Question
COP-4005 Assignment 2: Mini Battleship In this assignment you will develop a program where users can play the classic Battleship game. In our version of
COP-4005 Assignment 2: Mini Battleship
In this assignment you will develop a program where users can play the classic Battleship game. In our version of the game, the computer will display a 4x4 array of Labels in the screen, and the player must guess where are the two hidden ships. The player can click on each of the labels, which will change their background color depending on whether theres a ship or just water under it. The game finishes when the player has uncovered all the ships.
Requirements
You must create a Visual Studio solution and project for this assignment. When executed, the program will display 16 Labels in a 4x4 array and a Start button. The initial game will start when the Form is loaded, and the player can click the Start button to re-initialize the game.
There are 2 hidden ships under the 16 labels. Each ship is 2 labels long. One of them is always placed horizontally, and the other one is always vertical. When a game is started, the program will find random positions for the 2 ships, ensuring they dont overlap.
The player uncovers a position by clicking on its Label. If there are no ships in that position, the Label will change its color to blue (water). If there is a part of a ship in that position, the Label will change to a different color, depending on which of the ships is there.
This is an example of the game after the player has uncovered both ships:
In the example, blue Labels show empty positions, red Labels show the horizontal ship, and black Labels are for the vertical ship. Gray Labels are still uncovered.
You will need to maintain one or more arrays as attributes to keep track of the ships positions. Add event handlers to the 16 labels so that when a label is clicked, its color will change depending on whats at that position of the array. Remember that you can change a Labels color by assigning a Color object to its BackColor property.
Differently from our Tic-Tac-Toe example, I strongly recommend that you keep an array of Labels so that the program knows the position of each of the 16 Labels. Then, if the player clicks on a position, say [2,3], you can do something like
labels[2, 3].BackColor = ... Creating an array of labels follows the same principle as creating an array of ints. You can have a
declaration like this in your class:
private Label[,] labels;
Have in mind that you cannot assign the values in the class body, because the labels have not yet been initialized. You can do that in the Form Load event handler. For example:
private void Form1_Load(object sender, EventArgs e)
{
labels = new Label[ROWS, COLS] {
{ label00, label01, ... },
{ label10, label11, ... },
...
}; }
The game ends when the player has uncovered the 4 ship spots in the array. When this happens, you must show a message telling the user how many clicks were needed to win the game. When the game is started again, make sure you re-generate the board and cover all the Labels again.
You can use an array of booleans to keep track of the labels that the player has already uncovered. This will help you knowing when the user is clicking on an already uncovered label and not count that as a click.
In summary, the parts of this assignment are:
Generate a random board when the game starts. Place a horizontal and a vertical boat of size 2.
Every time the user clicks on a Label, change its color depending on whether theres water, ship1 or ship2 under it.
When the player has fully uncovered both ships, show a message with the number of clicks needed to win.
Tips
The initial generation of the board with random positions of the ships may be tricky. Heres an example pseudo-code to place the horizontal ship:
1. Generate a random column c between 0 and COLS-2 (i.e., never place it on
the rightmost column)
2. Generate a random row r between 0 and ROWS-1
3. If either position board[r,c] or position board[r,c+1] are occupied by a
ship, go back to Step 1. You can use a do { } while()
4. Otherwise, place the ship on board[r, c] and board[r, c+1]
Remember you can generate a random number between 0 and X-1 with Random rand = new Random();
rand.Next(X);
We used an array of strings in our Tic-Tac-Toe example, and represented user choices with X, O or . In this game, I recommend you use ints. For example, you can represent an empty space with a 0, ship1 with a 1, and ship2 with a 2.
Grading
The assignment is worth 20 points total. Out of these, the grading will be assigned as follows:
Completeness (60%) Does the program perform all the required functions?
Robustness (20%) Does the program tolerate faulty user input and corner cases without crashing?
Style (20%) Is the program well written? Are variable, method and control names meaningful? Is the program logic broken down into classes and methods?
Extra points for very well thought-of interfaces, creative use of .NET controls, advanced features, etc.
Submission
Once you have finished the program, create a zip file with the whole solution, assets and code, and submit it to Blackboard (Please don't submit generated binaries).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
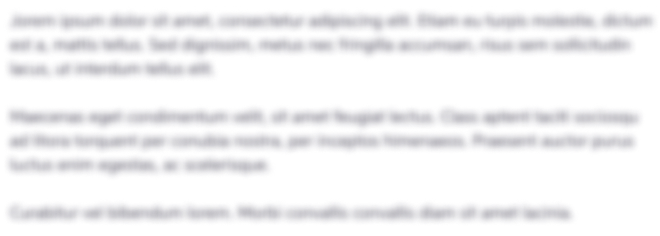
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started