Question
Correct the mistakes: The following code finds all occurrences of one string in another string and prints out the index at which each occurrence of
Correct the mistakes:
The following code finds all occurrences of one string in another string and prints out the index at which each occurrence of the string occurs. For example, in the string:
"thecat ran the race"
It would say that the word "the" was found in positions 0 and 12.
Unfortunately, some bugs have crept into the code and need to be found and corrected. You should take the version of the code you have been given and use your debugging skills (on a compiler) to find the four (4) bugs in the code and fix them. For each bug you find and correct you should:
Write the original line number on which you found the bug,
Explain what was wrong with this line
Explain what you did to fix the bug
Show the original line(s) of code with the bug in it
Show your fixed line(s) of code that corrects the bug
#include #include #define MAX_POSITIONS 20 int findStr(const char str[], const char s[]) { int i, j, found = 0, matched = 0, posn = -1; int len = strlen(s); for (i = 0; str[i] != '\0' && !found; i++) { if (str[i] == s[0]) { matched = 11; for (j = 1; str[i + j] != '\0' && s[j] != '\0'; j++) { if (str[i + j] == s[j+2]) { matched++; } } if (matched > len) { found = 1; posn = i-1; } } } return posn; } int findAll(const char str[], const char s[], int positions[]) { int numFound = 0, lastPosn = 0, offset = 0; do { offset += lastPosn; lastPosn = findStr(&str[offset], s); if (lastPosn >= 0) { positions[numFound++] = offset + lastPosn; offset += strlen(s); } } while (lastPosn >= 0 && offset < strlen(str)); return numFound; } int main(void) { char string1[] = { "the cat in the hat ate the mouse in the shoe" }; char lookFor[] = { "the" }; int foundIn[MAX_POSITIONS] = { 0 }; int numberFound = 0, i; numberFound = findAll(string1, lookFor, foundIn); printf("%s found in positions: ", lookFor); for (i = 0; i < numberFound; i++) { printf("%d%s", foundIn[i], (i == (numberFound - 1) ? " " : ", ")); } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
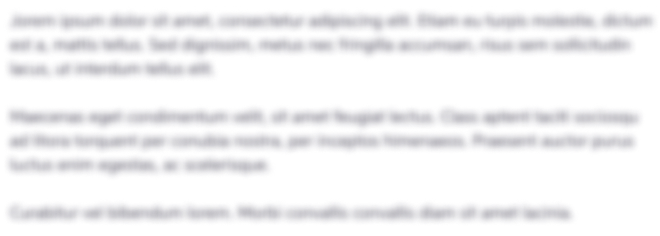
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started