Question
Consider the following C++ program. At the top, you can see the interface for the Temperature class that lists the public methods and the private
Consider the following C++ program. At the top, you can see the interface for the Temperature class that lists the public methods and the private attributes. Next, we have the implementation of all of the class methods. Finally, the main program that prompts the user for a start and end temperature and prints a small Fahrenheit to Celsius temperature conversion table.
#includeusing namespace std; // Interface for Temperature class class Temperature { public: Temperature(); Temperature(const Temperature & Temp); ~Temperature(); double getCelsius() const; double getFahrenheit() const; void setCelsius(double Temp); void setFahrenheit(double Temp); private: static const double ABSOLUTE_ZERO = -273.15; double CelsiusTemperature; }; // Implementation of Temperature class Temperature::Temperature() { CelsiusTemperature = 0; } Temperature::Temperature(const Temperature & Temp) { CelsiusTemperature = Temp.CelsiusTemperature; } Temperature::~Temperature() { } double Temperature::getCelsius() const { return CelsiusTemperature; } double Temperature::getFahrenheit() const { return 9.0 * CelsiusTemperature / 5.0 + 32.0; } void Temperature::setCelsius(double Temp) { CelsiusTemperature = Temp; if (CelsiusTemperature < ABSOLUTE_ZERO) CelsiusTemperature = ABSOLUTE_ZERO; } void Temperature::setFahrenheit(double Temp) { CelsiusTemperature = (Temp - 32.0) * 5.0 / 9.0 ; if (CelsiusTemperature < ABSOLUTE_ZERO) CelsiusTemperature = ABSOLUTE_ZERO; } // Program to test Temperature class int main() { double Start, End; cout << "Enter start temperature: "; cin >> Start; cout << "Enter end temperature: "; cin >> End; cout.precision(3); for (double F = Start; F <= End; F++) { Temperature Temp; Temp.setFahrenheit(F); cout << F << "F = " << Temp.getCelsius() << "C "; } return 0; }
Step 1: Copy this program into your C++ program editor, and compile it. Hopefully you will not get any error messages. Run the program and type in "75 85" to see what is printed out.
Step 2: In general, it is helpful to have a "print" method in every class so users can more easily output the values stored in the object. To do this we must make changes to both the interface and implementation of the class. Your first task is to add a new method prototype to the class interface. Add "void print();" into the public section of the Temperature class
Step 3: Your next task is to implement the print method. Remember, to tell the compiler this is part of the Temperature class, your first line should be "void Temperature::print()". Write your method so the Fahrenheit temperature is printed first followed by the Celsius temperature to match the output of the current main program (eg. "80F = 26.7C"). Remember, when you are inside the implementation of a Temperature method, you can access any private variable. You can also call any method without adding an object name before the method call.
Step 4: Compile and run your program to verify that the print method is syntactically correct. Now it is time to call the print method in your main program instead of using cout statements. Edit your code to make these changes, and recompile the program. When you run it, the output should be the same as before, but the main program should be smaller and slightly cleaner looking.
Step 5: Since we have a way to output temperatures, lets add a method to input temperatures. Edit your program and add "void read();" to the public class interface.
Step 6: Now add the implementation of the "read" method. Your code should use cin to read a number followed by a single character. If the user enters "30 c" or 30 C", you should store this as 30 Celsius. If the user enters "80 f" or "80 F", you should store this as 80 Fahrenheit. If the user enters any other character besides c,C,f,F you should "fail quietly" and simply ignore their input.
Step 7: Compile and run your program to verify that the read method is syntactically correct. Now it is time to modify the main program to use your new read method. First, go to the main program and declare two Temperature objects called "Start" and "End" (and remove the double variables with these names). Next, remove the cin statements and use the "read" method to initialize your two temperature values.
Step 8: If you try to compile at this point, you will get syntax errors. To fix your program, you must modify the first line of the for loop. Think about what we are trying to do. We want the program to loop over a range of Fahrenheit temperatures. How can you do this using the Start and End objects, and the methods in the Temperature class?
Step 9: Once you have your code compiling, run the program and test it by typing in "75 F 85 F" to verify that you are correctly reading temperature values. You should get the same output as your initial program. Complete your testing by trying a variety of Celsius values.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
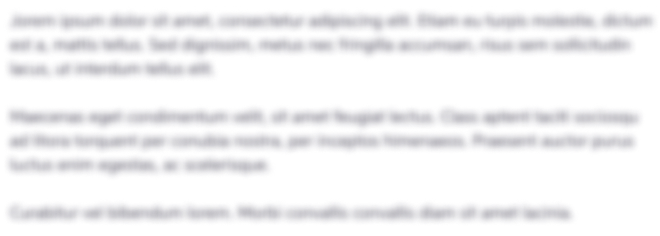
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started