Question
Could you recode this Ceasar cipher from LC-3 to Mips (Mars) assembly code? Thank you ;the ceasar cipher asks user for encrypt or decrypt ;it
Could you recode this Ceasar cipher from LC-3 to Mips (Mars) assembly code? Thank you
;the ceasar cipher asks user for encrypt or decrypt
;it will encrypt or decrypt user input string by shifting
;by a user chosen number
; main code
.ORIG x3000
; greeting
LD R0, greetingAddr
PUTS
greetingAddr .FILL greeting
;clear all registers
Start AND R0, R0, 0
STI R0, cryptAddr
STI R0, keyAddr
AND R0, R0, 0
AND R1, R0, 0
AND R2, R0, 0
AND R3, R0, 0
AND R4, R0, 0
AND R5, R0, 0
AND R6, R0, 0
AND R7, R0, 0
cryptAddr .FILL crypt
keyAddr .FILL key
; ask user to choose encrypt, decrypt, or exit
LD R0, askCryptAddr
PUTS ; print prompt string
LD R0, newlineAddr ; new line
PUTS
Loop1 GETC
OUT
; check if user wants to exit
LD R1, negOfx
ADD R1, R0, R1 ; check if hit x
BRz EndProgram
; check if user wants to encrypt
LD R1, negOfe
ADD R1, R0, R1 ; check if e
BRz SetToEncrypt
; check if user wants to decrypt
LD R1, negOfd
ADD R1, R0, R1 ; check if d
BRz SetToDecrypt
BR Loop1
SetToEncrypt AND R1, R1, 0 ; clear R1
ST R1, crypt ; set crypt to 0 (Encrypt)
BR InputCipherKey
SetToDecrypt AND R1, R1, 0 ; clear R1
ADD R1, R1, #1 ; add 1
ST R1, crypt ; set crypt to 1 (Decrypt)
BR InputCipherKey ; next
askCryptAddr .FILL askCrypt ; indirect
newlineAddr .FILL newline
; ask user to input cipher shift key (the value to shift by, between 1-25)
InputCipherKey LD R0, askShiftAddr
PUTS
LEA R0, newline ; load newline with > symbol
PUTS
JSR NumberInput ; outputs number input to R1
ST R0, key ; store number input into key
BR InputString ; next
askShiftAddr .FILL askShiftkey
; ask user for the string
InputString LD R0, askStrAddr
PUTS
LEA R0, newline
PUTS
AND R1, R1, 0 ; R1 is column
AND R2, R2, 0 ; R2 is row
Loop2 GETC
ADD R4, R0, #-10 ; negative of line feed
BRz Null1 ; if user hit Enter, go to null
OUT
JSR Store ; Input: R0 data, R1 row, R2 column
ADD R1, R1, #1 ; go to next column
BR Loop2
Null1 LD R0, nullChar
JSR Store ; already at next space
BR CheckCrypt ; next
askStrAddr .FILL askString
; Encrypt or decrypt the string
CheckCrypt LD R0, crypt ; check encrypt or decrypt
BRz LetsEncrypt ; if crypt=0 then encrypt
BRp LetsDecrypt ; if crypt=1 then decrypt
LetsEncrypt JSR Encrypt ; encrypt the input
BR LetsPrint
LetsDecrypt JSR Decrypt ; decrypt it
LetsPrint BR PrintString
; Print the string
PrintString JSR PrintArray
BR Start ; loop to restart
EndProgram LD R0, byeExtra ; End program
PUTS
HALT
byeExtra .FILL bye
;end of main program code
;@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@
; SUBROUTINES START HERE
; NumberInput
; this subroutine converts ASCII to decimal
; if user input a number, console will print the number back out
NumberInput ST R7, saveR7_1 ; Save R7 since TRAPS overwrite it
LD R4, asciiToDec
AND R0, R0, 0
ST R0, NumberIn
InLoopSR1 GETC
ADD R1, R0, #-10 ; check if user hit Enter or line feed
BRz DoneInputValue ; if user hit Enter, end input value
OUT
ADD R0, R0, R4 ; convert ascii input to decimal value
LD R1, NumberIn ; load user input value
; multiply by ten
AND R3, R3, 0 ; R3 used to store
ADD R2, R2, #10 ; set counter to 10 to multiply 10 times
Mult BRz EndMult
ADD R3, R3, R1 ; do multiplcation
ADD R2, R2, #-1 ; decrease the counter
BR Mult
EndMult ADD R1, R3, R0 ; add user value input + entered char decimal value
