Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Course Project Guidance ======================= File input/output ----------------- (You should complete this part during week 3.) Make sure you use relative (not absolute) paths for the
Course Project Guidance ======================= File input/output ----------------- (You should complete this part during week 3.) Make sure you use relative (not absolute) paths for the input and output text file locations. User interface -------------- (You should complete this part during week 5.) A big help for this is to study the week 5 video "Java GUI List Components". In this video, note the 2 list boxes and their corresponding list models. Use a border layout for your contents pane. In the north region include the following title: Reverse a Text File via a Stack. Use a panel in the center region to contain the 2 list boxes (side-by-side). Place the 3 buttons in the south region. Stack usage ----------- (You should complete this part during week 7.) Use the Java class library Stack class for your stack. Suggested design/outline ------------------------ Below is a suggested design/outline for your project code. It defines a single class called ReverseFileViaStack. Inside this class, the outline suggests various fields and functions. Add any other fields that are needed. Code the bodies of the suggested functions. Test your program carefully to make sure it looks and performs as specified. Suggested import statements: import java.awt.*; import java.awt.event.*; import java.io.*; import javax.swing.*; import javax.swing.border.*; import java.util.*; Suggested class statement: public class ReverseFileViaStack extends JFrame implements ActionListener { // Everything in your class (see below). } Some suggested fields: // Fonts: private final Font fontBold = new Font(Font.DIALOG, Font.BOLD, 14); private final Font fontPlain = new Font(Font.DIALOG, Font.PLAIN, 14); // Lists: private JListlist1; private JList list2; // List models: private DefaultListModel listModel1 = new DefaultListModel<>(); private DefaultListModel listModel2 = new DefaultListModel<>(); // Stack (from the Java Collections Framework): private Stack stack = new Stack<>(); Some suggested functions: // Constructor. public ReverseFileViaStack() { // It should setup the entire UI. // Here are a few tricky parts: listModel1 = new DefaultListModel(); list1 = new JList<>(listModel1); listModel2 = new DefaultListModel(); list2 = new JList<>(listModel2); } // Button handler. // Calls the appropriate function based on which button was clicked. public void actionPerformed(ActionEvent e) { Object source = e.getSource(); if (source == btnRead) { readFile(); return; } if (source == btnReverse) { reverseFile(); return; } if (source == btnWrite) { writeFile(); return; } } // Reads the input text file line-by-line. // Adds each line it gets to the left list box. // To add each item (line) to the left list box, use its list model // addElement function. private void readFile() { } // Pushes each item from the left list onto the stack. // This uses the stack's push function. // To read each item in the left list box, use its list model // elementAt function (with a loop index). // Then pops the items from the stack one-by-one and adds each of them // to the right list. // This uses the stack's pop function. // To add each item (line) to the right list box, use its list model // addElement function. // This results in the right list being in reverse order of the left. private void reverseFile() { } // See the video. private void setFonts() { UIManager.put("Button.font", fontBold); UIManager.put("ComboBox.font", fontBold); UIManager.put("Label.font", fontBold); UIManager.put("List.font", fontPlain); } // See the video. private void setSpecificSize(JComponent component, Dimension dimension) { component.setMinimumSize(dimension); component.setPreferredSize(dimension); component.setMaximumSize(dimension); } // Reads through the right list item-by-item. // Uses a PrintWriter to write these items to the output file. // Be sure to close the PrintWriter. private void writeFile() { } // The main function. public static void main(String[] args) { ReverseFileViaStack gui = new ReverseFileViaStack(); gui.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
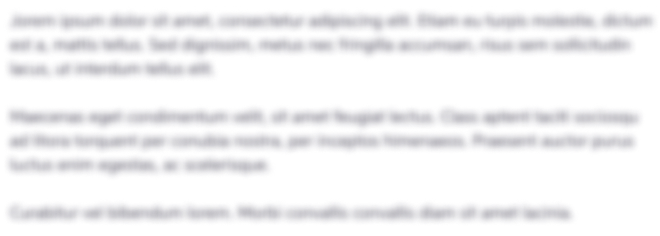
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started