Question
Could you please help me on this JavaScript program. This program works well, but I want to get the order list that I get in
Could you please help me on this JavaScript program. This program works well, but I want to get the order list that I get in preview when I click the order button also. Also, I would like to have a way to click in the final order list to go to new order again. Please help me, I really appreciate your help.
Index.html file
Nilanthi Pizza Palace
Pizza Order Form
Order Summary
Deliver to
Order
 Calculator
Calculator.html File:
Click go Back to Pizza Order From
Name
Script.js File:
//script.js
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
var box = document.getElementById('display');
function toScreen(x){
box.value+=x;
if (x==='c'){
box.value = '';
}
}
function answer(){
x = box.value
x=eval(x);
box.value=x;
}
function power(){
x=box.value;
x=eval(x*x);
box.value=x;
}
function backspace(){
var num = box.value;
var len=num.length-1;
var newNum=num.substring(0,len);
box.value = newNum;
}
Script2.js File:
/*
Programing Language: JavaScript
* Final Project: SEDV 250
* Author: Nilanthi Abeygunawardana
* Date: 04/14/18
Presented to: Prof. Scott Withrow
Ivy Tech Community Collage
* Filename: script.js
*/
"use strict"; // interpret document contents in JavaScript strict mode
/* global variable */
var formValidity = true;
var delivInfo = {};
var foodInfo = {};
var delivSummary = document.getElementById("deliverTo");
var foodSummary = document.getElementById("order");
function previewOrder() {
processDeliveryInfo();
processFood();
document.getElementsByTagName("section")[0].style.display = "block";
}
function processDeliveryInfo() {
var prop;
delivInfo.name = document.getElementById("nameinput").value;
delivInfo.addr = document.getElementById("addrinput").value;
delivInfo.city = document.getElementById("cityinput").value;
delivInfo.email = document.getElementById("emailinput").value;
delivInfo.phone = document.getElementById("phoneinput").value;
for (prop in delivInfo) {
delivSummary.innerHTML += "
" + delivInfo[prop] + "
";}
document.getElementById("deliverTo").style.display = "block";
}
function processFood() {
var prop;
var crustOpt = document.getElementsByName("crust");
var toppings = 0;
var toppingBoxes = document.getElementsByName("toppings");
var instr = document.getElementById("instructions");
if (crustOpt[0].checked) {
foodInfo.crust = crustOpt[0].value;
} else {
foodInfo.crust = crustOpt[1].value;
}
foodInfo.size = document.getElementById("size").value;
for (var i = 0; i < toppingBoxes.length; i++) {
if (toppingBoxes[i].checked) {
toppings++;
foodInfo["topping" + toppings] = toppingBoxes[i].value;
}
}
if (instr.value !== "") {
foodInfo.instructions = instr.value;
}
foodSummary.innerHTML += "
Crust: " + foodInfo.crust + "
";foodSummary.innerHTML += "
Size: " + foodInfo.size + "
";foodSummary.innerHTML += "
Topping(s): " + "
";foodSummary.innerHTML += "
- ";
- " + foodInfo["topping" + i] + " ";
for (var i = 1; i < 6; i++) {
if (foodInfo["topping" + i]) {
foodSummary.innerHTML += "
}
}
foodSummary.innerHTML += "
foodSummary.innerHTML += "
Instructions: " + foodInfo.instructions;
document.getElementById("order").style.display = "block";
}
/* create event listener */
function createEventListener() {
var previewBtn = document.getElementById("previewBtn");
if (previewBtn.addEventListener) {
previewBtn.addEventListener("click", previewOrder, false);
} else if (previewBtn.attachEvent) {
previewBtn.attachEvent("onclick", previewOrder);
}
}
/* create event listener when page finishes loading */
if (window.addEventListener) {
window.addEventListener("load", createEventListener, false);
} else if (window.attachEvent) {
window.attachEvent("onload", createEventListener);
}
/* remove default value and formatting from selection list */
function removeSelectDefault() {
var selectBox = document.getElementById("size");
selectBox.selectedIndex = -1;
