Question
Could you write algorithm and comment for the fllowing C++ code. The form of algorithm and comment show in the picture. Thank you very much
Could you write algorithm and comment for the fllowing C++ code. The form of algorithm and comment show in the picture. Thank you very much
#include
#include
#include
#include
#include
using namespace std;
class Book {
private:
string title;
string author;
public:
string getTitle() {
return title;
}
void setTitle(string title) {
this->title = title;
}
string getAuthor() {
return author;
}
void setAuthor(string author) {
this->author = author;
}
Book(string title, string author) {
this->title = title;
this->author = author;
}
Book() {
title = "NONE";
author = "NONE";
}
};
class User {
private:
string name;
int ratings[100];
int numRatings;
public:
User(string name, int r[], int num) {
this->name = name;
this->numRatings = num;
for (int i = 0; i
ratings[i] = r[i];
}
}
User() {
name = "NONE";
numRatings = 0;
for (int i = 0; i
ratings[i] = 0;
}
}
int getNumRatings() {
return numRatings;
}
void setNumRatings(int numRatings) {
this->numRatings = numRatings;
}
string getName() {
return name;
}
void setName(string name) {
this->name = name;
}
int getRatingAt(int index) {
if (index >= numRatings)
return -1000;
return ratings[index];
}
int setRatingAt(int index, int rating) {
if (index >= numRatings) {
return -1000;
}
if (rating != -5 && rating != -3 && rating != 0
&& rating != 1 && rating != 3 && rating != 5) {
cout
return -1;
}
ratings[index] = rating;
cout
return 0;
}
};
class Library {
private:
Book books[100];
User users[100];
int numOfUsers;
int numOfBooks;
int loginUser;
string toLower(string s) {
for (int i = 0;i
if (s[i] >= 'A'&&s[i]
s[i] += 32;
}
}
return s;
}
int findUser(string name) {
for (int i = 0; i
if (users[i].getName() == name) {
return i;
}
}
return -1;
}
int findBook(string name) {
for (int i = 0; i
int len = books[i].getTitle().length();
if (books[i].getTitle().substr(0,len-1) == name) {
return i;
}
}
return -1;
}
int ssd(int i, int j) {
int sum = 0;
for (int k = 0;k
sum += ((users[i].getRatingAt(k) - users[j].getRatingAt(k)) * (users[i].getRatingAt(k) - users[j].getRatingAt(k)));
}
return sum;
}
int mostSimilar() {
int minssd;
int min;
if (loginUser == 0) {
minssd = ssd(loginUser, 1);
min = 1;
}
else {
minssd = ssd(loginUser, 0);
min = 0;
}
for (int i = 0;i
if (i == loginUser)
continue;
if (ssd(loginUser, i)
minssd = ssd(loginUser, i);
min = i;
}
}
return min;
}
public:
Library() :numOfUsers(0), numOfBooks(0) { }
void loadData() {
ifstream bookfile("books.txt");
ifstream userfile("ratings.txt");
if (!bookfile.is_open() || !userfile.is_open()) {
cout
cout
exit(1);
}
string line;
while (!bookfile.eof()) {
if (bookfile.fail()) {
break;
}
getline(bookfile, line);
if (line.empty()) {
continue;
}
line = toLower(line);
int comma = line.find(',');
books[numOfBooks].setAuthor(line.substr(0, comma));
books[numOfBooks].setTitle(line.substr(comma + 1));
numOfBooks++;
}
bookfile.close();
while (!userfile.eof()) {
if (userfile.fail()) {
break;
}
getline(userfile, line);
if (line.empty()) {
continue;
}
line = toLower(line);
int comma = line.find(',');
stringstream ss;
int ratings[100];
ss.str(line.substr(comma + 1));
for (int i = 0; i
int rating;
ss >> rating;
ratings[i] = rating;
