Question
Convert the value returning functions below into void functions. Make the necessary changes to the function header, body and prototype: 1) #include using namespace std;
Convert the value returning functions below into void functions. Make the necessary changes to the function header, body and prototype:
1)
#include
using namespace std;
//declare the function
double timesTen(double num);
int main ()
{
//calling a function to get ten times the value of the argument.
double value = timesTen(10.50);
cout
return 0;
}
double timesTen(double num)
{
return num * 10;
}
Output:
2)
#include
using namespace std;
//declare the function
double findAverage(int num1, int num2, int num3, int num4);
int main ()
{
int num1,num2,num3,num4;
cout
cin >> num1;
cout
cin >> num2;
cout
cin >> num3;
cout
cin >> num4;
//calling a function to get average of four numbers.
double value = findAverage(num1,num2,num3,num4);
cout
return 0;
}
double findAverage(int num1, int num2, int num3, int num4)
{
return ((num1+num2+num3+num4)/4);
}
Output:
3)
#include
using namespace std;
//declare the function
double calDistance(int speed, int time);
int main ()
{
//calling a function to get distance.
double value = calDistance(30,45);
cout
return 0;
}
double calDistance(int speed, int time)
{
return speed * time;
}
Output:
4)
#include
using namespace std;
//declare the function
double fahrenheit(double centigrade);
int main ()
{
/* Calling it in a loop that displays a table of the Centigrade temperature 0 through 20
and their Fahrenheit equivalents, i.e. call the function inside the loop! */
for(int i=0; i
{
double value = fahrenheit(i);
cout Fahrenheit: "
}
return 0;
}
double fahrenheit(double centigrade)
{
return ((centigrade * 9.0) / 5.0 + 32);
}
Output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
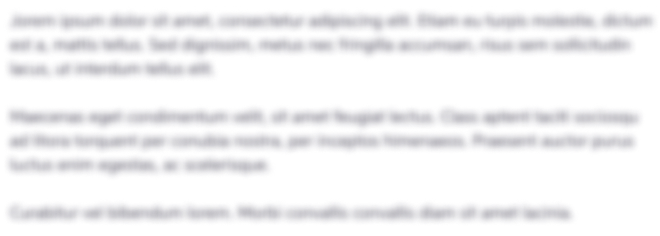
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started