Question
could do you solve each parts please and show me in each parts what should I put Make a copy of your NetBeans project from
could do you solve each parts please and show me in each parts what should I put
Make a copy of your NetBeans project from Lab Project 8: Graphical Object Classes I. Then, open it in NetBeans (remember: launch NetBeans, and then open the project using File > Open Project... (Ctrl+Shift+O) ).
this is lab 8
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package nb_grafix_object_thing;
import java.awt.Graphics; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.ArrayList; import javax.swing.Timer;
/** * Blank form for creating graphical objects * * @author */ public class NB_Grafix_Object_Thing extends javax.swing.JFrame implements ActionListener { private ArrayList Stuff; // generic list of objects private Timer timer; private static final int DELAY = 3000; // 3 seconds
/** * Creates new form Thing_Form */ public NB_Grafix_Object_Thing() { initComponents(); // create the generic stuff ArrayList Stuff = new ArrayList(); // create a timer for this Form // with the given delay timer = new Timer(DELAY, this); // start the timer timer.start(); } // end constructor
/** * method is called each time the form is repainted * * @param graphics the Form's Graphics object */ @Override public void paint(Graphics graphics) { // draw the window title, menu, buttons super.paint(graphics); // get the Graphics context for the canvas Graphics canvas = canvasPanel.getGraphics(); /*** ADD CODE TO DRAW ON THE canvas AFTER THIS LINE ***/ } // end paint method /** * method is called each time mouse is clicked in the form * * add code that does something with the click, like make things... * * @param xCoord x-coordinate of the click * @param yCoord y-coordinate of the click */ public void mouseClickedAt(int xCoord, int yCoord) { /*** ADD CODE TO REACT TO USER MOUSE CLICK AFTER THIS LINE ***/ } // end mouseClickedAt method /*************************************************** * WARNING: DO NOT ADD ANY CODE BELOW THIS LINE!!! ***************************************************/
/** * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always * regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // //GEN-BEGIN:initComponents private void initComponents() {
canvasPanel = new javax.swing.JPanel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); setTitle("Graphical Objects");
canvasPanel.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { canvasPanelMouseClicked(evt); } });
javax.swing.GroupLayout canvasPanelLayout = new javax.swing.GroupLayout(canvasPanel); canvasPanel.setLayout(canvasPanelLayout); canvasPanelLayout.setHorizontalGroup( canvasPanelLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGap(0, 999, Short.MAX_VALUE) ); canvasPanelLayout.setVerticalGroup( canvasPanelLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGap(0, 737, Short.MAX_VALUE) );
getContentPane().add(canvasPanel, java.awt.BorderLayout.CENTER);
setSize(new java.awt.Dimension(1015, 775)); setLocationRelativeTo(null); }// //GEN-END:initComponents
private void canvasPanelMouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_canvasPanelMouseClicked mouseClickedAt(evt.getX(), evt.getY()); }//GEN-LAST:event_canvasPanelMouseClicked
/** * @param args the command line arguments */ public static void main(String args[]) { /* Set the Nimbus look and feel */ // /* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel. * For details see http://download.oracle.com/javase/uiswing/lookandfeel/plaf.html */ try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(NB_Grafix_Object_Thing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(NB_Grafix_Object_Thing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(NB_Grafix_Object_Thing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(NB_Grafix_Object_Thing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } // //
/* Create and display the form */ java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new NB_Grafix_Object_Thing().setVisible(true); } }); }
// Variables declaration - do not modify//GEN-BEGIN:variables private javax.swing.JPanel canvasPanel; // End of variables declaration//GEN-END:variables
@Override public void actionPerformed(ActionEvent ae) { repaint(); }
}
Lab 9.1
The outlined boxes are a little hard to see in the form when drawn. Open the Box object class and modify its draw method so that it draws a filled box in its color (using the Graphics method fillRect), then calls the Graphics method drawRect to draw an outline of the box in black ( you can use the predefined Color constant Color.BLACK in the second call to the Graphics method setColor).
Lab 9.2
In the Box object class, add the following:
private member integer variables deltaX and deltaY
constructor code that initializes deltaX and deltaY to small positive integers (e.g., between -3 and +3)
a private move member method that increments the member variable x by deltaX and the member variable y by deltaY
Modify Box's draw method so that it moves the box (by calling its move method) before actually drawing.
Test your modified Box class by building/running the program; your boxes should now move after they are created!
Lab 9.3
Download the image file ThinkingDuke.png
Once the image file is downloaded, copy it to your project folder: for example, src > cps151_lab8 Create a Picture object class (similar to the Box object class) with:
private member integer variables x and y
private member integer variables deltaX and deltaY
private member variable image, whose type is javax.swing.ImageIcon
constructor code that: initializes x and y to the first and second integer parameters, respectively initializes deltaX and deltaY to small positive integers (e.g., between -3 and +3) initializes the image variable: for example, image = new javax.swing.ImageIcon("src/cps151_lab8/ThinkingDuke.png")
a public paint method with a Graphics context grafix that: calls its move method (below) to move has the image paint itself by calling its paintIcon method with the given grafix note: you may assume null for the first (Component) argument in the call to paintIcon
a private move method that increments the member variable x by deltaX and the member variable y by deltaY
Modify the application class (e.g., cps151_lab8):
Change the mouseClickedAt method so that it creates and adds Picture objects to the Stuff list (instead of Box objects)
In the paint method, change the for-each loop so that it draws Picture objects (instead of Box objects)
Test your Picture class and the modified the application class by building/running the program. Each mouse click in the form should create images of Duke that move after they are created!
I need code for these:
the modified Box class
the Picture class
the application class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
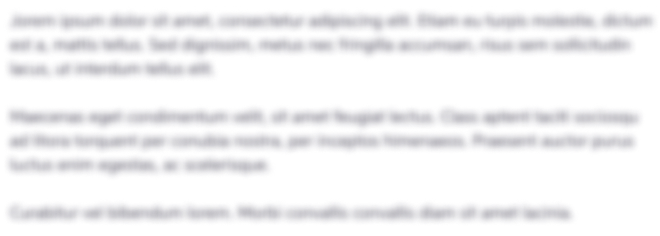
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started