ST R1, NumberIn
BR InLoopSR1
DoneInputValue LD R7, saveR7_1 ; load R7 with correct return address
LD R0, NumberIn ; load user input to return
RET
NumberIn: .FILL 0
saveR7_1: .FILL 0
; Encrypt
; Input: None
; Output: None
Encrypt ST R7, saveR7_3
; calculate load address
AND R1, R1, 0 ; R1 is column
AND R2, R2, 0 ; R2 is row
ST R1, CountColmn ; set column count to 0
EncryptLoop AND R2, R2, 0 ; go to row 0
LD R1, CountColmn ; go to correct column
JSR Load ; R0 is data output
ADD R0, R0, 0 ; will be 0 if char is null
BRz NullSR1 ;
LD R1, key ; load key
JSR PerformEncrypt ; encrypt the char
; calculate store address
AND R2, R2, 0
ADD R2, R2, #1 ; go to row 1
LD R1, CountColmn ; go to correct column
JSR Store ; store data in (row 1, CountColmn)
;this stores encrypted characters into last row
LD R1, CountColmn ; load to iterate column
ADD R1, R1, #1 ; go to next column over
ST R1, CountColmn ; store column counter
BR EncryptLoop
NullSR1 LD R0, nullChar
AND R2, R2, 0 ; R1 is row
ADD R2, R2, #1 ; go to row 1
JSR Store ; column R1 already at next space
LD R7, saveR7_3
RET
CountColmn .FILL 0 ; counts the columns of each row
saveR7_3: .FILL 0 ; save R7 for return
; Decrypt
; Input: None
; Output: None
Decrypt ST R7, saveR7_4 ;save R7
AND R1, R1, 0 ; R1 is row
AND R2, R2, 0 ; R2 is column
ST R1, CountColmn2 ; set column count to 0
DecryptLoop AND R2, R2, 0 ; go to row 0
LD R1, CountColmn2 ; go to correct column
JSR Load ; R0 is data output
ADD R0, R0, 0 ; will be 0 if char is null
BRz NullSR2
LD R1, key ; load the key
JSR PerformDecrypt ; encrypt the char
; calculate store address
AND R2, R2, 0
ADD R2, R2, #1 ; go to row 1
LD R1, CountColmn2 ; go to correct column
JSR Store ; store data in (row 1, colmnCount2)
LD R1, CountColmn2 ; load to move column
ADD R1, R1, #1 ; go to next column over
ST R1, CountColmn2 ; store column counter
BR DecryptLoop
NullSR2 LD R0, nullChar
AND R2, R2, 0 ; R2 is row
ADD R2, R2, #1 ; go to row 1
JSR Store ; column R1 already at next space
LD R7, saveR7_4
RET
CountColmn2 .FILL 0 ; iterator count for moving columns
saveR7_4: .FILL 0 ; save R7 for return
; Perform the actual encryption
; Input: R0, the char. R1, the shift key
; Output: R0, the encrypted char.
PerformEncrypt
; first test the inputs
; test if input is uppercase
LD R2, negOfA
ADD R3, R0, R2
BRn FinEncrypt ; not abc char
LD R2, negOfZ
ADD R3, R0, R2
BRnz UppercaseEncrp
; test if input is lowercase
LD R2, negOfa
ADD R3, R0, R2
BRn FinEncrypt ; if negative, then input was not a abc character
LD R2, negOfz
ADD R3, R0, R2
BRnz LowercaseEncrp
BRp FinEncrypt
UppercaseEncrp ADD R0, R0, R1 ; perform encryption
LD R2, negOfZ
ADD R3, R0, R2
BRnz FinEncrypt ; no extra, so end
; add the extra if there is any
LD R4, A ; load uppercase A
ADD R0, R3, R4 ; add the extra difference to A
; then subtract 1 and end
ADD R0, R0, #-1 ; subtract 1 (ex: if there is extra of 1, then subtract it
; to loop back to original character)
BR FinEncrypt
LowercaseEncrp ADD R0, R0, R1 ; perform encryption
; check if went past lowercase z
LD R2, negOfz
ADD R3, R0, R2
BRnz FinEncrypt ; if less than or equal zero, then no extra, so end
; if so, subtract difference and add to a
LD R4, a ; load lowercase a
ADD R0, R3, R4 ; add the extra difference to a
ADD R0, R0, #-1 ; subtract 1
BR FinEncrypt
FinEncrypt RET
; Perform the actual decryption
; Input: R0, the char . R1, the shift key
; Output: R0, the decrypted char.
