Question
Consider the definition of a LinkedIntList class and ListNode class below. Write a method for this class, called everyOther(). This method takes no arguments, but
Consider the definition of a LinkedIntList class and ListNode class below. Write a method for this class, called everyOther(). This method takes no arguments, but it eliminates every other node in the linked list. For example, if a LinkedList list contains [1, 2, 3, 4, 5], after a the call: list.everyOther(), list contains [1, 3, 5]. If list contains [1, 2, 3, 4], after the call it will contain [1, 3]. If the list contains 0 or 1 elements, the list is unchanged. You are not allowed to assume any other methods are defined on the LinkedIntList class other than the ones defined on the below.
// Class LinkedIntList can be used to store a list of integers.
import java.util.*;
public class LinkedIntList {
// first value in the list
private ListNode front;
// size of list
private int size;
// post: constructs an empty list
public LinkedIntList() {
front = null;
}
// post: returns the current number of elements in the list
public int size() {
return size;
}
// pre : 0 <= index < size()
// post: returns the integer at the given index in the list
public int get(int index) {
return nodeAt(index).data;
}
// post: creates a comma-separated, bracketed version of the list
public String toString() {
// if the list is empty
if (front == null) {
return "[]";
}
// otherwise
String result = "[" + front.data;
ListNode current = front.next;
while (current != null) {
result += ", " + current.data;
current = current.next;
}
result += "]";
return result;
}
// post : returns the position of the first occurrence of the given
// value (-1 if not found)
public int indexOf(int value) {
int index = 0;
ListNode current = front;
while (current != null) {
if (current.data == value) {
return index;
}
index++;
current = current.next;
}
return -1;
}
// post: appends the given value to the end of the list
public void add(int value) {
size++;
// if this is the only node in this list
if (front == null) {
front = new ListNode(value);
return;
}
// otherwise, we need to put the new node at the end of the list
ListNode current = front;
while (current.next != null) {
current = current.next;
}
current.next = new ListNode(value);
}
// pre: 0 <= index <= size()
// post: inserts the given value at the given index
public void add(int index, int value) {
size++;
if (index == 0) {
// put the new node at the front
front = new ListNode(value, front);
} else {
// get the node just this node will link to
ListNode current = nodeAt(index - 1);
current.next = new ListNode(value, current.next);
}
}
// pre : 0 <= index < size()
// post: removes value at the given index
public void remove(int index) {
size--;
// if we are removing the front ndoe
if (index == 0) {
front = front.next;
} else {
// get the node that the node we are removing is linked to
ListNode current = nodeAt(index - 1);
current.next = current.next.next;
}
}
// pre : 0 <= i < size()
// post: returns a reference to the node at the given index
private ListNode nodeAt(int index) {
ListNode current = front;
for (int i = 0; i < index; i++) {
current = current.next;
}
return current;
}
public int deleteBack() {
// Empty list
int nodeData = 0;
if (front == null) {
// throw new NoSuchElementException();
}
// List length 1
else if (front.next == null) {
nodeData = front.data;
remove(0);
}
else {
ListNode current = nodeAt(size - 2);
nodeData = current.next.data;
remove(size - 1);
}
return nodeData;
}
public int lastIndexOf(int value) {
int index = -1;
int position = 0;
ListNode current = front;
if (front != null) {
while (position < size) {
if (current.data == value)
index = position;
position++;
current = current.next;
}
}
return index;
}
}
--------------------------------------------------------------
// ListNode is a class for storing a single node of a linked
// list. This node class is for a list of integer values.
public class ListNode {
public int data; // data stored in this node
public ListNode next; // link to next node in the list
// Post: constructs a node with data 0 and null link
public ListNode() {
this(0, null);
}
// Parameter: data, the integer value to be stored
// Post: constructs a node with given data and null link
public ListNode(int data) {
this(data, null);
}
// Parameters: data, the integer value to be stored,
// next, the link to set next to
// Post: constructs a node with given data and given link
public ListNode(int data, ListNode next) {
this.data = data;
this.next = next;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
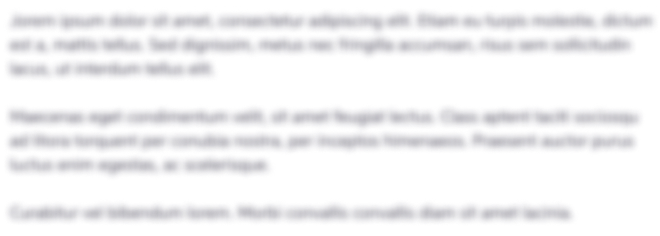
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started