Question
Consider the class Course given in the appendix . Rewrite the addStudent function in the Course.cpp to throw a runtime_error if the number of students
Consider the class Course given in the appendix . Rewrite the addStudent function in the Course.cpp to throw a runtime_error if the number of students exceeds the capacity
StackOfIntegers.h
#ifndef COURSE_H
#define COURSE_H
#include
using namespace std;
class Course
{
public:
Course(const string& courseName, int capacity);
~Course();
string getCourseName() const;
void addStudent(const string& name);
void dropStudent(const string& name);
string* getStudents() const;
int getNumberOfStudents() const;
private:
string courseName;
string* students;
int numberOfStudents;
int capacity;
};
#endif.
StackOfIntegers.cpp
#include
#include "Course.h"
using namespace std;
Course::Course(const string& courseName, int capacity)
{
numberOfStudents = 0;
this->courseName = courseName;
this->capacity = capacity;
students = new string[capacity];
}
Course::~Course()
{
delete [] students;
}
string Course::getCourseName() const
{
return courseName;
}
void Course::addStudent(const string& name)
{
students[numberOfStudents] = name;
numberOfStudents++;
}
void Course::dropStudent(const string& name)
{
// Left as an exercise
}
string* Course::getStudents() const
{
return students;
}
int Course::getNumberOfStudents() const
{
return numberOfStudents;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
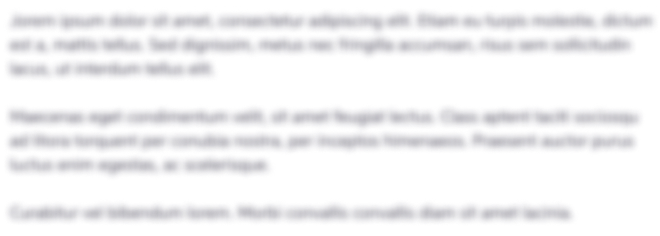
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started