Question
Constraints: Display any numerical output with 2 digits after the decimal point DO NOT use break and continue DO NOT use exit() to exit a
Constraints: Display any numerical output with 2 digits after the decimal point
DO NOT use break and continue
DO NOT use exit() to exit a program
All attributes must be defined as private.
NOTE: Recall that only the latest constructor definition is used. So, if you have more than one constructor, the constructor defined last will take precedence.
Assignment: Write a program that involves solving problems using OOP principles of inheritance.
Process:
1. Write code to define a class that represents a Person.
The attributes should be the first name, last name, and age in years.
Define a constructor with parameters that sets appropriate initial values for the attributes based on the given parameters.
Define the SETTER and GETTER methods that provide access to the private member variables.
Define a __str__ method that displays the values of the attributes.
Define a member method that displays the values of the attributes.
2. Write code to define a class that represents a Doctor inherited from the class Person.
This class will have an attribute to store a doctors specialty (Anesthesiologist, Cardiologist, etc.).
Define a constructor with parameters that sets appropriate initial values for the attributes based on the given parameters.
Define the SETTER and GETTER methods that provide access to the private member variables.
Define a __str__ method that prints the values of the attributes.
3. Write code to define a class that represents a Patient inherited from the class Person.
This class will have an attribute to store a patients identification number and an account number (account number may include letters and special symbols).
Define a constructor with parameters that sets appropriate initial values for the attributes based on the given parameters.
Define the SETTER and GETTER methods that provide access to the private member variables.
Define a __str__ method that displays the values of the attributes.
4. Show the usage of your classes by defining various objects that uses the constructors and your methods. At a minimum, we will need:
2 objects from the Person class
2 objects the Doctor class.
2 objects the Patient class.
Allow for input for the attributes and show the results after exercising your methods.
Make sure to show usage of your GETTER and SETTER methods.
Test run:
Test 1: A default person Person Person: Goof Ball Age: 25 Using the display member method First Name: Goof Last Name: Ball Age: 25 Enter the first name of a person: alan Enter the last name of a person: turing Enter age: 42 Test 2: Updated data for a Person Person: alan turing Age: 42 Test 3: A typical Doctor Doctor: Person: Mary Jones Age: 33 specialty: Internist Update the Doctor's specialty: Cardiologist Test 4: A Doctor with updated specialty Doctor: Person: Mary Jones Age: 33 specialty: Cardiologist Test 5: A typical patient Patient: Person: Donald Duck Age: 55 Identification number: 1001 Account number: A12345 Update the identification number: 2001 Update the account number: B-12345 Test 6: A patient with updated data Patient: Person: Donald Duck Age: 55 Identification number: 2001 Account number: B-12345 Show the use of the getter method! - Last patient's First and last name: Donald Last Name: Duck
Step by Step Solution
There are 3 Steps involved in it
Step: 1
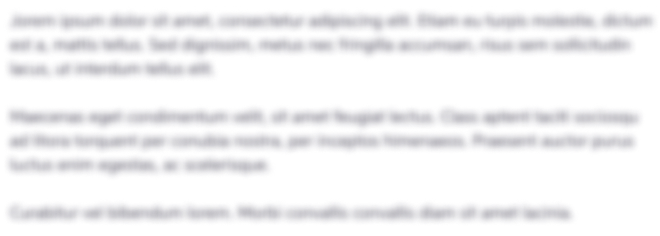
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started