Question
Copy and pasteable code: module alu( input [3:0] A,B, // ALU 4-bit Inputs input [3:0] ALU_Sel ,// ALU Selection output [3:0] ALU_Out , // ALU
Copy and pasteable code: module alu( input [3:0] A,B, // ALU 4-bit Inputs input [3:0] ALU_Sel ,// ALU Selection output [3:0] ALU_Out , // ALU 4-bit Output output CarryOut // Carry Out Flag ); reg [7:0] ALU_Result; wire [4:0] tmp; assign ALU_Out = ALU_Result; // ALU out assign tmp = {1b0 ,A} + {1b0 ,B}; assign CarryOut = tmp [4]; // Carryout flag always @(*) begin case(ALU_Sel) 4b0000: // Addition ALU_Result = A + B; 4b0001: // Subtraction ALU_Result = A - B; 4b0010: // Multiplication ALU_Result = A ** B; 4b0011: // Division ALU_Result = A/B; 4b0100: // Logical shift left ALU_Result = A>1; 4b0110: // Rotate left ALU_Result = {A[2:0] ,A[3]}; 4b0111: // Rotate right ALU_Result = {A[3],A[3:1]}; 4b1000: // Logical and ALU_Result = A && B; 4b1001: // Logical or ALU_Result = A || B; 4b1010: // Bitwise xor ALU_Result = A ^ B; 4b1011: // Logical nor ALU_Result = A || B ); 4 b1100 : // Logical nand ALU_Result = A && B ); 4 b1101 : // Bitwise xnor ALU_Result = A ^ B ); 4 b1110 : // Greater comparison ALU_Result = (A >B) ? 4 b0 : 4 b1 ; 4 b1111 : // Equal comparison ALU_Result = (A==B) ? 4b1 : 4b0; default: ALU_Result = A + B; endcase end endmodule
Code from lab 0:
module HA ( A, B, Sum , Carry ); input A; input B; output Sum; output Carry; assign Sum = A ^ B; // bitwise xor assign Carry = A & B; // bitwise and endmodule // half_adder
Testbench from lab 0: module tb_HA; reg r_A = 0; reg r_B = 0; wire w_Sum; wire w_Carry; HA HA_inst ( .A(r_A), .B(r_B), .Sum(w_Sum), .Carry(w_Carry) ); initial begin r_A = 1b0; r_B = 1b0; #10; r_A = 1b0; r_B = 1b1; #10; r_A = 1b1; r_B = 1b0; #10; r_A = 1b1; r_B = 1b1; #10; end endmodule // tb_HA
Flip Flop code:
module d-ff (Q, D, clock); input D, clock; output Q; reg Q; always @(posedge clock) begin Q
Flip Flop Testbench:
module test_dff(); reg D; reg clock; wire Q; //instantiate Design Under Test (DUT) dff dff_instant(.clock(clock),.D(D), .Q(Q)); initial begin // reset flop D = 1bx; clock = 0; end //clock generation always begin clock = 1b1; #20 // high for 20 * timescale clock = 1b0; #20 // low for 20 * timescale end // data generation always begin #10 D = 1b1; #30 D= 1b0; #10 D = 1b0; end //Debugging always begin #1 $display("d:%0h, q:%0h", D, Q); end endmodule
In this lab, you are given an arithmetic logic unit (ALU) design, which you are to test. The design has some bugs in it. You will design a test bench to test the functions of the ALU to find the bugs. You will also revise the sequential logic (D flip-flops) and create testbench for it. 1 ALU Overview An ALU performs basic arithmetic and logic operations. Examples of arithmetic operations are addition, subtraction, multiplication, and division. Examples of logic operations are comparisons of values ,such as NOT, AND, and OR. This ALU has 2 4-bit inputs A and B for operands, a 4-bit input ALU_Sel for selecting the operation, a 4-bit output ALU_Out, and a 1-bit output CarryOut. The datapath is just 4-bits wide to allow for easy coverage testing of the different operations. The operations of the ALU are specified in the table below. ALU-Sel 0000 0001 0010 0011 0100 0101 0110 0111 1000 1001 1010 1011 1100 1101 1110 1111 ALU Operation ALU-Out = A + B ALU-Out = A - B ALU_Out = A * B ALU_Out = A/B ALU_Out = A> 1 ALU_Out = A rotated left by 1 ALU_Out = A rotated right by 1 ALU_Out = A and B ALU_Out = A or B ALU_Out = A xor B ALU_Out = A nor B ALU_Out = A nand B ALU_Out = A xnor B ALU_Out = 1 if A>B else 0 ALU_Out = 1 if A=B else 0 Below you see the Verilog code for the ALU. Create a new Vivado project and copy this code to a file named alu.v. Code 1: 4-bit ALU. module alu input [3:0] A,B, // ALU 4-bit Inputs input [3:0] ALU_Sel,// ALU Selection output [3:0] ALU_Out, // ALU 4-bit Output output CarryOut // Carry Out Flag ); reg [7:0] ALU_Result; wire [4:0] tmp; assign ALU_Out = ALU_Result; // ALU out assign tmp = {1'50, A} + {1'50,B}; assign CarryOut = tmp [4]; // Carryout flag always @() begin case (ALU_Sel) 4'50000// Addition ALU_Result = A + B; 4'50001: // Subtraction ALU_Result = A - B; 4'60010: // Multiplication ALU_Result = A ** B; 4 'b0011: // Division ALU_Result = A/B; 4'50100: // Logical shift left ALU_Result = A>1; 4'b0110: // Rotate left ALU_Result = {A (2:0), A[3] }; 4'b0111: // Rotate right ALU_Result = {A[3] , A (3:1] }; 4' 51000: // Logical and ALU Result = A && B; 4'51001: // Logical or ALU Result = A || B; 4'b1010: // Bitwise xor ALU Result = A^ B; 4'b1011: // Logical nor ALU_Result = A || B); 4' 51100: // Logical nand ALU Result = A && B); 4' 51101: // Bitwise xnor ALU_Result = A A B); 4'b1110: // Greater comparison ALU Result = (A/B) ? 4'50 : 4'61; 4' 1111: // Equal comparison ALU_Result = (A==B) ? 4'bi : 4'50; default: ALU_Result = A + B; endcase end endmodule 2 Verification of Combinational Logic - ALU 1. Create the testbench, called tb_alu.v and add it to your Vivado project. You can use the test bench from lab 0 as a starting point. 2. Test the Addition (0000) operation. (a) Modify the testbench to provide stimulus that sets ALU_sel to the appropriate op code and sweeps through all possible combinations of inputs from A=0000, B=0000 to A=1111, B=1111. Include the simulation waveform in your report. (Note: only report several com- binations of A and B. Explain the different parts of these waveform in details such as, the inputs, the outputs, and the expected result.) (b) Is the operation implemented correctly? If not, indicate on the waveform where at least one of outputs was incorrect. Identify which line in alu.v contains the mistake, and propose a correction to this line in you report. Create a new file called alu_fixed.v with the mistakes being corrected. The ports declaration should be the same as alu.v and the name of the new module should be alu_fixed. Any changes in the port declaration will cause your module to fail during grading and you will lose all the points. 3. Repeat Step 2(a-b) for the following operations: multiplication, logical shift left, rotate right, logical AND, and greater comparison. Note: If the operation is not implemented correctly, you need to report the simulation waveform for at least one of the parts for which the output was incorrect. If the operation is implemented correctly, you need to report the simulation waveform for only 2-3 parts of the waveform and show why the outputs are correct. Note: Remember the fixed version of your ALU has to fix all the bugs. For example, the carryout is not implemented correctly in the given ALU. You need to think how to fix it to make sure that your operations are running correctly. Note: Your module name of the the fixed version of the alu has to be called (alu_fixed) with the same ports names as the provide module above. The file name has to be called (alu.fixed.v) 3 Verification of Sequential Logic - D flip-flop In lecture 1, we covered D flip-flop as an example for sequential logic circuits. Use the code from the lecture as a start and implement the following two versions of the D flip-flop: D flip-flop with synchronous set/reset. (filename: dff_SR.v) D flip-flop with asynchronous preset/clear. (filename: dff_PC.v) Design two testbenches to test for the correct operation of the two version of D flip-flop. Show the waveform from your test bench. Explain on your waveform how your flip-flop works correctly as expected. File names should be (filename: tb_dff_SR.v), and(filename: tb_dff_PC.v). The module declarations in your code for this question must be as follows: D flip-flop with synchronous set/reset: module dff_SR (clk, d, set, reset, q); D flip-flop with asynchronous preset/clear: module dff_PC (clk, d, preset, clear, q); Note: If the simulation of the new testbenches does not show up, you should do the following: File > Close Simulation Right-Click on the test bench you want to simulate > Set as Top Run the simulation againStep by Step Solution
There are 3 Steps involved in it
Step: 1
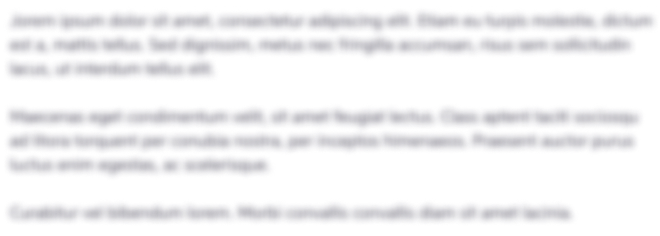
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started