Question
COSC 143 7 Programming Assignment 2 Programming Assignment 2 You will write a program that reads a binary file that contains records of creatures. Each
COSC 143
7
Programming Assignment
2
Programming Assignment
2
You will write a program that reads a binary file that contains records of creatures. Each record can be
stored in a Creature struct. You should present the user with a menu of three options: display a specific
creature, display
all the creatures sorted by name, or quit. If the user chooses to display a specific
creature, you should ask the user which creature to display.
You will be supplied with a starter file PA
2
_
Starter
.cpp. You will need to complete some of the code in
main a
nd write the definitions for the three functions. For sorting, you should use the sort function
found in the algorithm header file. You can read more about this function here:
http://www.cplusplus
.com/articles/NhA0RXSz/
.
Make sure you use good programming style.
Name your file PA
2
_lastName_firstName.cpp, replacing lastName with your actual last name and
firstName with your actual first name.
Submit only the cpp file.
A possible sample run will look
as follows:
Menu
1. Display a specific creature
2. Display all creatures sorted by name
3. Quit
Enter your choice (1, 2, 3):
1
Which creature do you want to view?
Enter 1 to 10:
3
Creature Report
Name: Kobold
Type: Small Humanoid
HP: 4
Armor Class: 15
S
peed: 6
Press any key to continue . . .
COSC 143
7
Programming Assignment
2
Menu
1. Display a specific creature
2. Display all creatures sorted by name
3. Quit
Enter your choice (1, 2, 3):
1
Which creature do you want to view?
Enter 1 to 10:
8
Creature Report
Name: Frost Worm
Type: Huge
Magical Beast
HP: 147
Armor Class: 18
Speed: 6
Press any key to continue . . .
Menu
1. Display a specific creature
2. Display all creatures sorted by name
3. Quit
Enter your choice (1, 2, 3):
1
Which creature do you want to view?
Enter 1 to 10:
15
Inva
lid entry. Try again.
Which creature do you want to view?
Enter 1 to 10:
2
Creature Report
Name: Orc
Type: Medium Humanoid
HP: 5
Armor Class: 13
Speed: 6
Press any key to continue . . .
COSC 143
7
Programming Assignment
2
Menu
1. Display a specific creature
2. Display all creatures
sorted by name
3. Quit
Enter your choice (1, 2, 3):
2
Basili
s
k
Medium Magical Beast
45
16
4
Clay Golem
Large Construct
90
22
4
Ethereal Filcher
Medium Abberation
22
17
8
Frost Worm
Huge Magical Beast
147
18
6
Goblin
Small Humanoid
5
15
6
Harpy
Medium
Monstrous Humanoid
31
13
4
COSC 143
7
Programming Assignment
2
Hobgoblin
Medium Humanoid
6
15
6
Kobold
Small Humanoid
4
15
6
Medusa
Medium Monstrous Humanoid
33
15
6
Orc
Medium Humanoid
5
13
6
Press any key to continue . . .
Menu
1. Display a specific creature
2. Display all
creatures sorted by name
3. Quit
Enter your choice (1, 2, 3):
3
Press any key to continue . .
main.cpp file
/****************************** Name Date PA1_Starter.cpp Description ********************************/
// Headers #include
// Global variables const int CREATURE_NAME_SIZE = 30; // Max length of a creature name const int CREATURE_TYPE_SIZE = 26; // Max length of a creature type const string FILENAME = "creatures.dat"; // Name of file that contains the data
// struct used to create records in file struct Creature { char name[CREATURE_NAME_SIZE]; // Name of the creature char creatureType[CREATURE_TYPE_SIZE]; // Type of creature int hp; // Hit points for creature int armor; // Armor class for creature int speed; // Speed of creature };
// This function returns true if the name of the left creature is less than the name of the right creature // Use this function when running the sort function bool sortByName(const Creature &lhs, const Creature &rhs) { string name1(lhs.name), name2(rhs.name); return name1 < name2; }
// Function declarations // You will need to code the definitions for each of these functions int getCreatureNumber(int numCreatures); void displayCreature(fstream& file, int num); void displaySorted(fstream& file);
int main() { char choice; // choice made by user for menu fstream creatureFile; // file stream for input file int numCreatures; // the number of creatures saved to the file // Open the creatureFile for input and binary (one statement of code)
// Get the number of creatures in the file (two statements of code) // The number of creatures should be assigned to numCreatures
do { cout << "Menu" << endl; cout << "1. Display a specific creature "; cout << "2. Display all creatures sorted by name "; cout << "3. Quit "; cout << "Enter your choice (1, 2, 3): "; cin >> choice;
while (cin.get() != ' ');
switch (choice) { case '1': // Display a specific creature displayCreature(creatureFile, getCreatureNumber(numCreatures)); break;
case '2': // Display all the creatures in order displaySorted(creatureFile); break;
case '3': // Quit break;
default: cout << "Invalid option. "; break; }
if (choice != '3') { system("PAUSE"); system("CLS"); }
} while (choice != '3');
creatureFile.close(); // Make sure we place the end message on a new line cout << endl;
// The following is system dependent. It will only work on Windows system("PAUSE");
/* // A non-system dependent method is below cout << "Press any key to continue"; cin.get(); */ return 0; }
/******************************************************************* getCreatureNumber gets and returns the record number from the file that the user would like to be displayed PARAM: numCreatures should be the value of the total number of records (creatures) in the file PRE: numCreatures contains a value that is equal to the number of records in the file POST: A value between 1 and numCreatures is returned as selected by the user NOTE: Do not allow a value less than 1 or greater than numCreatures to be returned ********************************************************************/ int getCreatureNumber(int numCreatures) {
}
/******************************************************************* displayCreature displays record number num from file PARAM: file is a fstream that should be open for input and binary num contains the record number that is to be read in file PRE: file is a fstream that is open for input and binary num is a value between 1 and the number of records in file POST: The record number num is displayed to the monitor ********************************************************************/ void displayCreature(fstream& file, int num) {
}
/******************************************************************* displaySorted should read file into a vector. It should then sort the vector by the name of the creature. Last it should display the vector PARAM: file is a fstream that should be open for input and binary PRE: file is open for input and binary POST: Each record is displayed sorted by the name of the creature ********************************************************************/ void displaySorted(fstream& file) {
}
creature.dat file
Harpy Medium Monstrous Humanoid Orc Medium Humanoid Ethereal Filcher Medium Abberation Hobgoblin Medium Humanoid Basilik Medium Magical Beast - Frost Worm Huge Magical Beast Clay Golem Large Construct Z Medusa Medium Monstrous Humanoid ! Kobold Small Humanoid Goblin Small Humanoid
Step by Step Solution
There are 3 Steps involved in it
Step: 1
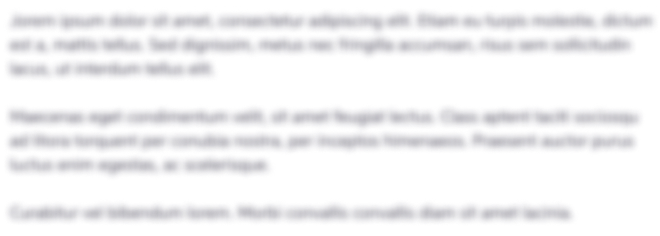
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started