Question
Consider the following two files: 1) courses.txt contains the following 6 records (but you are not supposed to know the exact number of the records):
Consider the following two files:
1) "courses.txt" contains the following 6 records (but you are not supposed to know the exact number of the records):
CSC114, Computer Science 1, 4
CIS130, Computer Organization, 3
CSC164, Computer Science 2, 4
CIS211, Data Structures, 4
CSC264, Applied Computer Capstone, 4
Each record contains 3 fields seperated by a comma and the three fields follow:
i. Course number: a string ( or 6 characters)
ii. Course name: a string
iii. Credits: integer
2) "updates.txt" contains the following records"
A, CSC236, Computer Organization and Assembly Language, 4
D, CIS130
d, CSC264
a, CSC175, Web Programming and Internet Technologies, 3
Each record contain either two or four fields seperated by a comma. The first letter is the action code just like your project two except the code 'A' or 'D' could be either upper or lower case. in case of adding a record, the fields that follow are the course number, course name and credits.
Assignment
1) Construct a class named Course which will represent the record in courses.txt and have it stored as a seperated file called Course.java
a) one constructor that accepts course number, course name, and credits
b) getter and setter
c) toString()
2) Name your main program "Program1" and have the main method call a method named "step 1" that contains the following:
a) Reserve an array, called courseArray, of size 10 for Course objects
b) Read "courses.txt" file and have all records stored in courseArray
c) Write your full name and a blank line to an output file called "ex1out1.txt" "Please make up a name"
and then followed by the contents of courseArray in the descending order
d) Add an ArrayList of Course class and call it courseArrayList
e) in addition to reading "courses.txt" file into courseArray in step b, have the file read into the courseArrayList as well
f) Append the contents of "courseArrayList" in ascending order to "ex1out1.txt" after the output of "courseArray
g) print the two java files
3) For the following two steps, you are going to implement the Delete Byte approach that you did in project 2
a) Construct a class named CourseID (CourseD.java) that inherits Course and contain
1. deleteByte: either True or False;
2. Constructor that accepts "Course Number", "Course Name", "Credits" and "Delete Byte";
3. Getter and setter for Delete Byte;
4. toString();
b) Construct a class named ReadCourseID. This class will open and read courses.txt file and store all records into an ArrayList of CourseD
c) Comment out "step 1()" so that this method will be executed
d) Add a method named "step 2" in the main program which does the following:
1) Make an instance of "ReadCourseD" using a name of your own choosing
2) Arrange to have the records of courses.txt read into an ArrayList of CourseD (use a meaningful name of your own choosing)
3) Print the contents of the ArrayList of CourseD to an output file named "ex1out2.txt"
e) Run the program
4) In this step, you are going to complete step 2 above by ading the following functions:
a) Implement "add" and "delete" methods;
b) Read "changes.txt" file and call the appropriate methods to update ArrayList of CourseD. When adding a new course, we assume the course is indeed a new one.
c) Send the contents of the updated ArrayList of ItemD to ex1out2.txt
Step by Step Solution
There are 3 Steps involved in it
Step: 1
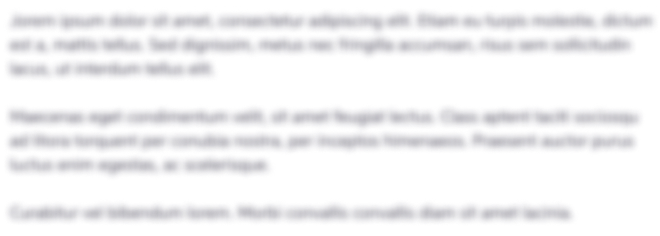
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started