Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Correct the logical error in the loop! #include #include using namespace std; //declaring variables char recommendedpackage, answer='1'; string packagedetails, fname, packagename; double additionaldata, monthlypayment, balanc,
Correct the logical error in the loop!
#include
#include
using namespace std;
//declaring variables
char recommendedpackage, answer='1';
string packagedetails, fname, packagename;
double additionaldata, monthlypayment, balanc, additionalminutes, cost, savedamount;
double const basicadditionaldata = 0.3, basicmonthlypayment = 30.0, limitedmonthlypayment = 120, unlimitidmonthlypayment = 200;
/*creating get in value function to take in the users first and last name, phone number, used minets and data, and
the choosen package*/
void getinvalue(string & fname, string lname, string & phone, int &minutes, int &dataused,
char & package);
void getinvalue(string & fname, string lname, string & phone, int &minutes, int &dataused,
char & package) {
//outputs so the user can enter her/his first and last name
cout
cin >> fname;
cout
cin >> lname;
do {
cout
cin >> phone;
cout
cin >> package;
cout
cin >> minutes;
cout
cin >> dataused;
} while (answer == 1);
}
// creating another function to print out the price based on the used minutes and data and the choosen package
double calc_price(int minutes, int dataused, char package);
double calc_price(int minutes, int dataused, char package) {
//for the differnet 3 different cases
switch (package) {
//case 1: if the user chosed plan b or B
case 'b':
case 'B':
//declaring the package name and the details of it
package = 'B';
packagename = "Basic";
packagedetails = "30 AED / month includes 30 minutes. Additional minute costs 30 fills. Data usage is 30 fills per 1MB.";
//if the user exeded the set minutes then the additial charges will apply
if (minutes > 30) {
additionalminutes = 0.3;
}
//if not then no additional charges will apply
else {
additionalminutes = 0;
}
//to calculate the total balance
return cost = basicadditionaldata * dataused + additionalminutes * (minutes - 30) + basicmonthlypayment;
//breaking the previous case
break;
//case 2: if the user chosed plan l or L
case 'L':
case 'l':
//declaring the package name and the details of it
package = 'L';
packagename = "Limited";
packagedetails = "120 AED/month includes 60 minutes, 100MB of data. Additional minute costs 20 fills, and any extra data usage beyond the 100MB costs 30 fills per 1MB.";
//if the user exeded the set minutes then the additional charges will apply
if (minutes > 60) {
additionalminutes = 0.2;
}
//if not then no additional charges will apply
else {
additionalminutes = 0;
}
//if the user exedde the set data then the additional charges will apply
if (dataused > 100) {
additionaldata = 0.3;
}
//if not then no additional charges will apply
else {
additionaldata = 0;
}
//to calculate the total balance
return cost = limitedmonthlypayment + (dataused - 100)*additionaldata + (minutes - 60)*additionalminutes;
//breaking the previous case
break;
//case 3: if the user chosed plan U or u then
