Question
Consider simple infix expressions that consist of single-digit operands; +,-,*,%, and/; and parentheses. Assume that unary operators are illegal and that the expression contains no
Consider simple infix expressions that consist of single-digit operands; +,-,*,%, and/; and parentheses. Assume that unary operators are illegal and that the expression contains no embedded spaces.
Design and implement a class for the infix calculator. Use the algorithms given in this chapter to convert the infix expression to postfix form and to evalute the resulting postfix expression. Note that if the methodsevaluate and getPostfix method, then the exceptionIllegalStateException should be dirown by these methods.
class Calculator{
public Calculator(String exp)//initializes infix expression
public String toString() // returns infix expression
private boolean convertPostfix() //creates postfix expression
//returns true if successful
//The following methods should throw IllegalStateException if // they are called before convertPostfix
//returns the resulting postfix expression
public String getPostfix() throws IllegalStateException
//evalutes the expression
public int evaluate () throws IllegalStateException //end Calculator
As the problem describes you should create a class Calculator. Your class Calculator should PRINT NOTHING. Be sure to provide a Unit Test Driver.
Below I have created the Calculator class I need help with creating a good Unit Test Driver. Also there is a bonus that I would like to incorporate as well.
BONUS: To receive a 20% bonus make sure your implementation will discard spaces and then recognize multiple adjacent (no spaces between) digits as integers. Processing should follow from there.
import java.util.Stack;
public class Calculator { private String infix; private String postfix; public Calculator(String exp) { infix = exp; }
public int evaluate() throws IllegalStateException { if(postfix == null) throw new IllegalStateException("First Convert Infix Expression to Postfix"); int n,r=0; n=postfix.length() ; Stack
public boolean convertPostfix() { String postfixString = " "; Stack
for (int index = 0; index < infix.length(); ++index) { char chValue = infix.charAt(index); if (chValue == '(') { stack.push('('); } else if (chValue == ')') { Character oper = stack.peek(); while (!(oper.equals('(')) && !(stack.isEmpty())) { postfixString += oper.charValue(); stack.pop(); oper = stack.peek(); } stack.pop(); } else if (chValue == '+' || chValue == '-') { //Stack is empty if (stack.isEmpty()) { stack.push(chValue); //current Stack is not empty } else { Character oper = stack.peek(); while (!(stack.isEmpty() || oper.equals(new Character('(')) || oper.equals(new Character(')')))) { stack.pop(); postfixString += oper.charValue(); } stack.push(chValue); } } else if (chValue == '*' || chValue == '/') { if (stack.isEmpty()) { stack.push(chValue); } else { Character oper = stack.peek(); while (!oper.equals(new Character('+')) && !oper.equals(new Character('-')) && !stack.isEmpty()) { stack.pop(); postfixString += oper.charValue(); } stack.push(chValue); } } else { postfixString += chValue; } } while (!stack.isEmpty()) { Character oper = stack.peek(); if (!oper.equals(new Character('('))) { stack.pop(); postfixString += oper.charValue(); } } postfix = postfixString; return true; }
private int prec(char x) { if (x == '+' || x == '-') return 1; if (x == '*' || x == '/' || x == '%') return 2; return 0; } public String getPostfix() throws IllegalStateException { if(postfix == null) throw new IllegalStateException("First Convert Infix Expression to Postfix"); return new String(postfix); }
@Override public String toString() { return new String(infix); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
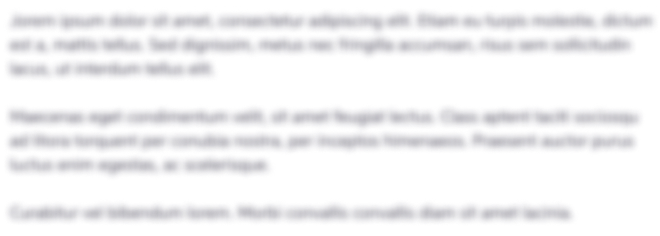
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started