Question
Create a C++ program that dynamically creates and resizes string arrays. Please read this document in its entirety before you start coding. 1. Design a
Create a C++ program that dynamically creates and resizes string arrays. Please read this document in its entirety before you start coding. 1. Design a class that has a pointer to the string array and the array size as instance data fields. 2. Your class must have a default constructor (no argument) which creates an array of a default size and another constructor that creates an array of a size equal to this constructors single parameter of type int. Both constructors must create arrays dynamically and populate these arrays with arbitrary generated strings. Ask the user for the desired size of the string array. 3. Create a member function with the following prototype: string* add(int& size, string toAdd); where the parameter: - size is the size of the new array dynamically created inside the function - toAdd is the string to be added to the new array The function should: a. create dynamically a new string array with the size one element larger than the existing one; b. copy all elements from the existing array into the new array; c. add the toAdd string parameter to the new array; d. assign the new array size to the size parameter; e. release the memory used by the existing array; f. assign the new array pointer to the existing array pointer and appropriately adjust the private array size field; g. return the pointer to the new array. 4. Create another member function with the following prototype: string* del(int& size, string toDel); where the parameter: - size is the size of the new array dynamically created (if necessary) inside the function - toDel is the string to be removed (if found) from the existing array If the toDel string is found in the existing array, the function should: a. create dynamically a new string array with the size one element smaller than the existing one; b. copy all elements with the exception of the one equal to toDel from the existing array into the new array; c. assign the new array size to the size parameter; d. release the memory used by the existing array; e. assign the new array pointer to the existing array pointer and appropriately adjust the private array size field; f. return the pointer to the new array. If the toDel string is not found in the existing array, the function should return the NULL pointer indicating that the toDel string was not found. Notes: a. If the existing array has more than one occurrence of the toDel string it is enough to delete the first occurrence only. b. The term existing array refers to the array that is a private instance data member of the class created. 5. Overload the insertion operator << to allow the human readable output of all elements of the string array with a single cout << statement. 6. Test created two functions in your main() function. The initial size of the string array as well as toAdd and toDel parameter strings should be provided by the user. 7. To prove that your code works, output all created arrays using the overloaded operator << in an easy to read format. 8. Make sure that you release all allocated memory as soon as possible (inside the functions add and del as well as in the class destructor) and definitely before exiting main() so that your program does not create any memory leaks. 9. Implement error processing to recover from invalid user data, and invalid system calls. 10. Every method must have a header showing name of the method, describing its purpose, and any input/output along with its Pre-& Post-condition. This information must be included in the methods implementation and not with its prototype.
11.Create a menu as follows:
Welcome to string array: To Add or NOT to Add Please specify the Size of the Array: 5 Menu ------- 1 - Add a string to an Array 2 - Delete a String from an Array 3 - Display Array Contents 4 - Add an entire Array of Strings to an Array 5 - Delete an entire Array of Strings from an Array 6 - Exit Program
Tie the menu items 1, 2, 3, and 6 to your existing code, and adding prompt for user input as appropriate
Implement menu items 4 and 5 with the two new member functions: (void addArray(string arrayToAdd[], int size, int& capacity);) (void removeArray(string arrayToRemove[], int size, int& capacity);)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
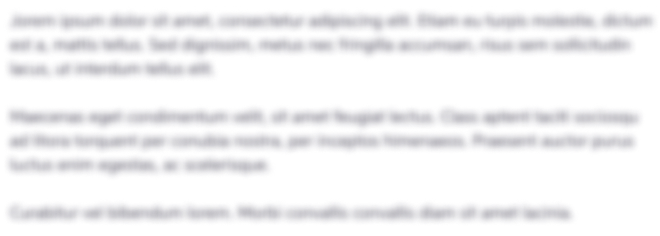
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started