Question
could you check my code? BC my teacher said I have to call a method (displayInfo() sections) but he said he don't tell me why(he
could you check my code? BC my teacher said I have to call a method (displayInfo() sections) but he said he don't tell me why(he pointed out the the area of every displayInfo() So i really don't know where should we edit). I really don't understand what I miss. ALSO I hope you can edit my code and feedback to me.
direction is this.
"
- An enumeration Month class consists of month constants from Jan to Dec.
- A name class has a first name and a last name; i.e., are the two instance variables of this class
- A Date class. Note that, other than the usual information for a calendar date i.e., private instance variables the day, the month (enum type), and the year; you have constructors, accessor and mutator methods. For the default constructor, initialize the date to 1st January 2023.
- A Height class has the same features as the Date class (constructors, accessor and mutator methods). Two private instance variables feet and inches. One important method is to convert the feet and inches and returns its equivalent value in meter.
A HealthProfile class has the same features as the Date class (constructors, accessor and mutator methods). Some important instance variables that you will see later in some UML diagram. You include also a few important methods related to the class; for example, get the maximum heart rate method, get the 3 minimum and the maximum of the targeted heart rate; compute and return the BMI; and a method to print out an object of this class."
Put all the classes in a Java file named it as YourName_A2.java (remove the word public from all classes)
(what is that mean? should I change all Public to private from my classes?)
input.txt should be like this
final output should be this
I did all UML in my code. Please help me to solve this code.
-----------------------------
import java.util.Scanner;
import java.io.File;
import java.io.IOException;
enum Month {Jan, Feb, Mar, Apr, May, Jun, Jul, Aug, Sep, Oct, Nov, Dec};
class Date{
private int day;
private Month month;
private int year;
//default constructor
public Date()
{
this.day = 1;
this.month = Month.Jan;
this.year = 2023;
}
//other constructor
public Date (int day, Month month, int year)
{
this.day = day;
this.month = month;
this.year =year;
}
//copy constructor
public Date(Date d)
{
this(d.day, d.month, d.year);
}
// Accessor methods
public int getDay()
{
return day;
}
public Month getMonth()
{
return month;
}
public int getYear()
{
return year;
}
//mutator methods
public void setDate(int day, Month month, int year)
{
this.day = day;
this.month = month;
this.year = year;
}
}
class Height
{
private int feet;
private int inches;
//default constructor
public Height()
{
this.feet = feet;
this.inches = inches;
}
//other constructor
public Height(int feet, int inches)
{
this.feet = feet;
this.inches = inches;
}
//copy constructor
public Height(Height h)
{
this(h.feet, h.inches);
}
// Accessor methods
public int getFeet()
{
return feet;
}
public int inches()
{
return inches;
}
// Mutator method
public void setHeight(int feet, int inches)
{
this.feet = feet;
this.inches = inches;
}
// feet to meters
public double getMeters()
{
double meters = feet * 0.3048;
return meters;
}
// Convert inches to meters
public double getCentimeters()
{
double centimeters = inches * 0.0254;
return centimeters;
}
public double getHeightInMeter()
{
double heightinmeter = getMeters() + getCentimeters();
return heightinmeter;
}
}
class Name {
private String firstName;
private String lastName;
public Name(String firstName, String lastName)
{
this.firstName = firstName;
this.lastName = lastName;
}
public Name(Name n)
{
this(n.firstName, n.lastName);
}
// Accessor methods
public String getFirstName()
{
return firstName;
}
