Question
Core Java: OOPS Concept_ Problem Statement 3 Anjali is so glad as its her nephew Anirudh's first birthday. She wished to send a very special
Core Java: OOPS Concept_ Problem Statement 3
Anjali is so glad as its her nephew Anirudh's first birthday. She wished to send a very special birthday gift to her lilttle nephew from Canada to Singapore. She came to know the fact that a shipment can be dispatched by Agents and Companies on behalf of Customers. But she wanted to know the best means of shipping to send her birthday gift and needs your help on the same. Total shipment cost when shipped through Companies is the sum of base price, luxury tax and corporate tax, whereas when shipped through Agents it is the sum of base price and the agent's referal fee. Write a program to guide Anjali to choose the best means of shipment.
This problem uses the concept of Method Overriding through Hierarchical Inheritance. In this type of inheritance multiple classes inherits from a single class the two classes Agent and Company is inherited from Shipment class. If the two subclasses provides the specific implementation of the method that has been already provided by one of its parent class, it is known as method overriding.
Create a class named Shipment class with the following protected member variables / attributes
String name
String source
String destination
Double price
Include appropriate getters and setters.
Include 4 argument constructor with argument order Shipment(String name, String source, String destination, double price).
And this class includes the following method
No | Method Name | Description |
1 | double calculateShipmentAmount() | This is the super class method, which is overrided by the sub-classes. |
Use this format for output.
System.out.format("%-15s %-15s %-15s %s","Name","Source","Destination","Total Amount"). Create a AgentShipment class, which extends Shipment class with the following private member variables / attributes
Double referalFee
Include appropriate getters and setters.
Include 5 argument constructor with argument order Agent(String name,String source,String destination,double price,double referalFee).
Call the super method inside the constructor with four arguements, which calls the Shipment's constructor.
And this class includes the following method
No | Method Name | Method Description |
1 | double calculateShipmentAmount() | In this method, calculate and return the total amount. |
Create a CompanyShipment class,which extends Shipment class with the following private member variables / attributes
private Double luxuryTax
private Double corporateTax
Include appropriate getters and setters.
Include 6 argument constructor with argument order Company(String name,String source,String destination,double price,double luxuryTax,double corporateTax).
Call the super method inside the constructor with four arguements, which calls the Shipment's constructor.
And this class includes the following method
No | Method Name | Method Description |
1 | double calculateShipmentAmount() | In this method, calculate and return the total amount. |
Create a Main class to test the above 3 classes. Invoke the calculateShipmentAmount methods in the CompanyShipment and AgentShipment class for calculate the total shipment amount and return that calculated amount like sample output.
Problem Specification :
Calculate the total amount using method overriding.
In AgentShipment class the shipment price will be added to referal fee.
In CompanyShipment class, need some calculation to compute the total amount. The luxury tax and corporate tax are in percentage(%).
Input and Output Format: Print the total amount by two decimal places.
Refer sample input and output for formatting specifications.
[Note :Strictly adhere to the object oriented specifications given as a part of the problem statement.Use the same class names, attribute names and method names.]
[All text in bold corresponds to input and the rest corresponds to output.] Sample Input and Output 1:
Enter the shipment name :
NYK Line
Enter the source :
Perth
Enter the destination :
Gold Coast
Enter the price :
1800
1. Agent
2. Company
Enter your choice :
1
Enter the referal fee :
350.50 Agent details :
Name Source Destination Total Amount
NYK Line Perth Gold Coast 2150.50 Sample Input and Output 2:
Enter the shipment name :
Wan Hai Lines
Enter the source :
Ballarat
Enter the destination :
Albany
Enter the price :
2000
1. Agent
2. Company
Enter your choice :
2
Enter the luxury tax and corporate tax:
15
12.75 Company details :
Name Source Destination Total Amount
Wan Hai Lines Ballarat Albany 2555.00
Now, I am providing the given piece of Code. We just need to modify it as per the given criteria. Please update the code accoprdingly and send me back.
AgentShipment.Java:
public class AgentShipment{ private double referalFee;
public double getReferalFee() { return referalFee; }
public void setReferalFee(double referalFee) { this.referalFee = referalFee; } @Override double calculateShipmentAmount() { //fill code here. } }
Shipment.Java:
public class Shipment { protected String name; protected String source; protected String destination; protected double price;
public Shipment(String name, String source, String destination, double price) { this.name = name; this.source = source; this.destination = destination; this.price = price; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getSource() { return source; }
public void setSource(String source) { this.source = source; }
public String getDestination() { return destination; }
public void setDestination(String destination) { this.destination = destination; }
public double getPrice() { return price; }
public void setPrice(double price) { this.price = price; } double calculateShipmentAmount() { return Double.NaN; } }
CompanyShipment.Java:
public class CompanyShipment { private Double luxuryTax; private Double corporateTax;
public double getLuxuryTax() { return luxuryTax; }
public void setLuxuryTax(double luxuryTax) { this.luxuryTax = luxuryTax; }
public Double getCorporateTax() { return corporateTax; }
public void setCorporateTax(double corporateTax) { this.corporateTax = corporateTax; } @Override double calculateShipmentAmount() { //fill code here. } }
Manin.Java:
import java.io.*;
public class Main {
public static void main(String[] args) throws Exception {
String name,source,destination;
double price;
int choice;
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter the shipment name :");
name = br.readLine();
System.out.println("Enter the source :");
source = br.readLine();
System.out.println("Enter the destination :");
destination = br.readLine();
System.out.println("Enter the price :");
price =Double.parseDouble(br.readLine());
System.out.println("1. Agent 2. Company Enter your choice :");
choice = Integer.parseInt(br.readLine());
Shipment shipment;
if(choice == 1)
{
double referalFee;
System.out.println("Enter the referal fee :");
referalFee = Double.parseDouble(br.readLine());
System.out.println("Agent details :");
//fill code here.
System.out.format("%-15s %-15s %-15s %s ","Name","Source","Destination","Total Amount");
//fill code here.
}
else if(choice == 2)
{
double luxuryTax,corporateTax;
System.out.println("Enter the luxury tax and corporate tax:");
luxuryTax = Double.parseDouble(br.readLine());
corporateTax = Double.parseDouble(br.readLine());
System.out.println("Company details :");
//fill code here.
System.out.format("%-15s %-15s %-15s %s ","Name","Source","Destination","Total Amount");
//fill code here.
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
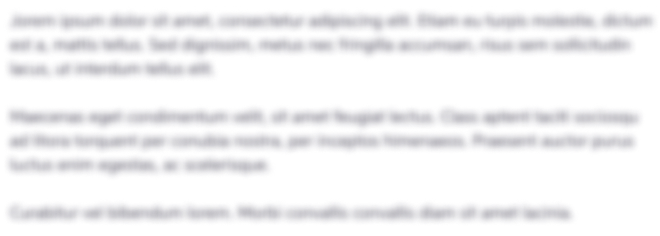
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started