}
users[numOfUsers++] = User(line.substr(0, comma), ratings, numOfBooks);
}
userfile.close();
cout
}
void login() {
cout
string name;
getline(cin, name);
while (name.empty()) {
cout
cout
getline(cin, name);
}
string lowername = toLower(name);
int res = findUser(lowername);
if (res != -1) {
cout
loginUser = res;
}
else {
cout
User newUser;
newUser.setName(toLower(name));
newUser.setNumRatings(numOfBooks);
loginUser = numOfUsers;
users[numOfUsers] = newUser;
numOfUsers++;
}
}
char menu() {
string input;
while (true) {
cout
getline(cin, input);
if (input.length() == 1
&& (input[0] == 'v' || input[0] == 'V'
|| input[0] == 'r' || input[0] == 'R'
|| input[0] == 'g' || input[0] == 'G'
|| input[0] == 'q' || input[0] == 'Q')) {
return input[0];
}
else {
cout
}
}
}
void viewRatings() {
bool hasRated = false;
for (int i = 0; i
if (users[loginUser].getRatingAt(i) != 0) {
hasRated = true;
break;
}
}
if (!hasRated) {
cout
return;
}
cout
for (int i = 0;i
if (users[loginUser].getRatingAt(i) != 0) {
string s1 = books[i].getTitle();
int ans = users[loginUser].getRatingAt(i);
int len = s1.length();
s1 = s1.substr(0,len-1);
cout
cout
cout
}
}
}
void rateBook() {
while (true) {
cout
string title;
string str;
getline(cin, title);
str = title;
string lowerTitle = toLower(title);
cout
int rating;
cin >> rating;
getchar();
int index = findBook(lowerTitle);
if (index == -1) {
cout
}
else {
users[loginUser].setRatingAt(index, rating);
cout
return;
}
}
}
void recommend() {
int similar = mostSimilar();
bool hasRecommend = false;
for (int i = 0; i
if (users[similar].getRatingAt(i) >= 3 && users[loginUser].getRatingAt(i) == 0) {
hasRecommend = true;
break;
}
}
if (!hasRecommend) {
cout
return;
}
cout
int recommendBooks = 0;
for (int i = 0; i
if (users[similar].getRatingAt(i) >= 3 && users[loginUser].getRatingAt(i) == 0) {
int l1 = books[i].getTitle().length();
int l2 = books[i].getAuthor().length();
cout
recommendBooks++;
}
}
}
void quit() {
ofstream out("ratings_new.txt");
for (int i = 0;i
out
for (int j = 0;j
out
}
out
}
out.close();
cout
}
};
int main()
{
Library library;
library.loadData();
library.login();
char opt;
while (true) {
opt = library.menu();
switch (opt) {
case 'v':
case 'V':
library.viewRatings();
break;
case 'r':
case 'R':
library.rateBook();
break;
case 'g':
case 'G':
library.recommend();
break;
case 'q':
case 'Q':
library.quit();
return 0;
}
}
7 using namespace std; ALgorithe: that checks what range a given NPG folls into . 1. Take the mpg value passed to the function. 11 12 13 14 15 16 Comments (10 points) 2 Check f tt is greater than se If yes, then print "Nice Job . If not, then check f t ts greater than 25 Alorithm If yes, then print "Not great, but oay 4. If not, then print "So bad, so very, very bad (10 points) Input parometers: iles per gollon (Float type 18Output:dfferent string bosed on three cotegortes of G:50, 25-49, and Less than 25 Returns: nothing 21 23 void checkPG(Float mpg) 50) check f the Input volue ts greater than 5e 25 26 27 28 29 30 31 32 cout ee "Nice job e endl; 7 output message lse if(ng25) 7/ff not, check f is greater than 25 coutStep by Step Solution
There are 3 Steps involved in it
Step: 1
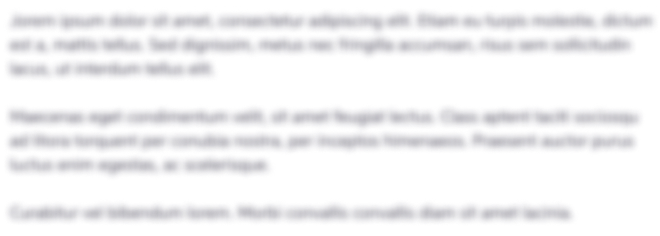
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started