Question
Consider following Binary Search Tree (BST) implementation taken from some online resource. Delete(treeNode *node, int data) deletes a node with value as data from the
Consider following Binary Search Tree(BST) implementation taken from some online resource. Delete(treeNode *node, int data) deletes a node with value as data from the tree.
Understand the behavior of the function Delete(treeNode *node, int data).
Write 3 unit tests for this function. Try to cover as much of code as possible. Please, use proper assertions to verify your expected results.
Discuss if any code is missed to test after writing all 3 tests.
Notes:
You dont need to use/run gcov to measure code coverage. Just simple discussion of coverage of the method using your unit tests will be sufficient.
You are provided few Binary Search Tree Operations at the beginning, use them to facilitate your testing. If they are not sufficient, just assume and use any Binary Search Tree operations you want to use to facilitate your test writing
typedef struct treeNode { int data; struct treeNode *left; struct treeNode *right; }treeNode; //BST operations treeNode* FindMin(treeNode *node) treeNode* FindMax(treeNode *node) treeNode * Insert(treeNode *node,int data) treeNode * Find(treeNode *node, int data) treeNode * Delete(treeNode *node, int data) { treeNode *temp; if(node==NULL) { printf("Element Not Found"); } else if(data < node->data) { node->left = Delete(node->left, data); } else if(data > node->data) { node->right = Delete(node->right, data); } else { if(node->right && node->left) { temp = FindMin(node->right); node -> data = temp->data; node -> right = Delete(node->right,temp->data); } else { temp = node; if(node->left == NULL) node = node->right; else if(node->right == NULL) node = node->left; free(temp); } } return node; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
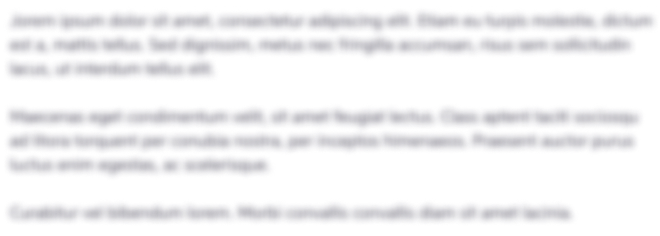
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started