Question
Core Java_Thread_Problem Statement 3 The payment process in the Shipping management takes a huge amount of time when it comes to a large number of
Core Java_Thread_Problem Statement 3
The payment process in the Shipping management takes a huge amount of time when it comes to a large number of customers. Because their implementation is processed one at a time so the customers are unhappy with the service provided for payment system. And so the technical team has decided to implement the payment process using multithreading to increase the performance of the system where parallel processing of payments is achieved. The payment details are received from the user in the following format payment id,amount, payment code For example, 123,5000,CHEQ 1245,7888,CC
Payment code | Payment mode |
CHEQ | The payment mode is by CHEQUE |
CC | The payment mode is by Credit Card |
Create a class named ChequeProcessingThread that implements the Runnable interface with the following private member variables
ArrayBlockingQueue queue
Include appropriate getters and setters. And include a parametrized constructor and methods as follows
Access specifier | Method name | Method description |
public | void addCheque(String val) | Add the string to the queue |
public | void run() | Override the run method to take values from the queue, split the payment details string, process the payment and print the result. |
Create another class named CreditCardProcessingThread implements the Runnable interface with the following private member variables
ArrayBlockingQueue queue
Include appropriate getters and setters. And include a parametrized constructor as follows
Access specifier | Method | Purpose |
public | void addCreditCard(String val) | Add the string to the queue |
public | void run() | Override the run method to take values from the queue, split the payment details string, process the payment and print the result. |
\
Create an instance of both the classes ChequeProcessingThread and CreditCardProcessingThread
Now create an instance of the Thread with the instance of ChequeProcessingThread as parameter and start the thread
Similarly create an instance of the Thread with the instance of CreditCardProcessingThread as parameter and start the thread
Read the input from the user in the main method. if the payment mode is cheque then the input is add to the ChequeProcessingThread thread . Similarly, if the payment mode is creditcard then the input is add to the CreditCardProcessingThread thread
In the run method split the string with comma and print the output in the following format
processing completed for payment id
For example Cheque processing completed for payment id 123 Credit card processing completed for payment id 47 Hints : Input to the thread class is send using the blocking queue which is maintained in the thread class. A BlockingQueue is typically used on thread produce objects, which another thread consumes. [Note :Strictly adhere to the object oriented specifications given as a part of the problem statement.Use the same class names, attribute names and method names.] Input Format The first line of input contains an integer n, the number of payments. Then the payment details are received in a comma-seperated string from the user in the following format payment id,amount,payment code Output Format The output contains string corresponding to each input. Please refer to the sample input and output for more details. [All text in bold corresponds to input and the rest corresponds to output.] Sample Input and Output Enter number of payment: 4 Enter all the payment details 123,5000,CHEQ Cheque processing completed for payment id 123 1245,7888,CC Credit card processing completed for payment id 1245 14587,23,CHEQ Cheque processing completed for payment id 14587 478,78,CC Credit card processing completed for payment id 47
Here I am providing the piece of Code. Please update the code based on the information provided and send me back. This program should run with the given input and output conditions:
Main.Java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public static void main(String args[]) throws IOException, InterruptedException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter number of payment:");
int numberOfPayments = Integer.parseInt(reader.readLine());
System.out.println("Enter all the payment details");
// fill in your code here
for(int i=0;i
//fill in your code here
}
// fill in your code here
}
}
CreditCardProcessingThread.Java
import java.util.concurrent.ArrayBlockingQueue;
public class CreditCardProcessingThread //fill in your code here { // fill in your attributes here
public void run() { //fill in your code here } public void addCreditCard(String val) { queue.add(val); } }
ChequeProcessingThread.Java
import java.util.Collection; import java.util.Iterator; import java.util.concurrent.ArrayBlockingQueue; import java.util.concurrent.BlockingQueue; import java.util.concurrent.TimeUnit;
public class ChequeProcessingThread //fill in your code here { ArrayBlockingQueue queue = new ArrayBlockingQueue(10);
public void run() { //fill in your code here } public void addCheque(String val) { //fill in your code here } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
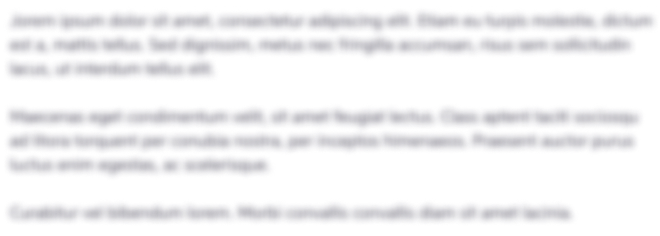
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started