case 'U':
case 'u':
//declaring the package name and the details of it
package = 'U';
packagename = "Unlimited";
packagedetails = "200 AED/month includes unlimited calling minutes and unlimited data usage. ";
return cost = unlimitidmonthlypayment;
//breaking the previous case
break;
//for any other choices it will set s default and print out the following
default:
cout
}
}
//creating a new function to recommend the best packages according to the saved amount
char recommendpackage(int minutes, int data);
char recommendpackage(int minutes, int data) {
//declaring new variables
double comparinglimited, comparingbasic, comparingunlimited=200;
//if the user exeded the set minutes then the additial charges will apply for the basic
if (minutes > 30) {
additionalminutes = 0.3;
}
//to calculate the compared value for the basic
comparingbasic = basicadditionaldata * data + additionalminutes * (minutes - 30) + basicmonthlypayment;
//if the user exeded the set minutes then the additional charges will apply for the limited
if (minutes > 60) {
additionalminutes = 0.2;
}
//if the user exeded the set data then the additional charges will apply for the limited
if (data > 100) {
additionaldata = 0.3;
}
//to calculate the compared value for the limited
comparinglimited = limitedmonthlypayment + (data - 100)*additionaldata + (minutes - 60)*additionalminutes;
//if compared basic is less than both the cpombared limited and unlimited then the saved amount will equal to
if ((comparingbasic
savedamount = cost - comparingbasic;
//if the saved ampount is greater than zero then the recommended package will be
if (savedamount > 0) {
return recommendedpackage = 'B';
}
}
//if compared limited is less than both the cpombared basic and unlimited then the saved amount will equal to
if ((comparinglimited
savedamount = cost - comparinglimited;
//if the saved ampount is greater than zero then the recommended package will be
if (savedamount > 0) {
return recommendedpackage = 'L';
}
}
//if compared unlimited is less than both the cpombared limited and basic then the saved amount will equal to
if ((comparingunlimited
savedamount = cost - comparingunlimited;
//if the saved ampount is greater than zero then the recommended package will be
if (savedamount > 0) {
return recommendedpackage = 'U';
}
}
else if (savedamount
cout
}
}
//creating a new function to print out the invoice
void displayInvoice(string fname, string lname, string phone, int minutes, int dataused, char
package, double cost);
void displayInvoice(string fname, string lname, string phone, int minutes, int dataused, char
package, double cost) {
//if the user entered 1 as an answer, the following loop will be repeated until it breaks
while (answer == '1') {
//to print out the users the invoice
cout
cout
cout
cout
cout
cout
cout
//if the saved amount was greater than and does not equal to zero then it should print out the following
if ((savedamount != 0) && (savedamount > 0)) {
cout
}
//else if the saved amount eas less than or equal to zero then it should print out the following
else if (savedamount
cout
}
cout
cout