PerformDecrypt NOT R1, R1 ; perform 2's Complment
ADD R1, R1, #1 ; turn key into negative
; is char uppercase
LD R2, negOfA
ADD R3, R0, R2
BRn FinDecrypt ; not alphabetical char
LD R2, negOfZ
ADD R3, R0, R2
BRnz UppercaseDcryp
; is char lowercase
LD R2, negOfa
ADD R3, R0, R2
BRn FinDecrypt ; not alphabetical char
LD R2, negOfz
ADD R3, R0, R2
BRnz LowercaseDcryp
BRp FinDecrypt ; not alphabetical char
UppercaseDcryp ADD R0, R0, R1 ; perform decryption
; check if went below uppercase A
LD R2, negOfA
ADD R3, R0, R2
BRzp FinDecrypt ; if greater or equal to zero, then no extra, so end
; if so, use the difference and subtract from Z
LD R4, Z
ADD R0, R3, R4 ; subtract the extra from Z
; then add 1 and end
ADD R0, R0, #1 ; add 1 to the value
BR FinDecrypt
LowercaseDcryp ADD R0, R0, R1 ; perform decryption
; check if went below lowercase a
LD R2, negOfa
ADD R3, R0, R2
BRzp FinDecrypt ; if less than or equal, then no extra, so end
; if so, use the difference and subtract from z
LD R4, z ; load lowercase z
ADD R0, R3, R4 ; subtract the extra from z
ADD R0, R0, #1 ; add 1
BR FinDecrypt
FinDecrypt RET
a .FILL #97
negOfa .FILL #-97
z .FILL #122
negOfz .FILL #-122
A .FILL #65
negOfA .FILL #-65
Z .FILL #90
negOfZ .FILL #-90
; Store
; this stores data into CIPHER_ARRAY
; Input: R0, ASCII character to store. R1, the column. R2, the row
; Output: None
Store LD R3, CipherAddr
ADD R4, R2, #-1 ; check which row
BRn SkipSR1 ; if first row, then skip
LD R4, fifty ; R4 = 50
ADD R3, R3, R4 ; calculate the row
SkipSR1 ADD R3, R3, R1 ; calculate the column
STR R0, R3, #0 ; store
RET
; Load
; this subroutine loads data from CIPHER_ARRAY
; Input: R1, the column. R2, the row.
; Output: R0, contents of CIPHER_ARRAY
Load LD R3, CipherAddr
ADD R4, R2, #-1 ; check which row
BRn SkipSR2 ; if first row, then skip
LD R4, fifty ; fifty is # of columns in a row
ADD R3, R3, R4 ; calculate the row
SkipSR2 ADD R3, R3, R1 ; calculate the column
LDR R0, R3, #0 ; load data into R0
RET
CipherAddr .FILL CIPHER_ARRAY ; indirect
; PrintArray
; this subroutine prints out encrypted/decrpyed strings
PrintArray ST R7, saveR7_2 ; Save R7
LD R0, crypt
BRz PrintEncrypt ; if crypt=0, encrypt
PrintDecrypt LEA R0, encryptedStr
PUTS
LEA R0, CIPHER_ARRAY
PUTS
LEA R0, decryptedStr
PUTS
LEA R0, CIPHER_ARRAY
LD R1, fifty ; R1 = 50
ADD R0, R0, R1 ; go to next row (add 50)
PUTS
BR DonePrint
PrintEncrypt LEA R0, decryptedStr
PUTS
LEA R0, CIPHER_ARRAY
PUTS
LEA R0, encryptedStr
PUTS
LEA R0, CIPHER_ARRAY
LD R1, fifty ; R1 =50
ADD R0, R0, R1 ; go to next row (add 50)
PUTS ; print encrypted text
BR DonePrint
DonePrint LD R7, saveR7_2
RET
saveR7_2: .FILL 0 ; save R7
crypt: .FILL 0 ; 0 is encrypt; 1 is decrypt
key: .FILL 0
negOfx: .FILL -120 ; use to test if x entered
negOfd: .FILL -100 ; use to test of d entered
negOfe: .FILL -101 ; use to test if e entered
ascii: .FILL 48 ; decimal to ascii
asciiToDec: .FILL -48 ; ascii to decimal
nullChar: .FILL 0 ; counts as null char
fifty: .FILL 50 ; 2x50 array
newline: .STRINGZ " > "
greeting: .STRINGZ " Hi, welcome to my Caesar Cipher program"
askCrypt: .STRINGZ " Do you want to (e) ncrypt, (d)ecrypt, or e(x)it?"
askShiftkey: .STRINGZ " What's the cipher shift key (1-25)?"
askString: .STRINGZ " What's the string? (up to 50 chars)"
bye: .STRINGZ " See Ya!"
encryptedStr: .STRINGZ " Encrypted: "
decryptedStr: .STRINGZ " Decrypted: "
CIPHER_ARRAY .BLKW 100 ; 2D array, row major
.END
Step by Step Solution
There are 3 Steps involved in it
Step: 1
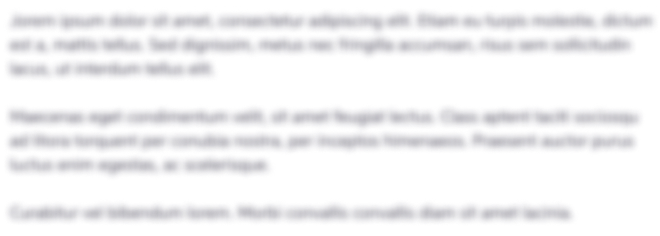
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started