public String getLastName()
{
return lastName;
}
public void setName(String firstName, String lastName)
{
this.firstName = firstName;
this.lastName = lastName;
}
public void displayInfo()
{
System.out.printf("%nName: %s, %s%n", firstName, lastName);
//System.out.printf("Date of birth: %d %s %d %n" );
}
}
class HealthProfile{
private Name name;
private Date dob;
private Height h;
private double weight;
private int currentYear;
//constuctor
public HealthProfile(Name name, Date dob, Height h, double weight, int currentYear)
{
this.name = name;
this.dob = dob;
this.h = h;
}
public HealthProfile(HealthProfile hr)
{
this(hr.name, hr.dob, hr.h, hr.weight, hr.currentYear);
}
public Name getName()
{
return hr.name;
}
public void setName(Name name)
{
this.name = name;
}
public Date getDOB()
{
return hr.dob;
}
public Height getHeight()
{
return hr.h;
}
public double getweight()
{
return hr.weight;
}
public void setDOB(Date dob)
{
this.dob = dob;
}
public void setHeight(Height h)
{
this.h = h;
}
public int getCurrentYear()
{
return hr.currentYear;
}
public void setCurrentYear(int currentYear)
{
this.currentYear = currentYear;
}
public int getAge()
{
int age = currentYear - dob.getYear();
return age;
}
public int getMaximumHeartRate()
{
int maxHR = 220 - getAge;
return maxHR;
}
public doube getMinimumTargetHeartRate()
{
double minTHR = getMaximumHeartRate()*0.5;
return minTHR;
}
public double getMaximumTargetHeartRate()
{
double maxTHR = getMaximumHeartRate() * 0.85;
return maxTHR;
}
public double getBMI()
{
double bmi = weight / (h.getHeightInMeter() *h.getHeightInMeter());
return bmi;
}
public void displayInfo()
{
}
}
class ABC
{
public static void main (String [] args) throws IOException
{
// Construct a Scanner class object, link to data file called input.txt
Scanner input = new Scanner (new File("/Users/"myname"/Desktop/"folder name"/input.txt"));
// Declare private variables
Name firstName;
Name lastName;
Date dob = new Date();
Height h = new Height();
double weight;
int currentYear;
firstName = input.nextLine();
lastName = input.nextLine();
dob.setDate(input.nextInt, Month.valueOf(input.next()), input.nextInt());
h.setHeight(input.nextInt(), input.nextInt());
weight = input.nextDouble();
currentYear = input.nextInt();
input.nextLine();
// Construct a HealthProfile class object
HealthProfile hr = new HealthProfile (firstName, lastName, dob, h, weight, currentYear);
// Display patient 1 info
System.out.printf("First patient%n");
System.out.println("--------------");
hr.printInfo();
// patient 2 info from file
firstName = input.nextLine();
lastName = input.nextLine();
dob.setDate(input.nextInt(), Month.valueOf(input.next()), input.nextInt());
h.setHeight(input.nextInt(), input.nextInt());
weight = input.nextDouble();
currentYear = input.nextInt();
HealthProfile hr2 = new HealthProfile(firstName, lastName, dob, h, weight, currentYear);
// Display patient 2 info
System.out.printf("Second patient%n");
System.out.println("--------------");
hr2.printInfo();
// Display patient 2 info
System.out.printf("%nSecond patient%n");
System.out.println("--------------");
hr1.printInfo();
}
}
Einget-NotepadMohamedAliBinAbdullah15Oct19515663.52022AlanRichardHeng31Dec20056898.82022 Name: Mohamed Ali, Bin Abdullah Date of birth: 15 Oct 1951 Your weight: 63.5kg Your height: 5 feet 6 inches; equivalent to: 1.68 meter Current year: 2022 Your age: 71 years old Clinic analysis, base on your age: 1. Your maximum heart rate is 149 2. Your minimum target heart rate is 74.50 3. Your maximum target heart rate is 126.65 Your BMI 22.6 Weight category Range Underweight / too low Below 18.5 Healthy range 18.525 Overweight 2530 Obese 3035 Severe Obesity 3540 Morbid Obesity Over 40Step by Step Solution
There are 3 Steps involved in it
Step: 1
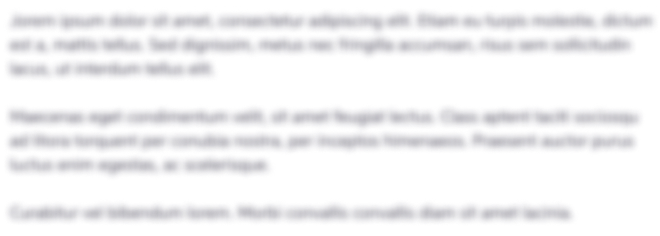
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started