Question
COSC 1010 Shapes and Lines Help!!! Here is the Line Class, import java.awt.*; import java.awt.geom.*; /** * A line that can be manipulated and that
COSC 1010 Shapes and Lines Help!!!
Here is the Line Class,
import java.awt.*; import java.awt.geom.*;
/** * A line that can be manipulated and that draws itself on a canvas * * @author Derek Green (a slightly modified copy of the Circle class written by Michael Kolling and David J. Barnes) * @version 1.0 (2/7/04) */ public class Line { private int x1; private int y1; private int x2; private int y2; private String color; private boolean isVisible;
/** * Create a new line using default values. */ public Line() { x1 = 0; y1 = 0; x2 = 10; y2 = 10; color = "blue"; isVisible = false; }
//-------------------------------------------------------------------------------- // THIS IS WHERE THE CODE NEEDS TO GO //--------------------------------------------------------------------------------
// Write all of your code for the problems here between the lines.
//--------------------------------------------------------------------------------
/** * Make this line visible, if already visible do nothing. */ public void makeVisible() { if (!isVisible){ isVisible = true; draw(); } }
/** * Make this line invisible, if already invisible do nothing. */ public void makeInvisible() { if(isVisible){ erase(); isVisible = false; } }
/** * Move the line 20 pixels to the right without deformation. */ public void moveRight() { moveHorizontal(20); }
/** * Move the line 20 pixels to the left without deformation. */ public void moveLeft() { moveHorizontal(-20); }
/** * Move the line 20 pixels up without deformation */ public void moveUp() { moveVertical(-20); }
/** * Move the line 20 pixels down without deformation. */ public void moveDown() { moveVertical(20); }
/** * Move the line horizontally by 'distance' pixels without deformation. */ public void moveHorizontal(int distance) { erase(); x1 += distance; x2 += distance; draw(); }
/** * Move the line vertically by 'distance' pixels without deformation. */ public void moveVertical(int distance) { erase(); y1 += distance; y2 += distance; draw(); }
/** * Slowly move the line horizontally by 'distance' pixels without deformation. */ public void slowMoveHorizontal(int distance) { int delta;
if(distance < 0) { delta = -1; distance = -distance; } else { delta = 1; }
for(int i = 0; i < distance; i++) { x1 += delta; x2 += delta; draw(); } }
/** * Slowly move the line vertically by 'distance' pixels without deformation. */ public void slowMoveVertical(int distance) { int delta;
if(distance < 0) { delta = -1; distance = -distance; } else { delta = 1; }
for(int i = 0; i < distance; i++) { y1 += delta; y2 += delta; draw(); } }
/** * Change the color. Valid colors are "red", "yellow", "blue", "green", * "magenta" and "black". */ public void changeColor(String newColor) { if (newColor.equals("red") || newColor.equals("yellow") || newColor.equals("blue") || newColor.equals("green") || newColor.equals("magenta") || newColor.equals("black")){ color = newColor; draw(); } }
/** * Draw the line. */ private void draw() { if(isVisible) { Canvas canvas = Canvas.getCanvas(); canvas.draw(this, color, new Line2D.Double(x1, y1, x2, y2)); canvas.wait(10); } }
/* * Erase the line on screen. */ private void erase() { if(isVisible) { Canvas canvas = Canvas.getCanvas(); canvas.erase(this); } } }
Here are the directions,
1. Create a constructor that allows you to specify the 2 endpoints of the line being created. Leave the original constructor alone. Your program should have 2 constructors now.
2. Create an accessor method that returns the color of the line.
3. Create a mutator method that lets you change the (x, y) coordinates of the endpoints to newly specified values. Don't just change values by some constant, allow new values to be entered for each coordinate. Don't forget to redraw the line after you change it.
4. Create an accessor method that returns the length of the line as an integer (not a double).
5. Create a method that prints out the midpoint of a line (print it out, this method returns nothing: void). Use the following format (i.e. if I use your program to create a line from (10, 20) to (100, 120) this method should print exactly as follows): The midpoint of the line from (10, 20) to (100, 120) is (55, 70). Hint: The midpoint remains unchanged if you swap the endpoints.
6. Create a method that finds the slope of a line. The method will print out the slope if it is defined, otherwise it will print out the x-value of the line. Print the slope as a fraction int/int (i.e. do not do the division. Note: Since the origin is in the upper left corner with x increasing to the right and y increasing down the slope will be different from what you would expect. What is different? Fix your code so that lines rising from left to right have a positive slope and lines falling from left to right have a negative slope.) If the slope is not defined the method should return false. If it is defined the method returns true. Here are several examples using different lines: For a line with endpoints (0,0) and (100, 200), acceptable outputs are: "Slope is : 200/-100" or "Slope is : -200/100" For a line with endpoints (100,0) and (0,200), acceptable outputs are: "Slope is : 200/100" or "Slope is : -200/-100" For a line with endpoints (0,100) and (200,100), the acceptable output is: "Slope is : 0" For a line with endpoints (100,0) and (100,200), the acceptable output is: "Slope is undefined: x = 100"
Step by Step Solution
There are 3 Steps involved in it
Step: 1
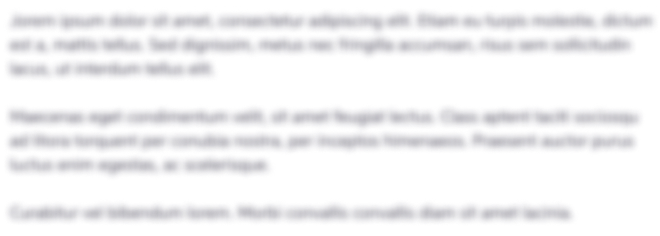
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started