Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Correct code and screenshot of the output This is the code: #include #include #include #include #include #include using namespace std; // implementing the dynamic List
Correct code and screenshot of the output This is the code:
#include
#include
#include
#include
#include
#include
using namespace std;
// implementing the dynamic List ADT using Linked List
class Node{
private:
int data;
Node* nextNodePtr;
public:
Node(){}
void setData(int d){
data = d;
}
int getData(){
return data;
}
void setNextNodePtr(Node* nodePtr){
nextNodePtr = nodePtr;
}
Node* getNextNodePtr(){
return nextNodePtr;
}
};
class List{
private:
Node *headPtr;
public:
List(){
headPtr = new Node();
headPtr->setNextNodePtr(0);
}
Node* getHeadPtr(){
return headPtr;
}
bool isEmpty(){
if (headPtr->getNextNodePtr() == 0)
return true;
return false;
}
void insert(int data){
Node* currentNodePtr = headPtr->getNextNodePtr();
Node* prevNodePtr = headPtr;
while (currentNodePtr != 0){
prevNodePtr = currentNodePtr;
currentNodePtr = currentNodePtr->getNextNodePtr();
}
Node* newNodePtr = new Node();
newNodePtr->setData(data);
newNodePtr->setNextNodePtr(0);
prevNodePtr->setNextNodePtr(newNodePtr);
}
void insertAtIndex(int insertIndex, int data){
Node* currentNodePtr = headPtr->getNextNodePtr();
Node* prevNodePtr = headPtr;
int index = 0;
while (currentNodePtr != 0){
if (index == insertIndex)
break;
prevNodePtr = currentNodePtr;
currentNodePtr = currentNodePtr->getNextNodePtr();
index++;
}
Node* newNodePtr = new Node();
newNodePtr->setData(data);
newNodePtr->setNextNodePtr(currentNodePtr);
prevNodePtr->setNextNodePtr(newNodePtr);
}
int read(int readIndex){
Node* currentNodePtr = headPtr->getNextNodePtr();
Node* prevNodePtr = headPtr;
int index = 0;
while (currentNodePtr != 0){
if (index == readIndex)
return currentNodePtr->getData();
prevNodePtr = currentNodePtr;
currentNodePtr = currentNodePtr->getNextNodePtr();
index++;
}
return -1; // an invalid value indicating
// index is out of range
}
void modifyElement(int modifyIndex, int data){
Node* currentNodePtr = headPtr->getNextNodePtr();
Node* prevNodePtr = headPtr;
int index = 0;
while (currentNodePtr != 0){
if (index == modifyIndex){
currentNodePtr->setData(data);
return;
}
prevNodePtr = currentNodePtr;
currentNodePtr = currentNodePtr->getNextNodePtr();
index++;
}
}
void IterativePrint(){
Node* currentNodePtr = headPtr->getNextNodePtr();
while (currentNodePtr != 0){
cout getData()
currentNodePtr = currentNodePtr->getNextNodePtr();
}
cout
}
void pairwiseSwap(){
// Implement the pairwiseSwap function
}
};
int main(){
int listSize;
cout
cin >> listSize;
int maxValue;
cout
cin >> maxValue;
srand(time(NULL));
List integerList; // Create an empty list
for (int i = 0; i
int value = 1 + rand() % maxValue;
integerList.insert(value);
}
cout
Linked List class (named: List) for this Consider the implementation of the Singly given to you question. Your task is to add a member function called pairwiseS wap) to the Singly Linked List class such that it can be called from the main function (as given in the code) to swap the elements of an integerList (an object of the class List) pairwise and print the updated list. For example. if the List before the pairwiseSwap is 4 .> 5 .> 2 > 3-> l-> 6, after the pairwiseSwap, the contents of the list should be: 5->4->3-26>1. If the List has an odd number of elements, like: 4>5->2->3->1, then after the pairwiseSwap, the contents of the list should be: 5-4 32-> 1 Test your code with 10 elements and 11 elements (with a maximum value of 25 in each case) and take screenshots of the List before and after pairwiseS wap, as printed in the main function You need to submit the following as part of your answer for this question: o) the complete code for the Node class. List class (including the pairwiseS wap ) function) and the main function. (ii) Snapshots of the execution of the code with 10 elements and I1 elements (with a maximum value of 25 in each case integerList.IterativePrint();
integerList.pairwiseSwap();
cout
integerList.IterativePrint();
return 0;
}

Step by Step Solution
There are 3 Steps involved in it
Step: 1
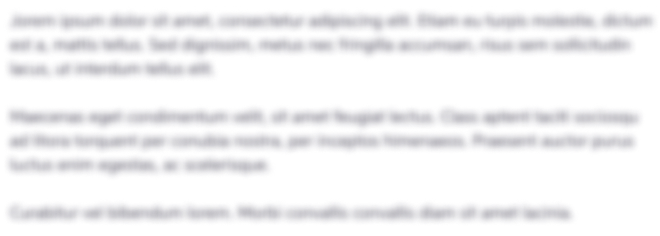
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started