Question
COP-4005 Assignment 3: Real Estate Inventory In this assignment you will develop a program where users can calculate their Real Estate investment value. Your program
COP-4005 Assignment 3: Real Estate Inventory
In this assignment you will develop a program where users can calculate their Real Estate investment value. Your program will allow users to add properties they own and then it will give them an approximate price based on an internal model.
Requirements
You must create a Visual Studio solution and project for this assignment. When executed, the program will allow users to add Real Estate properties using a form. In order to represent different types of properties, you should create different classes that use inheritance to reuse common functionality. This is the minimal set of classes you need to create:
1. A base abstract class called Property. A Property must have at least an address, a year built and a number of square feet.
2. A CommercialProperty class that inherits Property. This class must have a private enum field called type, with the possible values of INDUSTRIAL, OFFICE or RETAIL.
3. A ResidentialProperty class that inherits Property. Residential properties must have a private field of type List. Addition is another class with three possible subclasses: Pool, TennisCourt and Waterfront.
4. A SingleFamilyHome class that inherits ResidentialProperty. This class must have a boolean field called garage, which will be true if the house has an attached garage.
5. An Apartment class that inherits ResidentialProperty. The Apartment class must have a floor private field to indicate in which floor it is located.
The program will keep an internal List of Properties. Every time the user adds a new property, it will be appended to the list.
The program will also have an option to calculate the total cost of the properties in the system and another option to print a report of all the properties. The Property abstract class must define a CalculateCost method to be overridden by all subclasses. All classes must also override the ToString method, used when printing the report.
The CalculateCost method needs to have different implementations depending of the type of property. Here is an example, assuming you have defined a PRICE_PER_SQFT constant in your program:
All properties have a base cost of their number of square feet * PRICE_PER_SQFT
All properties subtract 0.1% of the base price per year since they were built
CommercialProperties add 30% of their base price if they are of industrial type, 35% if they are retail and 40% if they are offices ResidentialProperties add 10% per each Pool, 15% per each TennisCourt and 20% if they are Waterfront
SingleFamilyHomes add 5% if they have a garage
Apartments add 0.5% for each floor of height
Instead of doing all the calculations in one place, each class should be able to calculate its own cost by calling the parents class CalculateCost method and adding or subtracting an amount based on their own internal logic. For example, a SingleFamilyHome would get the cost from a ResidentialProperty and add an amount based on whether it has a garage. The ResidentialProperty would get the base cost from the Property class and add an amount based on its additions.
Have in mind that a class has all its attributes plus its parents attributes. For example, when users add an Apartment to the system, they need to enter the address, the year it was built, how many square feet it has, the additions and the floor number.
In summary, the parts of this assignment are:
1. Create a class hierarchy as explained above. Each class must have its own attributes, a CalculateCost method and a ToString method.
2. Create a form where the user has the choice to add a new Property of a given type. You can use different forms for each property type.
3. Add an option to list all the properties in the system. This will output one string for each property that was added, using its ToString method.
4. Add an option to calculate the total cost of all the properties in the system. This will call the CalculateCost of each of them and add the results up.
Feel free to modify the requirements for this assignment by adding additional property types, attributes, or different cost models. However, your class hierarchy should at least have the complexity of the classes described above.
Grading
The assignment is worth 20 points total. Out of these, the grading will be assigned as follows:
Completeness (60%) Does the program perform all the required functions?
Robustness (20%) Does the program tolerate faulty user input and corner cases without crashing?
Style (20%) Is the program well written? Are variable, method and control names meaningful? Is the program logic broken down into classes and methods?
Extra points for very well thought-of interfaces, creative use of .NET controls, advanced features, etc.
Submission
Once you have finished the program, create a zip file with the whole solution, assets and code, and submit it to Blackboard (Please don't submit generated binaries).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
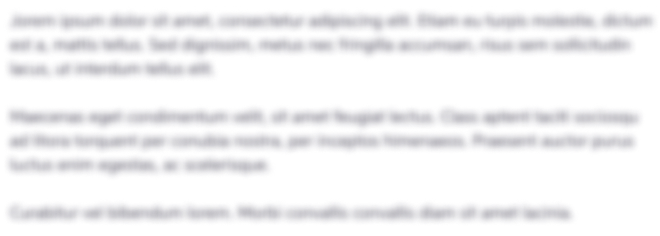
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started