selectBox.style.boxShadow = "none";
}
/* remove fallback placeholder text */
function zeroPlaceholder() {
var instrBox = document.getElementById("instructions");
instrBox.style.color = "black";
if (instrBox.value === instrBox.placeholder) {
instrBox.value = "";
}
}
/* restore placeholder text if box contains no user entry */
function checkPlaceholder() {
var instrBox = document.getElementById("instructions");
if (instrBox.value === "") {
instrBox.style.color = "rgb(178,184,183)";
instrBox.value = instrBox.placeholder;
}
}
/* add placeholder text for browsers that don't support placeholder attribute */
function generatePlaceholder() {
if (!Modernizr.input.placeholder) {
var instrBox = document.getElementById("instructions");
instrBox.value = instrBox.placeholder;
instrBox.style.color = "rgb(178,184,183)";
if (instrBox.addEventListener) {
instrBox.addEventListener("focus", zeroPlaceholder, false);
instrBox.addEventListener("blur", checkPlaceholder, false);
} else if (instrBox.attachEvent) {
instrBox.attachEvent("onfocus", zeroPlaceholder);
instrBox.attachEvent("onblur", checkPlaceholder);
}
}
}
/* validate required fields */
function validateRequired() {
var inputElements = document.querySelectorAll("input[required]");
var errorDiv = document.getElementById("errorMessage");
var crustBoxes = document.getElementsByName("crust");
var fieldsetValidity = true;
var elementCount = inputElements.length;
var currentElement;
try {
for (var i = 0; i < elementCount; i++) {
// validate all required input elements in fieldset
currentElement = inputElements[i];
if (currentElement.value === "") {
currentElement.style.background = "rgb(255,233,233)";
fieldsetValidity = false;
} else {
currentElement.style.background = "white";
}
}
currentElement = document.querySelectorAll("select")[0];
// validate state select element
if (currentElement.selectedIndex === -1) {
currentElement.style.border = "1px solid red";
fieldsetValidity = false;
} else {
currentElement.style.border = "";
}
if (!crustBoxes[0].checked && !crustBoxes[1].checked) {
// verify that a crust is selected
crustBoxes[0].style.outline = "1px solid red";
crustBoxes[1].style.outline = "1px solid red";
fieldsetValidity = false;
} else {
crustBoxes[0].style.outline = "";
crustBoxes[1].style.outline = "";
}
if (fieldsetValidity === false) {
throw "Please complete all required fields.";
} else {
errorDiv.style.display = "none";
errorDiv.innerHTML = "";
}
}
catch(msg) {
errorDiv.style.display = "block";
errorDiv.innerHTML = msg;
formValidity = false;
}
}
/* validate form */
function validateForm(evt) {
if (evt.preventDefault) {
evt.preventDefault(); // prevent form from submitting
} else {
evt.returnValue = false; // prevent form from submitting in IE8
}
formValidity = true; // reset value for revalidation
validateRequired();
if (formValidity === true) {
document.getElementById("errorMessage").innerHTML = "";
document.getElementById("errorMessage").style.display = "none";
document.getElementsByTagName("form")[0].submit();
} else {
document.getElementById("errorMessage").innerHTML = "Please complete the highlighted fields.";
document.getElementById("errorMessage").style.display = "block";
scroll(0,0);
}
}
/* create event listeners */
function createEventListeners() {
var orderForm = document.getElementsByTagName("form")[0];
if (orderForm.addEventListener) {
orderForm.addEventListener("submit", validateForm, false);
} else if (orderForm.attachEvent) {
orderForm.attachEvent("onsubmit", validateForm);
}
}
/* run initial form configuration functions */
function setUpPage() {
removeSelectDefault();
createEventListeners();
generatePlaceholder();
}
/* run setup functions when page finishes loading */
if (window.addEventListener) {
window.addEventListener("load", setUpPage, false);
} else if (window.attachEvent) {
window.attachEvent("onload", setUpPage);
}
Styles.css File:
/*
Programing Language: JavaScript
Final Project: SEDV 250
