Question
Could you help me with this lab on arraylist for Java? Thanks I have to implement the methods in two different data structure classes. package
Could you help me with this lab on arraylist for Java? Thanks
I have to implement the methods in two different data structure classes.
package studentCode;
import java.util.ArrayList;
public class MyPersonListV1 {
//DO NOT CHANGE THIS LINE
ArrayList
/**
* Instantiates the "people" variable as a new ArrayList of
* references to Person objects.
*/
public MyPersonListV1(){
throw new RuntimeException("You need to implement this...");
}
/**
* A copy constructor which makes the right kind of copy considering
* a Person is mutable.
*/
public MyPersonListV1(MyPersonListV1 other) {
throw new RuntimeException("You need to implement this...");
}
/** Adds the parameter to the end of the list. With ArrayList this
* will be much shorter in terms of code than you will see in the
* V2 approach using arrays. Of course here, we have no control
* over how much "spare" space is allocated in the interest of
* time efficiency behind the scenes.
*
* Note that you want to make sure that you don't just do a reference
* copy of the newMember Person since it is mutable.
*/
public void addItem(Person newMember){
throw new RuntimeException("You need to implement this...");
}
/** Gives each person in the list a raise of $1000. Note that the objects
* are mutable, which means you can use a for-each style loop as you update.
*/
public void giveRaises(){
throw new RuntimeException("You need to implement this...");
}
/** Returns the sum of the salaries of all people in the list.
*/
public int getTotalOfSalaries(){
throw new RuntimeException("You need to implement this...");
}
/** Returns the number of people in the list with a name
* that matches the parameter (possibly 0).
*/
/**
* @param searchName
* @return
*/
public int count(String searchName){
throw new RuntimeException("You need to implement this...");
}
/** Removes ALL people from the list whose names match the parameter
* (possibly more than one person). Consider that a for-each style
* loop cannot be used unless you create a shadow. There are code
* examples in the posted slides that will be useful here.
*/
public void remove(String name){
throw new RuntimeException("You need to implement this...");
}
}
package studentCode;
public class MyPersonListV2 {
//DO NOT CHANGE THIS LINE OF CODE
Person[] people;
/**
* Instantiates the "people" variable as a new (0-length) array of
* references to Person objects.
*/
public MyPersonListV2(){
throw new RuntimeException("You need to implement this...");
}
/**
* A copy constructor which makes the right kind of copy considering
* a Person is mutable.
*/
public MyPersonListV2(MyPersonListV2 other) {
throw new RuntimeException("You need to implement this...");
}
/** Adds the parameter to the end of the list. You'll need to
* create a new array that's one space longer and you'll need
* to copy everything from the old array into the new one, and
* then add the new one of course.
*
* Note that you want to make sure that you don't just do a reference
* copy of the Person since it is mutable.
*/
public void addItem(Person newMember){
throw new RuntimeException("You need to implement this...");
}
/** Gives each person in the list a raise of $1000
*/
public void giveRaises(){
throw new RuntimeException("You need to implement this...");
}
/** Returns the sum of the salaries of all people in the list.
*/
public int getTotalOfSalaries(){
throw new RuntimeException("You need to implement this...");
}
/** Returns the number of people in the list with a name
* that matches the parameter (possibly 0).
*/
/**
* @param searchName
* @return
*/
public int count(String searchName){
throw new RuntimeException("You need to implement this...");
}
/** Removes ALL people from the list whose names match the parameter
* (possibly more than one person). This should "shrink" the array
* if anyone is removed. That means you need to first find out how
* many times the name appears, then allocate a new smaller array
* of the right size, and then only copy the things that don't match
* the name from the old array into the new one.
*/
public void remove(String name){
throw new RuntimeException("You need to implement this...");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
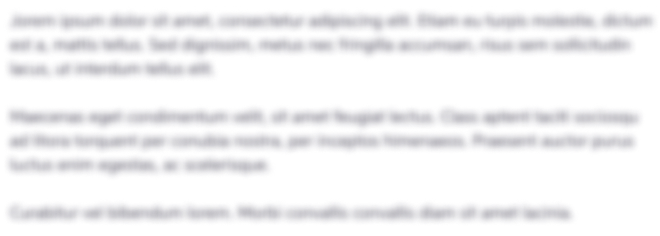
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started