Question
Could you write a C++ code for this task? Ive provided the skeletal implementation and requirements in the following. In case you dont know what
Could you write a C++ code for this task?
Ive provided the skeletal implementation and requirements in the following. In case you dont know what school method addition is, the contents of a picture will help you.
Actually, you are only allowed to write the implementations for the two functions Integer add() and Integer kuml() . For Integer add() it may need 'for loop' to implement and Integer kuml() needs recursion??(Its just my thought.)
Plz make sure your code could compile on linux or MAC(I usually use Xcode) by using g++ compiler.
main.cpp
#include
#include
#include "typedef.h" //Type definitions
#include "operations.h"
#include "utils.h"
using namespace std;
// The main method parses the arguments and check for correctness. Then calls sum and kmul method with the input values.
// Finally, the return values are displayed on the command-line.
int main(int nargs, char *argv[]){
//Note that in c/c++, the first argument is always the command name.
if (nargs
cerr
return 1;
}
//Parsing the base, which is the third input.
unsigned int base = stoul(string(argv[3]));
if (base10){
cerr
return 1;
}
//Parsing input Integers. In parsed (integer_1 and integer_2) values the zeroth index represent the most significant digit and
//the least significant digit is at the integer.size()-1 location.
Integer integer_1 = to_integer(argv[1],base);
Integer integer_2 = to_integer(argv[2],base);
//Calling school method of summation.
Integer sum = add(integer_1,integer_2,base);
//Calling Karatsuba multiplication
Integer kmultiplication = kmul(integer_1,integer_2,base);
//Displaying the output; first sum then multiplication.
cout
return 0;
}
utils.h
#pragma once
#include "typedef.h"
#include
// Return an Integer with length dim all filled with zero digits
Integer zeros_with_digits(unsigned int dim);
// Pad specified number (n) of zeros to the least significant end.
void pad_back(Integer &in, unsigned int n);
// Pad specified number (n) of zeros to the most significant end.
void pad_front( Integer &in, unsigned int n);
// Return the string representation of the Integer. If ignore_most_sig_zeros == true,
// then any leading zeros will not be in the string.
std::string to_string(Integer a,bool ignore_most_sig_zeros = false);
// Convert a sequence of digit string to an Integer with the specified base.
Integer to_integer(std::string str,unsigned int base);
utils.cpp
#include "utils.h"
#include "typedef.h"
#include
#include
Integer zeros_with_digits(unsigned int dim)
{
Integer out(dim,0);
return out;
}
void pad_back(Integer &in, unsigned int n)
{
for (unsigned int i = 0;i
in.push_back(0);
}
}
void pad_front( Integer &in, unsigned int n)
{
for (unsigned int i = 0;i
in.insert(in.begin(),0);
}
}
std::string to_string(Integer a,bool ignore_most_sig_zeros)
{
std::stringstream ss;
bool ignore=ignore_most_sig_zeros;
for (auto it = a.begin();it!=a.end();it++){
if (ignore && *it==0)
{
continue;
}else{
ignore=false;
}
ss
}
return ss.str();
}
Integer to_integer(std::string str,unsigned int base)
{
Integer out;
for (unsigned int i = 0;i
auto digit = (unsigned int)str[i]-48;
if (digit >=base){
throw std::invalid_argument("Input contains invalid characters");
}
out.push_back(digit);
}
return out;
}
typedef.h
#pragma once
#include
#include
// Define a single digit.
typedef unsigned int Digit;
// Define an integer as a vector of Digits.
typedef std::vector Integer;
operations.h
#pragma once
#include "typedef.h"
// Add two Integers a and b given in base, and returns the output as an Integer.
Integer add( Integer a, Integer b,unsigned int base);
// Multiply two Integers a and b given in base using the Karatsuba algorithm, and
// returns the output as an Integer.
Integer kmul( Integer a, Integer b,unsigned int base);
1 Task Description You are asked to use C++ to implement: .School Method for Integer Addition Karatsuba Algorithm for Integer Multiplication You must follow this guideline!! Your submission will be marked automatically. Failure to follow this guideline will result in 1.1 Submission Guideline You are given the skeletal implementation for a program that includes: i) main method with argument parsing (main.cpp); ii) type definitions (typedef h); iii) several utility functions (utils.cpp and utils. h) and iv) a header file with method signatures for school method addition, Karatsuba multiplication (operations. ?) in the Canvas. The functionalities of the given methods are described in the respective comments You cannot change any of the provided files. You are required to provide implementations for the methods signatures provided in the operations.h file Please submit the implementations for the required functions in your own source file(s) having the file extension with .see Your program takes one line as input. The input line contains three integers separated by spaces. Let the three integers be '11 12 B'. 11 and 12 are both non-negative integers up to 100 digits long. B represents 11 and 12's base (B is from 2 to 10) The code for parsing the arguments and displaying the final result is already written in main.cpp. The output will include 2 integers: i) the sum of 11 and 12, using the school method; and ii) the product of 1 and 12, using the Karatsuba algorithm. The results will still use base B. Sample input 1: 101 5 10 Sample output 1: 106 505 Sample input 2: 10 111 2 Sample output 2: 1001 1110 1 Task Description You are asked to use C++ to implement: .School Method for Integer Addition Karatsuba Algorithm for Integer Multiplication You must follow this guideline!! Your submission will be marked automatically. Failure to follow this guideline will result in 1.1 Submission Guideline You are given the skeletal implementation for a program that includes: i) main method with argument parsing (main.cpp); ii) type definitions (typedef h); iii) several utility functions (utils.cpp and utils. h) and iv) a header file with method signatures for school method addition, Karatsuba multiplication (operations. ?) in the Canvas. The functionalities of the given methods are described in the respective comments You cannot change any of the provided files. You are required to provide implementations for the methods signatures provided in the operations.h file Please submit the implementations for the required functions in your own source file(s) having the file extension with .see Your program takes one line as input. The input line contains three integers separated by spaces. Let the three integers be '11 12 B'. 11 and 12 are both non-negative integers up to 100 digits long. B represents 11 and 12's base (B is from 2 to 10) The code for parsing the arguments and displaying the final result is already written in main.cpp. The output will include 2 integers: i) the sum of 11 and 12, using the school method; and ii) the product of 1 and 12, using the Karatsuba algorithm. The results will still use base B. Sample input 1: 101 5 10 Sample output 1: 106 505 Sample input 2: 10 111 2 Sample output 2: 1001 1110Step by Step Solution
There are 3 Steps involved in it
Step: 1
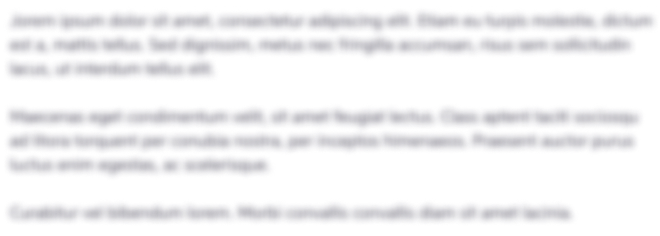
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started