Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Consider that a Stack class has been implemented containing the push ( element ) , pop ( ) , peek ( ) and isEmpty (
Consider that a Stack class has been implemented containing the pushelement pop peek and isEmpty functions.
Complete the function conditionalreverse which will take an object of Stack class as an input that contains some integer values. The function returns a new stack which will contain the values in reverse order from the given stack except the consecutive ones You cannot use any other data structure except Stack.
Note: The Stack class implements a singly linked listbased Stack hence overflow is not possible. The pop and peek functions return None in case of the underflow.
In the following example, consider the rightmost element to be the topmost element of the stack.
Sample Input Stack
Rightmost is the top
Output Reversed Stack Rightmost is the top
Explanation
Stack:
Top
New Stack:
Top
Consecutive and are not present in the output reversed stack
Driver code:
def conditionalreversestack:
#To Do
printTest
stStack
stpush
stpush
stpush
stpush
stpush
stpush
stpush
printStack:
printstackst
print
reversedstackconditionalreversest
printConditional Reversed Stack:
printstackreversedstack # This stack contains in this order whereas top element should be
print
Linked List based Stack is implemented in the following cell.
class Node:
def initselfelemNone,nextNone:
self.elem elem
self.next next
class Stack:
def initself:
self.top None
def pushselfelem:
nn Nodeelemself.top
self.top nn
def popself:
if self.top None:
#printStack Underflow'
return None
e self.top
self.top self.top.next
enext None
return eelem
def peekself:
if self.top None:
#printStack Underflow'
return None
return self.top.elem
def isEmptyself:
return self.top None
#You can run this driver code cell to understand the methods of Stack class
st Stack
stpush
stpush
stpush
stpush
stpush
printPeeked Element: stpeek
printPopped Element: stpop
printPopped Element: stpop
printPopped Element: stpop
printPeeked Element: stpeek
printPopped Element: stpop
printPopped Element: stpop
printPeeked Element: stpeek
printPopped Element: stpop
printstisEmpty
You can print your stack using this code segment
def printstackst:
if stisEmpty:
return
p stpop
printpend
if p:
print
else:
print
#print
printstackst
stpushp
# st Stack
# stpush
# stpush
# stpush
# stpush
# stpush
# printstackst
# print
Step by Step Solution
There are 3 Steps involved in it
Step: 1
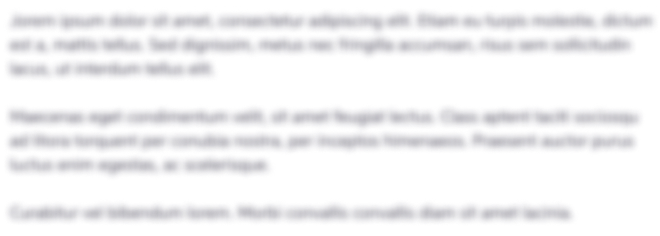
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started