Author: Nilanthi Aeygunawardana
Date: 04/14/18
Presented to: Prof. Scott Withrow
Ivy Tech Community Collage
Filename: styles.css
*/
/* apply a natural box layout model to all elements */
* {
-moz-box-sizing: border-box;
-webkit-box-sizing: border-box;
box-sizing: border-box;
}
/* reset rules */
html, body, div, span, applet, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
a, abbr, acronym, address, big, cite, code,
del, dfn, em, img, ins, kbd, q, s, samp,
small, strike, strong, sub, sup, tt, var,
b, u, i, center,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, embed,
figure, figcaption, footer, header, hgroup,
menu, nav, output, ruby, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
font-size: 100%;
font: inherit;
vertical-align: baseline;
}
/* HTML5 display-role reset for older browsers */
article, aside, details, figcaption, figure,
footer, header, hgroup, menu, nav, section {
display: block;
}
body {
line-height: 1;
max-width: 960px;
background: white;
margin: 0 auto;
font-family: Arial, Helvetica, sans-serif;
-webkit-box-shadow: 10px 0px 10px rgba(50, 50, 50, 1),
0px 10px 10px rgba(50, 50, 50, 1),
-10px 0px 10px rgba(50, 50, 50, 1);
-moz-box-shadow: 10px 0px 10px rgba(50, 50, 50, 1),
0px 10px 10px rgba(50, 50, 50, 1),
-10px 0px 10px rgba(50, 50, 50, 1);
box-shadow: 10px 0px 10px rgba(50, 50, 50, 1),
0px 10px 10px rgba(50, 50, 50, 1),
-10px 0px 10px rgba(50, 50, 50, 1);
}
ol, ul {
list-style: none;
}
/* page header */
header {
background: #04819E;
width: 100%;
color: #FFFFFF;
font-size: 48px;
text-align: center;
line-height: 1.5em;
border-bottom: 1px solid black;
}
/* main content */
article {
width: 960px;
text-align: left;
background: ivory;
padding: 20px;
overflow: auto;
}
h2 {
font-weight: bold;
font-size: 30px;
text-align: center;
width: 66.6667%;
}
#errorMessage {
display: none;
width: 80%;
text-align: center;
padding: 10px;
margin: 15px auto 5px;
background: #e3d5ba;
color: black;
border: 5px solid rgb(190,50,38);
font-weight: bold;
}
/* form */
form {
padding: 10px;
width: 66.6667%;
float: left;
}
fieldset {
margin-bottom: 10px;
position: relative;
padding: 2.5em 1em 0.5em 1em;
background: #e3d5ba;
}
fieldset fieldset {
padding-top: 15px;
}
legend, #submit, #instrlabel {
font-family: arial, helvetica, sans-serif;
font-weight: bold;
}
legend {
position: absolute;
top: 0;
left: 0;
font-size: 1.25em;
margin-top: 0.5em;
margin-left: 0.5em;
}
fieldset fieldset legend {
font-size: 1em;
margin-top: 25px;
top: -30px;
left: 8px;
}
input, textarea {
font-size: 1em;
}
#instructions {
display: block;
}
#deliveryinfo, #orderinfo, #additionalinfo, #submitbutton {
border: none;
}
#deliveryinfo label {
display: block;
float: left;
clear: left;
margin-top: 5px;
}
#deliveryinfo input {
display: block;
margin-left: 135px;
}
#nameinput, #emailinput, #addrinput {
width: 20em;
}
#cityinput, #phoneinput {
width: 12em;
}
#crustbox label, #toppingbox label {
margin-right: 25px;
}
#instrlabel {
display: block;
margin: 1em 0 0.5em 0;
}
textarea {
margin-bottom: 1em;
}
#submitBtn {
font-size: 1.25em;
}
#submitbutton {
background: inherit;
border: none;
padding: 0.5em 0 0 0;
text-align: center;
}
#previewBtn {
font-size: 1.25em;
}
#previewbutton {
background: inherit;
border: none;
padding: 0.5em 0 0 0;
text-align: center;
}
section {
float: right;
width: 33.3333%;
background: white;
border: 3px solid #04819E;
margin-top: 10px;
display: none;
}
section h2 {
width: 100%;
background: #e3d5ba;
padding: 5px;
}
section div {
margin-left: 20px;
display: none;
padding: 10px;
}
section div p {
margin-bottom: 5px;
}
section div p span {
font-weight: bold;
}
section li {
list-style-type: none;
margin-left: 20px;
}
h3 {
font-family: arial, helvetica, sans-serif;
font-weight: bold;
font-size: 1.25em;
margin: 0.5em 0 0.5em -0.5em;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
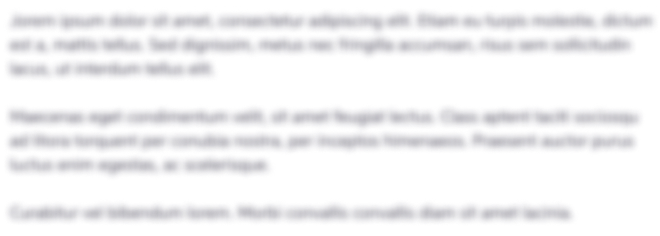
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started