Question
convert the following parser c program to c++ /* expr Parses strings in the language generated by the rule: -> {(+ | -) } */
convert the following parser c program to c++
/* expr
Parses strings in the language generated by the rule:
*/
void expr() {
printf("Enter
/* Parse the first term */
term();
/* As long as the next token is + or -, get
the next token and parse the next term */
while (nextToken == ADD_OP || nextToken == SUB_OP) {
lex();
term();
}
printf("Exit
} /* End of function expr */
/* term
Parses strings in the language generated by the rule:
*/
void term() {
printf("Enter
/* Parse the first factor */
factor();
/* As long as the next token is * or /, get the
next token and parse the next factor */
while (nextToken == MULT_OP || nextToken == DIV_OP) {
lex();
factor();
}
printf("Exit
} /* End of function term */
/* factor
Parses strings in the language generated by the rule:
*/ void factor() { printf("Enter /* Determine which RHS */ if (nextToken == IDENT || nextToken == INT_LIT) /* Get the next token */ lex(); /* If the RHS is ( left parenthesis, call expr, and check for the right parenthesis */ else { if (nextToken == LEFT_PAREN) { lex(); expr(); if (nextToken == RIGHT_PAREN) lex(); else error(); } /* End of if (nextToken == ... */ /* It was not an id, an integer literal, or a left parenthesis */ else error(); } /* End of else */ printf("Exit } /* End of function factor */ (sum + 47) /total should output Next token is: 25 Next lexeme is ( Enter Enter Enter Next token is: 11 Next lexeme is sum Enter Enter Enter Next token is: 21 Next lexeme is + Exit Exit Next token is: 10 Next lexeme is 47 Enter Enter Next token is: 26 Next lexeme is ) Exit Exit Exit Next token is: 24 Next lexeme is / Exit Next token is: 11 Next lexeme is total Enter Next token is: -1 Next lexeme is EOF Exit Exit Exit HERE is the lexer analyzer in c++ i used /* front.cpp - a lexical analyzer system for simple arithmetic expressions */ //This is front.cpp file #include #include #include using namespace std; /* Global declarations */ /* Variables */ int charClass; char lexeme[100]; char nextChar; int lexLen; int token; int nextToken; FILE *in_fp, *fopen(); /* Function declarations */ void addChar(); void getChar(); void getNonBlank(); int lex(); /* Character classes */ #define LETTER 0 #define DIGIT 1 #define UNKNOWN 99 /* Token codes */ #define INT_LIT 10 #define IDENT 11 #define ASSIGN_OP 20 #define ADD_OP 21 #define SUB_OP 22 #define MULT_OP 23 #define DIV_OP 24 #define LEFT_PAREN 25 #define RIGHT_PAREN 26 /******************************************************/ /* main driver */ int main() { /* Open the input data file and process its contents */ if ((in_fp = fopen("front.in", "r")) == NULL) //if (fopen(&in_fp,"front.in", "r")!=0) cout<<"ERROR - cannot open front.in"; else { getChar(); do { lex(); } while (nextToken != EOF); } int x; cin >> x; return 0; } /*****************************************************/ /* lookup - a function to lookup operators and parentheses and return the token */ int lookup(char ch) { switch (ch) { case '(': addChar(); nextToken = LEFT_PAREN; break; case ')': addChar(); nextToken = RIGHT_PAREN; break; case '+': addChar(); nextToken = ADD_OP; break; case '-': addChar(); nextToken = SUB_OP; break; case '*': addChar(); nextToken = MULT_OP; break; case '/': addChar(); nextToken = DIV_OP; break; default: addChar(); nextToken = EOF; break; } return nextToken; } /*****************************************************/ /* addChar - a function to add nextChar to lexeme */ void addChar() { if (lexLen <= 98) { lexeme[lexLen++] = nextChar; lexeme[lexLen] = 0; } else cout << "Error - lexeme is too long n"; } /*****************************************************/ /* getChar - a function to get the next character of input and determine its character class */ void getChar() { if ((nextChar = getc(in_fp)) != EOF) { if (isalpha(nextChar)) charClass = LETTER; else if (isdigit(nextChar)) charClass = DIGIT; else charClass = UNKNOWN; } else charClass = EOF; } /*****************************************************/ /* getNonBlank - a function to call getChar until it returns a non-whitespace character */ void getNonBlank() { while (isspace(nextChar)) getChar(); } /*****************************************************/ /* lex - a simple lexical analyzer for arithmetic expressions */ int lex() { lexLen = 0; getNonBlank(); switch (charClass) { /* Parse identifiers */ case LETTER: addChar(); getChar(); while (charClass == LETTER || charClass == DIGIT) { addChar(); getChar(); } nextToken = IDENT; break; /* Parse integer literals */ case DIGIT: addChar(); getChar(); while (charClass == DIGIT) { addChar(); getChar(); } nextToken = INT_LIT; break; /* Parentheses and operators */ case UNKNOWN: lookup(nextChar); getChar(); break; /* EOF */ case EOF: nextToken = EOF; lexeme[0] = 'E'; lexeme[1] = 'O'; lexeme[2] = 'F'; lexeme[3] = 0; break; } /* End of switch */ cout << " Next token is: " << nextToken << " , Next lexeme is: " < return nextToken; } /* End of function lex */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
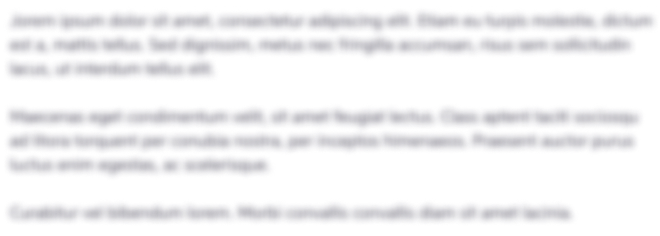
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started