Question
Create a C++ recursive function for the Fibonacci Sequence. Here is the program written in a non-recursive way that will display the first 10 digits
Create a C++ recursive function for the Fibonacci Sequence.
Here is the program written in a non-recursive way that will display the first 10 digits of the Fibonacci Sequence.
It must do the same, but needs to be written recursively.
Problem Two:
Here codes for non-recursive Fibonacci sequence problem, use it to rewrite a recursive Fibonacci solution:
//Program: Fibonacci number
#include
using namespace std;
void FibonacciNum( int position);
int main()
{
cout << "The Fibonacci numbers upto position "
<< 10 << " are " ;
FibonacciNum(11);
cout<< endl;
return 0;
}
void FibonacciNum( int nthFibNum)
{
int current;
int counter;
int first,second;
if (nthFibNum == 1)
{ first =1;
current = first;
cout<< current<<" ";
}
else if (nthFibNum == 2)
{
second = 2;
current = second;
cout<< current<<" ";
}
else
{
counter = 3;
while (counter <= nthFibNum)
{
current = second + first;
cout<< current<<" ";
first = second;
second = current;
counter++;
}//end while
}//end else
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
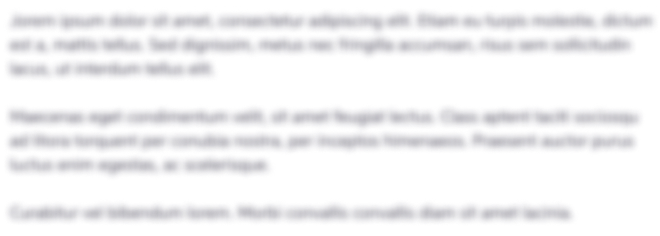
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started