Question
Could I get some help designing this sort of program in java? This week youll be writing a system to help the Department of Motor
Could I get some help designing this sort of program in java?
This week youll be writing a system to help the Department of Motor Vehicles (DMV) to organize people coming into the service center for various appointments. Like many businesses, people go to the DMV office for many different reasons:
Some people are there because they are getting their first drivers license. Theyll have to take a test.
Other people just need to renew their license. This doesnt require a test.
Other people are moving from another state, and need to transfer their license.
Finally some people are coming in to have their license reactivated after a violation.
Each employee that works there may only deal with one type of customer. So when the customer comes in, they are assigned a ticket number, which is something like A12 or C28, or D92. The letter represents what type of customer they are, while the number is their place in line for that type of customer.
Thus one employee may only deal with people getting their first license all day. They will start the day with A1, and move onto A2, A3 and then A4
For renewals, there may be 3 people who do renewals, so theyll pull B1, B2 etc.
In this system, weve decided the code is:
A: Take test for first license
B: Renew a license
C: Move from out of state
D: Violations/Suspended licenses
Each of these tasks requires different information, so theyve asked you to create an OOP that allows them to see how many people are in the queue at any given time.
Tasks:
First create an abstract class called Customer.
The customer class should have a private attribute for letter (char) and number (int) that together will tell us the customer ticket number (e.g. A15)
Youll need static variables to keep track of what the next A number will be, what the next B number will be, and the same for C and D.
Create a default constructor that sets letter to X and number to 0. This should never be used, but if any customer ends up with X0 well know there was an error Create an overloaded constructor that takes in a letter (we expect it to be passed A, B, C or D).
If the letter passed in is A, let letter to A, and number to the next A value, increment the static variable thats keeping track of the next A value.
Do the same for B, C and D.
If the letter passed in was anything else, let the letter to X and the number to 0 and print out Error, letter must be A, B, C or D Create a protected method called getTicketNumber which returns a string representation of the letter and number for that object (e.g. A47, or C15)
Create an abstract method called getCustomerInfo that takes no parameters and returns a string.
Next create a child class of Customer called NewTest. It will need to keep track of the customer's name (string) and dateOfBirth (string).
Create a constructor that takes in a name and date of birth (both strings):
Calls the parents constructor with the letter A as a parameter. This is because all new test customers get A numbers.
Sets the name and dateOfBirth attributes in the object.
Override getCustomerInfo to return New Drivers License. Ticket Number A15. Name: Jane DOB: 02/02/05
Note all the information above should represent the actual values this object has, not the placeholders in the above example.
Next create a child class of Customer called Renew.
It will need to keep track of the customer's name (string).
Create a constructor that takes in a name (string):
Calls the parents constructor with the letter B as a parameter. This is because all renewal customers get B numbers.
Sets the name attribute in the object.
Override getCustomerInfo to return Renewal License. Ticket Number B72. Name: Fred
Note all the information above should represent the actual values this object has, not the placeholders in the above example.
Next create a child class of Customer called Move. It will need to keep track of the customer's name (string) and what state they moved from (string).
Create a constructor that takes in a name (string) and previous state (string):
Calls the parents constructor with the letter C as a parameter. This is because all moved customers get C numbers.
Sets the name and previous state attributes in the object. Override getCustomerInfo to return Moved from NY. Ticket Number C02. Name: Fabian
Note all the information above should represent the actual values this object has, not the placeholders in the above example.
Next create a child class of Customer called Suspended.
It will need to keep track of the customer's name (string) and the violation they committed (string).
Create a constructor that takes in a name (string) and offense (string):
Calls the parents constructor with the letter D as a parameter. This is because all renewal customers get D numbers.
Sets the name and violation attribute in the object.
Override getCustomerInfo to return Violation: DUI. Ticket Number B72. Name: Grace
Note all the information above should represent the actual values this object has, not the placeholders in the above example.
In the main method of your driver create an ArrayList (Java) or List (C#) of Customer. Call the method menu passing it the arraylist/list.
In your driver class write a method called menu. It should take in an ArrayList (Java) or List (C#) of Customers.
Print out the menu and read in the users choice (see below for exact text of menu).
If the user chooses 1, prompt them for a name and date of birth. Insert into the ArrayList/List a new object of type NewTest with that data in it.
If the user chooses 2, prompt them for a name. Insert into the ArrayList/List a new object of type Renew with the data in it.
If the user chooses 3, prompt them for a name and the state they moved from. Insert into the ArrayList/List a new object of type Move with the data in it.
If the user chooses 4, prompt them for a name and the nature of the violation they committed. Insert into the ArrayList/List a new object of type Suspended with the data in it.
If the user chooses 5, use a loop to print out the customers info for all customers in the queue. Note each type of customer has a getCustomerInfo method you can call, and itll return the correct info.
The menu should keep prompting the user until they select 6.
Example Runs: [User input in red] 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 1 What is your name? Conor What is your date of birth? 09/09/03 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 1 What is your name? Sarah What is your date of birth? 2/10/05 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 2 What is your name? Nick 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 3 What is your name? Paula What state did you move from? MO 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 4 What is your name? Russ What violation did you commit? DUI 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 1 What is your name? Jane What is your date of birth? 01/01/65 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 5 New Drivers License. Ticket Number: A1. Name: Conor DOB: 09/09/03 New Drivers License. Ticket Number: A2. Name: Sarah DOB: 2/10/05 Renewal License. Ticket Number: B1. Name: Nick Moved from MO. Ticket Number: C1. Name: Paula Violation: DUI. Ticket Number: D1. Name: Russ New Drivers License. Ticket Number: A3. Name: Jane DOB: 01/01/65 1. Take test for new license 2. Renew existing license 3. Move from out of state 4. Answer citation/suspended license 5. See current queue 6. Quit 6
Step by Step Solution
There are 3 Steps involved in it
Step: 1
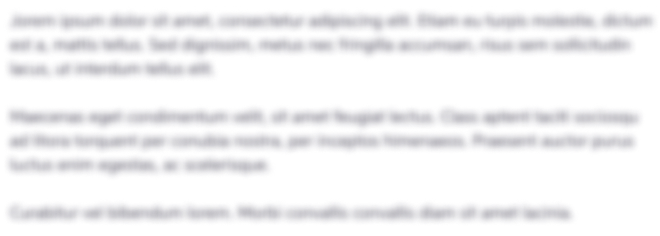
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started