Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Convert the following C++ code to C #include #include #include #include #include using namespace std; struct player { char row; int col; int carrier_hits; int
Convert the following C++ code to C #include #include #include #include #include using namespace std; struct player { char row; int col; int carrier_hits; int battleship_hits; int submarine_hits; int destroyer_hits; }; const int SIZE=10;//global constant to set the number of rows. enum vessel {Carrier='C', Battleship='B', Submarine='S', Destroyer='D', MISS='O', HIT='X', _=' '};// enumerated type that contains the ships and other variables such as O and X that will be used for the actual playing of the game. typedef vessel* vesselPtr; void personal_info(); //function that will display author's info. void instructions(int tries);//function that contains the instructions of the game. void initial_board(vesselPtr *Board);//initializes the board void print_board(vesselPtr *Board, bool reveal);//prints the board. void assignShips(vesselPtr *Board); bool check(vesselPtr *Board, player& hits); int main() { char choice;//will store the choice the user picks. int tries;// depending on which level tries is assigned a different value. int count; int length; string hitPos; string Colstr; vesselPtr *Board = new vesselPtr[SIZE]; player hits; bool sunk = false; srand(time(0));//randomization of ships and directions will be performed. for (int i = 0; i < SIZE; i++) { Board[i] = new vessel[SIZE]; } personal_info();//displays the author's information. //do while loop will keep looping as long as the difficulty level chosen in not one of the options given. do { cout << "Enter difficulty level (Easy, Normal, Hard) of game (E, N, or H): " << endl; cin >> choice; if (choice == 'E' || choice == 'e') { tries=30; } else if ( choice =='N' || choice =='n') { tries=25; } else if (choice=='H' || choice =='h') { tries=20; } }while((choice !='E')&&(choice !='e')&&(choice !='N')&&(choice !='n')&&(choice !='H')&&(choice !='h')); instructions(tries);//calls for instructions initial_board(Board);//calls for the initialization of the board. assignShips(Board);//once the second ship has been set this function calls for the actual positioning. print_board(Board, false);//printing of the board. hits.carrier_hits = 0; hits.battleship_hits = 0; hits.submarine_hits = 0; hits.destroyer_hits = 0; do { cout << "Enter position to fire torpedo #" << count++ << "(e.g., B7): "; cin >> hitPos; length = hitPos.length(); if ((length > 1) && (length < 4)) { hits.row = toupper (hitPos[0]); Colstr = hitPos.substr(1, hitPos.size() - 1); hits.col = atoi(Colstr.c_str()); if ((hits.row < 'A')||(hits.row > 'J')||(hits.col < 1)||(hits.col > 10)) { cout << "Invalid Position at " << hitPos << ". Try Again..." << endl; // count++; print_board(Board, false); continue; } sunk = check(Board, hits); print_board(Board, false); } }while ((count < tries) && (!sunk)); if (sunk) { cout << "Congratulations! You sunk both ships in " << count++ << " tries!" << endl; } else { cout << "Sorry, but you were not able to sink my ships! Tough break..." << endl; print_board(Board, true); } for (int i = 0; i < SIZE; i++) { delete [] Board[i]; } delete[] Board; return 0; } /* =================================================================================== Function: personal_info Parameters: none Return: void function returns no value Description:Display information about author. ================================================================================== */ void personal_info() { cout << " W e l c o m e t o B a t t l e s h i p " << endl; return; } /* =================================================================================== Function: instructions Parameters: int tries referring to the number of tries. Return: void function returns no value Description:Displays the instructions of the game along with how many tries the user get according to which level they chose. ================================================================================== */ void instructions(int tries) { cout << "-----------------------------------------------------------" << endl; cout << "This program will randomly choose two ships from your fleet" << endl; cout << "made up of the following vessels: Carrier, Battleship, Sub-" << endl; cout << "marine, and Destroyer. It will then randomly assign both of" << endl; cout << "the vessels to the board that are oriented either vertical-" << endl; cout << "ly or horizontally. As a player you will then have " << tries << " tries" << endl; cout << "to sink both of the computer's vessels!"