cin >> answer;
// if the user entered an invalid answer other than 2 or 1
if ((answer != '1') && (answer != '2')) {
cout
cout
cout
cin >> answer;
cout
arnin Solving problems using C++ Implement a program that makes use of the following programming concepts o Branches o Loops o Functions pass by value and by reference o Calling functions Problem RaeRae Mobile Communication Service provides their customers three different mobile plans i which customers can choose a package that suite them best. RaeRae mobile service plans are a follows Plan Basic: 30 AED/month includes 30 minutes. Additional minute costs 30 fills. Data usage is 30 fill per 1MB Plan Limited: 120 AED/month includes 60 minutes, 100MB of data. Additional minute costs 20 fills and any extra data usage beyond the 100MB costs 30 fills per 1MB. Plan Unlimited: 200 AED/month includes unlimited calling minutes and unlimited data usage Write a program that prompts the user for a customer first and last name, phone number, wha mobile package they use, and the number of minutes and data used that month. With tha information, calculate their monthly bill and display it to them. You will also include in their bill eithe a recommendation that they switch to a more cost effective mobile package or thank them for bein a customer if they have the appropriate package After the invoice is printed, the program shall ask the user if they need to enter the information fo another mobile phone or if they wish to exit. If they select the option "Yes" to enter another phon number, prompt the user again for only the phone number, the minutes used, and the data user during the month to calculate the bill for the other phone number. The program shall continuoush run until the user select the option "No" for not entering another phone number. In this case, th program will exit. create getinValues function that takes in the parameters as specified below. The function will get the input from the user for the first name, last name, phone number, minutes used, data used, and package selection . void getInValue(string &fname, string &1name, string &phone, int 8minutes, int &dataused, char &package) .calc_price function must be defined as specified below. The function takes in the minutes used data used, and package selection as input parameters and returns the price based on the use usage double calc price(int minutes, int dataused, char package) }
}
//if the user entered 2 as an answer the following output will print out
if (answer == '2') {
cout
}
}
void main() {
string lname, fname, phone;
char package;
int dataused, minutes, data=1;
getinvalue(fname, lname, phone, minutes, dataused, package);
calc_price(minutes, dataused, package);
recommendpackage(minutes, data);
displayInvoice(fname, lname, phone, minutes, dataused,
package, cost);
}
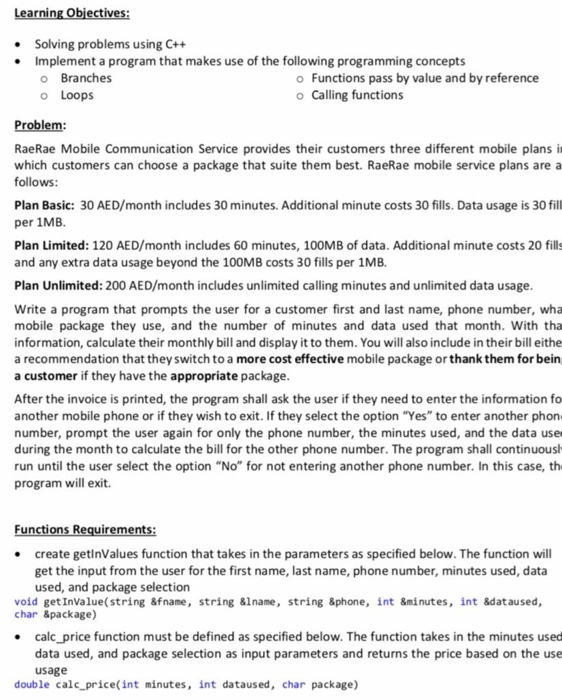
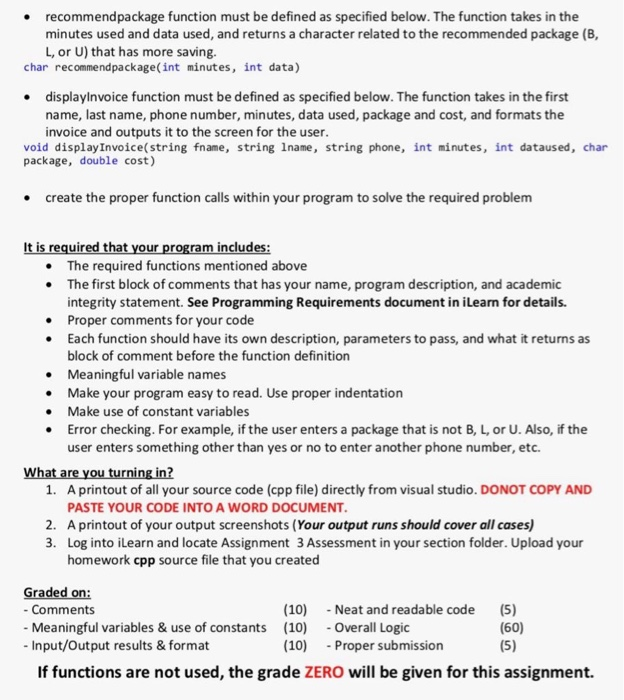
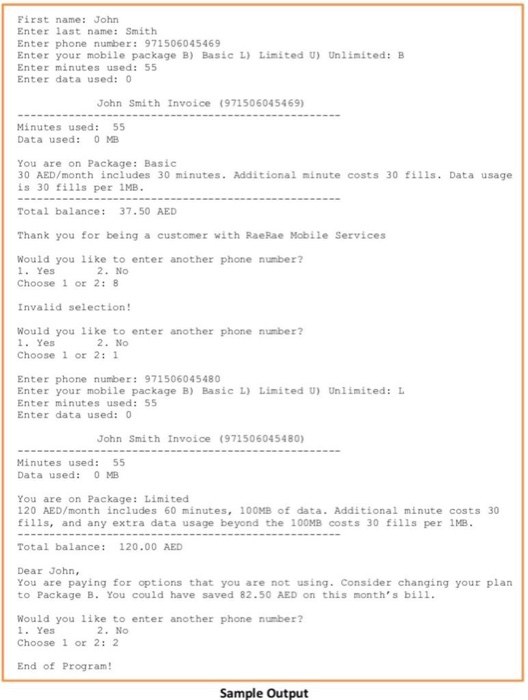
Step by Step Solution
There are 3 Steps involved in it
Step: 1
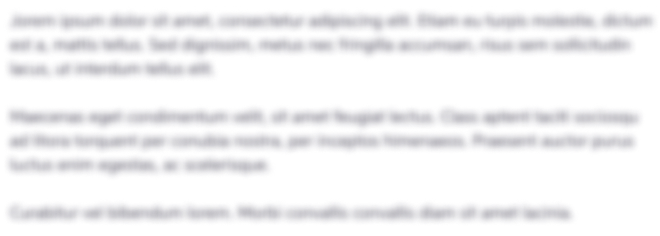
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started