Question
Create a C++ program A problem in timber management is to determine how much of an area to leave uncut so that the harvested area
Create a C++ program
A problem in timber management is to determine how much of an area to leave uncut so that the harvested area is reforested in a certain period of time. Given that reforestation takes place at a known rate per year (depending on climate and soil conditions) one can make a few helpful estimates.
Your job including writing two timber regrowth functions plus a welcome/menu function and an output function. These will go with a main() function (and other functions if/as needed) that will test them
Reforestation Model:
A reforestation equation expresses annual regrowth as a function of the amount of timber standing and the reforestation rate:
NewAcres = PreviousAcres + ReforestationRate * PreviousAcres
For example, if 100 acres are standing after harvesting and the reforestation rate is 0.05, then 100 + 0.05 * 100 = 105 acres are forested at the end of the first year; at the end of the second year it is 105 + 0.05 * 105 or 110.25 acres. If you repeat this for n years, you can find the total reforested area after a given period of time. For this program we will be using type double for acreage and reforestation rate variables and type unsigned int for years.
Required Functions:
WelcomeAndMenuWelcome the user. Show them the menu of options:
-
1) Reforestation Table
-
2) Determine Years to Reforest
3) Quit Accept their choice. You can decide if you want them to enter a char or an int for their choice. Return this choice to main().
ReforestTablethis function accepts the area left uncut (which is the initial value for "PreviousAcres"), the reforestation rate, and the number of years (an unsigned int) as parameters. (These values are input in main()). It outputs a table showing the number of acres forested at the end of each year, from year 0 (the starting point) through the given number of years. For example:
IMPORTANTto receive credit for this assignment, your program must not only find the correct answer but must include at least these functions, along with main(). Programs without these functions will not be graded.
Year Forested Acres
0 100.00
1 105.00
2 110.25
and so on. Note that year 0 is the initial uncut area AND the starting point for the table. YOU MUST USE A for-LOOP TO CREATE THIS TABLE. You may choose the digits-of-precision to be 1,
2 or 3 digits, but this must be consistent. Lining the table up counts to. The function returns no
value to the caller.
-
YearsToReforest this function accepts the area left uncut, the reforestation rate, and a
target area to be reforested. (For example, 2500 acres left standing, a rate of 0.02 and a target of 14,000 acres eventually forested.) It then determines and returns to the caller the number of years (an unsigned int) it will take for the given area to be completely reforested. YOU MUST USE A while LOOP FOR THIS CALCULATION. Looping stops when the number of acres reforested either equals or exceeds the target. (Note: In its final version, this function has no cout statements.)
-
OutputYears this function is used AFTER YearsToReforest has done its job. It accepts the number of years it will take for the area to be completely reforested as an unsigned int parameter and outputs this value with an appropriate message.
Testing the Functions:
Here is the basic outline of the main() function (which mostly calls functions and accepts a little input)
Call WelcomeAndMenu() While they have not chosen to quit: Use a switch statement to determine what happens next:
o If the user selects 1), request the area left uncut, reforestation rate and number of years, then call ReforestTable(). The values just input will be the arguments sent to the parameters of ReforestTable. To make data-entry code simpler, we will NOT do any data validation (even though we should). That is, we will assume users will (correctly) enter numbers that are >0 for each of these. (Using an unsigned int for the number of years handles this for length of time.) 1
o If the user selects 2), request the area left uncut, the reforestation rate and the target area to be reforested. The only input validation here is that the target area must be > the area originally left uncut. If this is NOT the case, allow the user to keep entering values until they get it right. USE A do-while LOOP. (Once you do this, the extra credit in the previous bullet might should be fairly easy to get .) Then call YearsToReforest(). After this function returns the years, call OutputYears().
o When they select 3), thank them and quit.
o If they type in any other value for their choice, output an error message (but DON'T quit). Unless they chose to quit call WelcomeAndMenu()
ALL data must be passed by using arguments and parameters or through return statements. GLOBAL VARIABLES WILL LEAD TO SEVERE POINT LOSS.
1 If youd like a little extra credit, use data validation do-while loop(s) to ensure that the area left uncut and reforestation rate are positive.
-
Note: while you do NOT need to include analysis/design with this program because Ive given you the outline, it wouldnt hurt to make a few notes before you begin (hint, hint).
-
Style in the selection of identifier names, layout and so on count.
Hand-in:
-
Your main.cpp
-
You may test your program with multiple forestation scenarios, but I need to see the following run
(and, yes, this should require only 1 run of the program):
Menu Selection 1 100 uncut 0.05 rate 20 years
2 100 uncut 0.05 rate 250 Target Area
2 2500 uncut 0.02 rate 1400 target area (retry)
1400 target area
4 (Error: should not as for any more data)
3 (Thank the user and quit)
Submit a screen shot showing this run. (Those of you using the little tiny XCode output screen may want to / need to enlarge this or rearrange the interface a bit or you won't be able to see the whole of a single run's output.)
THIS IS MY CODE SO FAR*******
#include
int WelcomeAndMenu() { int choice; cout << " TIMBER REGROWTH "; cout << "\t1. Reforestation Table "; cout << "\t2. Determine Years to Reforest "; cout << "\t3. Quit "; cout << " \tEnter the number of your choice: "; cin >> choice; return choice; } int main() { double reforestRate = 0.02; int acresUncut = 2500; int acresForested, menuChoice, newAcres, previousAcres, targetArea, acresReforested, YearsToReforest; int numYears= 1; int maxYears = 0;
cout << "Enter the amount of acres uncut: " << endl; cin >> acresUncut;
cout << "Enter the number of years: "; cin >> numYears;
cout << "Enter the reforestation rate" cin >> reforestRate;
cout << fixed << showpoint << setprecision(1); menuChoice = WelcomeAndMenu(); if (menuChoice == 3) { cout << "You have chosen to quit." << endl; cout << "Thank you for using the timber growth code!" << endl; } while (menuChoice != 4) { switch (menuChoice) { case 1: do { acresForested = reforestRate * acresForested; cout << "Enter the number of years: "; cin >> numYears; if (maxYears >= 1); cout << "Error: Has to be less than the maximum number of years." << endl; } while (numYears <= maxYears); { newAcres = previousAcres + reforestRate * previousAcres; } case 2: do{ cout << "Enter the target area to be restored: " << endl; cin >> targetArea; } while (acresReforested >= targetArea); { } case 3: do { cout << "Calculate the number of acres forested:" << endl; YearsToReforest = }
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
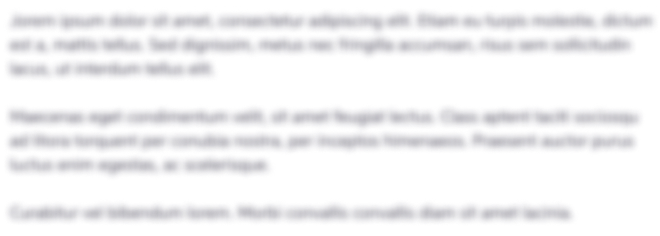
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started