " + numFormat.format(neg) + " " + numFormat.format(large) + " " + numFormat.format(maxBase)); System.out.println ("Expected: 5 (10000) 1000000 36"); numFormat = new BaseFormatter(2); // Binary System System.out.println ("Base 2 : " + numFormat.format(small) + " " + numFormat.format(neg) + " " + numFormat.format(large) + " " + numFormat.format(maxBase)); System.out.println ("Expected: 101 -10011100010000 11110100001001000000 100100"); numFormat = new BaseFormatter(8); // Octal System System.out.println ("Base 8 : " + numFormat.format(small) + " " + numFormat.format(neg) + " " + numFormat.format(large) + " " + numFormat.format(maxBase)); System.out.println ("Expected: 5 -23420 3641100 44"); numFormat = new BaseFormatter(20); // Base 20 System.out.println ("Base 20 : " + numFormat.format(small) + " " + numFormat.format(neg) + " " + numFormat.format(large) + " " + numFormat.format(maxBase)); System.out.println ("Expected: 5 -1500 65000 1g"); numFormat = new BaseFormatter(36); // Base 36 System.out.println ("Base 36 : " + numFormat.format(small) + " " + numFormat.format(neg) + " " + numFormat.format(large) + " " + numFormat.format(maxBase)); System.out.println ("Expected: 5 -7ps lfls 10"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
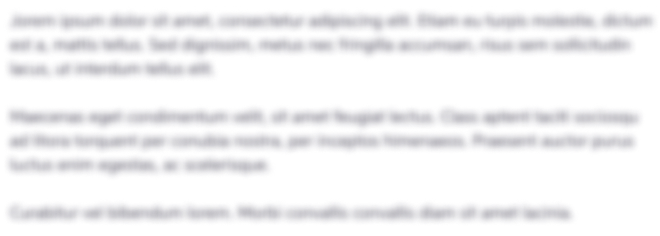
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started