Question
Could someone fix my code I keep getting 58 out of a 100 I wanna make sure I understand the material, I will rate highly.
Could someone fix my code I keep getting 58 out of a 100 I wanna make sure I understand the material, I will rate highly.
Define the class bankAccount to implement the basic properties of a bank account. An object of this class should store the following data: Account holders name (string), account number (int), account type (string, checking/saving), balance (double), and interest rate (double). (Store interest rate as a decimal number.) Add appropriate member functions to manipulate an object. Use a static member in the class to automatically assign account numbers. Also, declare an array of 10 components of type bankAccount to process up to 10 customers and write a program to illustrate how to use your class.
Example output is shown below:
1: Enter 1 to add a new customer. 2: Enter 2 for an existing customer. 3: Enter 3 to print customers data. 9: Enter 9 to exit the program. 1 Enter customer's name: Dave Brown Enter account type (checking/savings): checking Enter amount to be deposited to open account: 10000 Enter interest rate (as a percent): .01 1: Enter 1 to add a new customer. 2: Enter 2 for an existing customer. 3: Enter 3 to print customers data. 9: Enter 9 to exit the program. 3 Account Holder Name: Dave Brown Account Type: checking Account Number: 1100 Balance: $10000.00 Interest Rate: 0.01% ***************************** 1: Enter 1 to add a new customer. 2: Enter 2 for an existing customer. 3: Enter 3 to print customers data. 9: Enter 9 to exit the program. 9
Since your program handles currency, make sure to use a data type that can store decimals with a precision to 2 decimals.
bankAccount.h
#ifndef BANK_ACCOUNT_H
#define BANK_ACCOUNT_H
#include
#include
using namespace std;
class bankAccount
{
private:
string accountHolderName;//Account holder name
int accountNumber;//Account number
string accountType;//Account type(savings or checking)
double accountBalance;//Account balance
double interestRate;//Interest rate
static int n;
public:
//Constructor
bankAccount()
{
n++;
accountNumber = 10000 + n;//Assign account number
}
bankAccount(string name, string type, double balance, double rate) : accountHolderName(name), accountType(type), accountBalance(balance), interestRate(rate)
{
n++;
accountNumber = 10000. + n;//Assign account number
}
void deposit(double amount);
void withdraw(double amount);
double getInterest();
void updateBalance();
void print();
int getAccountNumber();
string getAccountHolderName();
string getAccountType();
double getBalance();
double getInterestRate();
void setAccountHolderName(const string &accountHolderName);
void setAccountType(const string &accountType);
void setAccountBalance(double accountBalance);
void setInterestRate(double interestRate);
};
bankAccountImp.cpp
#endif
#include "bankAccount.h"
#include
#include
using namespace std;
int bankAccount::n = 0;//Initialize static member n
//Method to perform deposit
void bankAccount::deposit(double amount)
{
accountBalance += amount;//Deposit
}
//Method to perform withdrawal
void bankAccount::withdraw(double amount)
{
if(accountBalance - amount < 0)//Check for sufficient balance
{
cout<<"Withdrawal failed: Insufficient balance"< return; } accountBalance -= amount;//Make withdrawal } //Method to return balance time interest double bankAccount::getInterest() { return (accountBalance * interestRate); } //Method to add interest to balance void bankAccount::updateBalance() { accountBalance += getInterest(); } //Method to print all account information void bankAccount::print() { cout << "Account Holder Name: " << accountHolderName << endl; cout << "Account Type: " << accountType << endl; cout << "Account number: " << accountNumber << endl; cout << "Balance: $" << accountBalance << endl; cout << "Interest Rate: " << interestRate << "%" << endl; cout << "**************" << endl; } //Method to get account number int bankAccount::getAccountNumber() { return accountNumber; } //Method to get account holder name string bankAccount::getAccountHolderName() { return accountHolderName; } //Method to get account type string bankAccount::getAccountType() { return accountType; } //Method to get balance double bankAccount::getBalance() { return accountBalance; } //Method to get interest rate double bankAccount::getInterestRate() { return interestRate; } void bankAccount::setAccountHolderName(const string &accountHolderName) { bankAccount::accountHolderName = accountHolderName; } void bankAccount::setAccountType(const string &accountType) { bankAccount::accountType = accountType; } void bankAccount::setAccountBalance(double accountBalance) { bankAccount::accountBalance = accountBalance; } void bankAccount::setInterestRate(double interestRate) { bankAccount::interestRate = interestRate; } main.cpp #include #include #include "bankAccount.h" using namespace std; void printMenu() { cout << "1: Enter 1 to add a new customer." << endl; cout << "2: Enter 2 for an existing customer." << endl; cout << "3: Enter 3 to print customers data." << endl; cout << "9: Enter 9 to exit the program." << endl; } int main() { bankAccount acc[10]; int option, size = 0; do{ printMenu(); cout << "Enter your choice: "; cin >> option; cin.ignore(255, ' '); switch(option) { case 1: { if(size == 10) { cout << "Array full. Cannot add more customers." << endl; break; } string name, type; double amount, interest; cout << "Enter customer's name: "; getline(cin, name); cout << "Enter account type(checking/saving): "; cin >> type; cout << "Enter amount to deposit to open account: $"; cin >> amount; cout << "Enter interest rate(as a percent): "; cin >> interest; acc[size].setAccountHolderName(name); acc[size].setAccountType(type); acc[size].setAccountBalance(amount); acc[size].setInterestRate(interest); size++; break; } case 2: { int accountNum; bool found = false; cout << "Enter account number: "; cin >> accountNum; for(int i = 0; i < size; i++) { if(acc[i].getAccountNumber() == accountNum) { int choice; double amount; cout << "1: Deposit" << endl; cout << "2: Withdrawal" << endl; cin >> choice; if(choice == 1) { cout << "Enter amount: $"; cin >> amount; acc[i].deposit(amount); } else if(choice == 2) { cout << "Enter amount: $"; cin >> amount; acc[i].withdraw(amount); } else { cout << "Invalid option." << endl; } found = true; break; } } if(!found) cout << "Account not found" << endl; break; } case 3: { for(int i = 0; i < size; i++) { acc[i].print(); cout << endl; } break; } case 9: { break; } default: cout << "Invalid option." << endl; } cout << endl; }while(option != 9); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
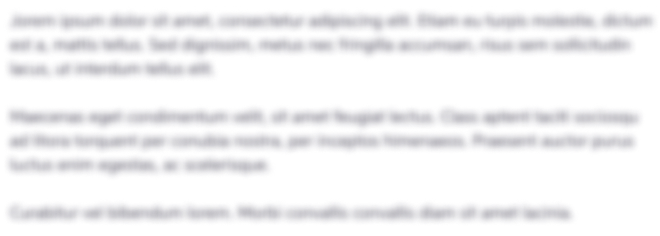
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started