<< endl; cout << "-----------------------------------------------------------" << endl; cout << "Initializing board..." << endl; cout << "Assigning vessels..." << endl; return; } /* =================================================================================== Function: initial_board Parameters: using the enum created vessel we refer to the Board and its coordinates. Return: void function returns no value Description:This function will initialize the board before printing it. ================================================================================== */ void initial_board(vesselPtr *Board) { for( int i=0; i< SIZE;i++)//for loop will check every spot that's less than the amount of ROWS { for (int j=0; j < SIZE; j++)//for loop will check every spot that's less than the amount of COLS. { Board[i][j]= _;//The board inside will just be blank spaces. } } } /* =================================================================================== Function: print_board Parameters: using the enum created vessel we refer to the Board and its coordinates. Return: void function returns no value. Description:This function will print the board. ================================================================================== */ void print_board(vesselPtr *Board, bool reveal) { cout << " 1 2 3 4 5 6 7 8 9 10 " << endl; cout << " +--------------------+" << endl; for (int i=0; i< SIZE; i++)//for loop sets the boundaries to less than ROW. { cout <(i+65) << "|";//this will output the letters and the right hand side border for (int j=0; j< SIZE; j++)//for loop sets the bottom boundaries to less than COLS. { if (((Board[i][j] == Destroyer)||(Board[i][j]== Submarine)||(Board[i][j] == Battleship)||(Board[i][j] == Carrier)) && (!reveal)) { cout << static_cast(_) << " "; } else { cout << static_cast(Board[i][j]) << " "; } } cout << "|" << endl;//the left hand side border } cout << " +--------------------+" << endl;//bottom border. return; } void assignShips(vesselPtr *Board) { const int NUM_SHIPS = 2; int i; int j; int vertical; int shipRowPos; int shipColPos; int ship[NUM_SHIPS]; bool valid; int counter = 0; vessel shipType; srand(time(0)); ship[counter] = (rand() % 4) + 2; counter++; do { ship[counter] = (rand()% 4) + 2; }while (ship[counter - 1] == ship [counter]); for (int index = 0; index < NUM_SHIPS; index++) { do{ valid = true; vertical = rand() % 2; shipRowPos = rand() % 10; shipColPos = rand() % 10; if (vertical) { if ((shipRowPos + ship[index]) <= SIZE) { for ( i = shipRowPos; i < (shipRowPos + ship[index]); i++ ) { if (Board[i][shipColPos] != _) { valid = false; } } } else { valid = false; } if (valid) { switch(ship[index]) { case 2: shipType = Destroyer; break; case 3: shipType = Submarine; break; case 4: shipType = Battleship; break; case 5: shipType = Carrier; break; } for (i = shipRowPos; i < (shipRowPos + ship[index]); i++) { Board[i][shipColPos] = shipType; } } } else { if ((shipColPos + ship[index]) <= SIZE) { for (j = shipColPos; j < (shipColPos + ship[index]); j++) { if (Board[shipRowPos][j] != _) { valid = false; } } } else { valid = false; } if (valid) { switch(ship[index]) { case 2: shipType = Destroyer; break; case 3: shipType = Submarine; break; case 4: shipType = Battleship; break; case 5: shipType = Carrier; break; } for (j = shipColPos; j < (shipColPos + ship[index]);j++) { Board[shipRowPos][j] = shipType; } } } }while (!valid); } return; } bool check(vesselPtr *Board, player& hits) { bool sunk = true; int row_number = hits.row - 'A'; switch(Board[row_number][hits.col - 1]) { case _: cout << "Miss..." << endl; Board[row_number][hits.col - 1] = MISS; break; case HIT: case MISS: cout << "Wasted shot.. already fired torpedo at this location!" << endl; break; case Destroyer: cout << "Hit... nice shot!" << endl; Board[row_number][hits.col - 1] = HIT; hits.destroyer_hits++; if (hits.destroyer_hits == 2) { cout << "Congratulations! You sank my Destroyer!!" << endl; } break; case Submarine: cout << "Hit... nice shot!" << endl; Board[row_number][hits.col - 1] = HIT; hits.submarine_hits++; if (hits.submarine_hits == 3) { cout << "Congratulations! You sank my Submarine!!" << endl; } break; case Battleship: cout << "Hit... nice shot!" << endl; Board[row_number][hits.col - 1] = HIT; hits.battleship_hits++; if (hits.battleship_hits == 4) { cout << "Congratulations! You sank my Battleship!!" << endl; } break; case Carrier: cout << "Hit... nice shot!" << endl; Board[row_number][hits.col - 1] = HIT; hits.carrier_hits++; if (hits.carrier_hits == 5) { cout << "Congratulations! You sank my Aircraft Carrier!!" << endl; } break; default: break; } for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { if ((Board[i][j] == Destroyer)||(Board[i][j] == Submarine)||(Board[i][j] == Battleship) || (Board[i][j] == Carrier)) { sunk = false; } } } return sunk; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
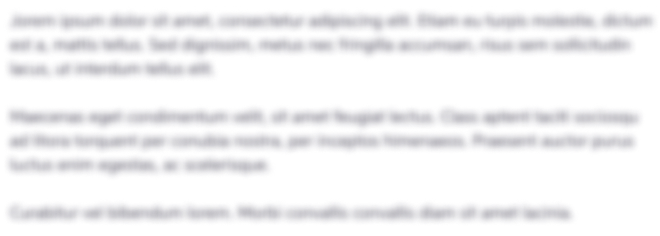
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started